Non Primitive Data types are those data types in java that have the same size and provide additional methods to perform certain operations; in short, non-primitive data types in java refer to objects and are also named reference types; examples of non-primitive data types available in java include array, string, classes, and interfaces.
Start Your Free Software Development Course
Web development, programming languages, Software testing & others
Syntax
Below is the syntax showing how non-primitive data types are used in java:
Array: An Array in java is used as the following:
int[] intArray; // declaring array of type int byte[] byteArray // declaring array of type byte long[] longArray; // declaring array of type long short[] shortArray // declaring array of type short float[] floatArray; // declaring array of type float long[] longArray // declaring array of type long char[] charArray // declaring array of type char ClassName[] classArray // declaring array a particular class Object[] objectArray // declaring array of objects
From the above, we can see that we can create an array of different data types or even objects and classes. Note that in an array, all elements should be of the same data type.
String: A Java String can be used like the following:
String str = "Edubca"; // declaring a string in java
Classes: Here is how a java class is created:
package <package name>; // declaring package of class public class <classname>{ //contains data and methods }</classname></package>
Interfaces: Here is how an interface is created in java:
package <package name>; // declaring package of interface public interface <interfacename>{ //contains unimplemented methods and data if needed }</interfacename></package>
Non Primitive Types in Java
The following are non-primitive data types available in java:
- Array: An Array can be defined as a homogenous collection of elements having a fixed size. Arrays can store one or more values belonging to the same data type, and individual elements of an array can be accessed through an index. This means an array follows the index-based approach for accessing elements.
- String: A string can be defined as a sequence of characters. A String is java is represented as an object of java.lang.String class. To create an instance of string in java, we need to create an object of java.lang.String class is an immutable and thread-safe class.
- Class: A java class can be defined as a blueprint of data defined by the user and used to create objects. A class in java defines a set of properties or methods used to define an object’s state or behavior.
- Interface: Interface in java is used to provide an abstraction over data. Similar to a class, interfaces contain data and methods, but methods declared inside an interface are by default abstract. Abstract methods are those methods that do not contain anybody; they only contain the method signature.
Examples of Non-Primitive Data Types in Java
Different examples are mentioned below
Example #1
In this example, we will show how to use array and string in java:
import java.util.*; public class DataTypeDemo { public static void main(String[] args) { byte[] byteArray= {88,77,66,55}; //declaring byte Array int[] intArray= {20,15,10,4}; // declaring integer Array short[] shortArray= {6000,5000,4000,3000}; //declaring short Array long[] longArray = {20000000000000L,30000000000000L,40000000000000L,50000000000000L}; //declaring long Array float[] floatArray= {1.1f,1.2f,1.3f,1.4f}; // declaring float Array double[] doubleArray = {29.94d,19.98d,20,87d}; // declaring double Array boolean[] booleanArray= {true,false,true, true}; //declaring boolean Array char[] charArray = {'A','B','C','D'}; // declaring character Array System.out.println("Array Declared using Byte Data Type is " + Arrays.toString(byteArray)); System.out.println("Array Declared using Integer Data Type is " + Arrays.toString(intArray)); System.out.println("Array Declared using Short Data Type is " + Arrays.toString(shortArray)); System.out.println("Array Declared using Long Data Type is " + Arrays.toString(longArray)); System.out.println("Array Declared using Float Data Type is " + Arrays.toString(floatArray)); System.out.println("Array Declared using Double Data Type is " + Arrays.toString(doubleArray)); System.out.println("Array Declared using Boolean Data Type is " + Arrays.toString(booleanArray)); System.out.println("Array Declared using Character Data Type is " + Arrays.toString(charArray)); } }
Output:
Example #2
In this example, we will see how classes and interfaces are used in java:
Here is how an interface is declared in java:
// declaring an interface interface namePrinter{ // declaring abstract method (method without body) String getName(); } //creating java class implementing interface namePrinter public class Main implements namePrinter{ public static void main(String[] args) { Main main =new Main(); String name=main.getName(); System.out.println("Name returned from getName method is >> " + name ); } // overriding method of an interface @Override public String getName() { String name ="Edubca"; // TODO Auto-generated method stub return name; } }
Output:
Conclusion
Properly understanding different data types is very important to learning any programming language. The above article explains the types in detail with examples and the significance of each data type.
The above is the detailed content of Non-Primitive Data Types in java. For more information, please follow other related articles on the PHP Chinese website!
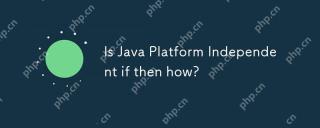
Java is platform-independent because of its "write once, run everywhere" design philosophy, which relies on Java virtual machines (JVMs) and bytecode. 1) Java code is compiled into bytecode, interpreted by the JVM or compiled on the fly locally. 2) Pay attention to library dependencies, performance differences and environment configuration. 3) Using standard libraries, cross-platform testing and version management is the best practice to ensure platform independence.
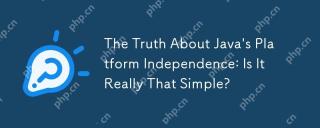
Java'splatformindependenceisnotsimple;itinvolvescomplexities.1)JVMcompatibilitymustbeensuredacrossplatforms.2)Nativelibrariesandsystemcallsneedcarefulhandling.3)Dependenciesandlibrariesrequirecross-platformcompatibility.4)Performanceoptimizationacros
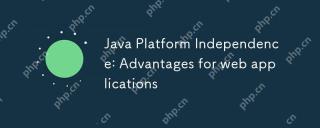
Java'splatformindependencebenefitswebapplicationsbyallowingcodetorunonanysystemwithaJVM,simplifyingdeploymentandscaling.Itenables:1)easydeploymentacrossdifferentservers,2)seamlessscalingacrosscloudplatforms,and3)consistentdevelopmenttodeploymentproce
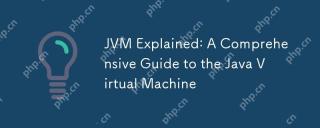
TheJVMistheruntimeenvironmentforexecutingJavabytecode,crucialforJava's"writeonce,runanywhere"capability.Itmanagesmemory,executesthreads,andensuressecurity,makingitessentialforJavadeveloperstounderstandforefficientandrobustapplicationdevelop
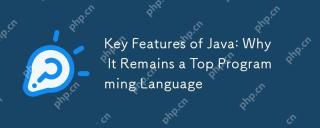
Javaremainsatopchoicefordevelopersduetoitsplatformindependence,object-orienteddesign,strongtyping,automaticmemorymanagement,andcomprehensivestandardlibrary.ThesefeaturesmakeJavaversatileandpowerful,suitableforawiderangeofapplications,despitesomechall
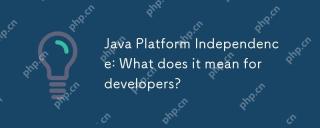
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
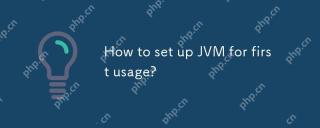
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
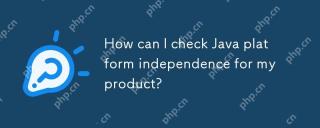
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
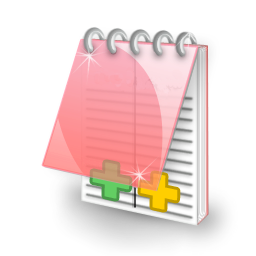
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
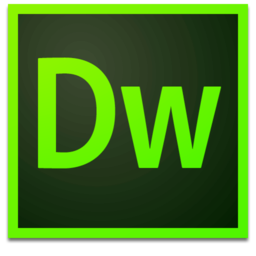
Dreamweaver Mac version
Visual web development tools
