In this tutorial, we will be learning how to implement star patterns in PHP. Printing different patterns in PHP is simple and easy to learn. And it would be good to have knowledge in other programming languages like C or C++. We can print pyramid triangle patterns, star patterns, number patterns, etc. We will be learning them in this tutorial, out of many different patterns. We use for loop to print these patterns. We can use the for each loop also and nested for loop to print these patterns. In these nested loops, we will be using an outer loop and inner loop to print the stars for one particular pattern.
ADVERTISEMENT Popular Course in this category PHP DEVELOPER - Specialization | 8 Course Series | 3 Mock TestsStart Your Free Software Development Course
Web development, programming languages, Software testing & others
Examples of Star Patterns in PHP
Below are the 6 examples of Star Patterns in PHP:
- This is a simple star pattern in PHP. We will be using two loops, outer and inner loop. The outer loop is for rows and the inner loop is for columns. The outer for loop with a value of I iterate 5 times starting from 0 and ending with value 5 as we want five rows. The outer for loop represents rows of the pattern.
- Next, the inner for loop also iterate 5 times starting from 0 and ends with a value less than the value of 5. The inner for loop represents columns of the pattern.
- As per the syntax of for loop, the outer loop begins with 0, checks the condition whether it is less than 5 if yes, it will go inside the loop. Inside the for loop is, again a for loop for columns, where the variable j is initialized to 0 and this loop will print stars(*) in the first row, and will loop till the condition $j
- After $j value is greater than 5 the control will come out of the loop and the cursor will come in the next line. Now the value of i is incremented by 1, which means the value is 2 and again the j for loop will loop 5 times and print the 5 stars.
- This will repeat 5 times until the value of i is greater than 5. Once the value is greater than 5 the program will stop executing and the desired output is printed.
Example #1
Code:
<?php //example to demonstrate star pattern-1 for($i=0; $i<5; $i++) { for($j=0; $j<5; $j++) { echo '*'; } echo '<br>'; } ?>
Output:
Example #2
In this example, the i for loop iterates 5 times and for each value of i, the inner j for loop will iterate and print as star *. The j for loop is used to print the stars. For the initial value of i as 1, one star will be printed. Next for the value of 2, two stars in one row will be printed, again for the value of 3, three stars will be printed and this will continue till the value is not greater than 5.
Code:
<?php //example to demonstrate star pattern-2 for($i=1; $i<=5; $i++) { for($j=1; $j<=$i; $j++) { echo '*'; } echo '<br>'; } ?>
Output:
Example #3
In this example, the i for loop will loop 5 times as we want 5 rows. The j for loop is used to print * depending upon the value of i. For the first time as we want 5 stars in the first row, so for the first value of i as 1, the j loop will print 5 stars. Next for the value of 2, the j loop will print 4 stars, for the next value 3, the j loop will print 3 times and so on. This will stop once the condition of i is greater than 5 and will print the desired output.
Code:
<?php //example to demonstrate star pattern-3 for($i=1; $i<=5; $i++) { for($j=5; $j>=$i; $j--) { echo '*'; } echo '<br>'; } ?>
Output:
Example #4
In this example, the value I for loop is iterated 5 times, as the number of rows in the star pattern is 5. Also in this example, we are using j for loop to print spaces and a new k for loop is used to print stars *.
Code:
<?php //example to demonstrate star pattern-4 for($i=1; $i<=5; $i++) { for($j=4; $j>=$i; $j--) //loop to print spaces { echo ' '; } for($k=1; $k'; } ?>
Output:
Example #5
In this example, three loops are used, one for the number of rows, second for the printing of spaces and third to print the stars. Both the loops defined are dependent on the value of i.
Code:
<?php //example to demonstrate star pattern-5 for($i=1; $i<=6; $i++) { for($j=1; $j<=$i; $j++) //loop to print spaces { echo ' '; } for($k=5; $k>=$i; $k--) //loop to print stars { echo '*'; } echo '<br>'; } ?>
Output:
Example #6
In this example, there is a combination of two stars patterns upper triangle and lower triangle. These triangles are already explained in the previous examples and for this, we use three loops, one for the number of rows, second for the printing of spaces and third for the printing of stars and this loop is repeated again with different initial values of i and j along with different conditions for the next half of the triangle pattern.
Code:
<?php //example to demonstrate star pattern-5 // this loop prints the upper half of the star pattern for($i=1; $i<=5; $i++) { for($j=1; $j<=$i; $j++) //loop to print spaces { echo '*'; } echo '<br>'; } // this loop prints the lower half of the pattern for($i=1; $i=$i; $j--) //loop to print stars { echo '*'; } echo '<br>'; } ?>
Output:
Conclusion
In this article, star patterns in PHP are explained. We have seen different forms of star patterns. These patterns are with an explanation of how the condition works, how the looping works to print the stars as desired.
The above is the detailed content of Star Patterns in PHP. For more information, please follow other related articles on the PHP Chinese website!
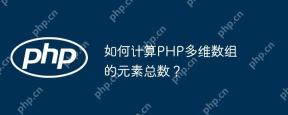
Calculating the total number of elements in a PHP multidimensional array can be done using recursive or iterative methods. 1. The recursive method counts by traversing the array and recursively processing nested arrays. 2. The iterative method uses the stack to simulate recursion to avoid depth problems. 3. The array_walk_recursive function can also be implemented, but it requires manual counting.
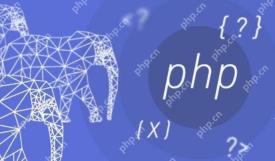
In PHP, the characteristic of a do-while loop is to ensure that the loop body is executed at least once, and then decide whether to continue the loop based on the conditions. 1) It executes the loop body before conditional checking, suitable for scenarios where operations need to be performed at least once, such as user input verification and menu systems. 2) However, the syntax of the do-while loop can cause confusion among newbies and may add unnecessary performance overhead.
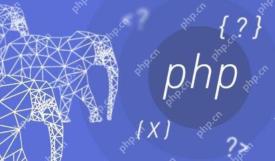
Efficient hashing strings in PHP can use the following methods: 1. Use the md5 function for fast hashing, but is not suitable for password storage. 2. Use the sha256 function to improve security. 3. Use the password_hash function to process passwords to provide the highest security and convenience.
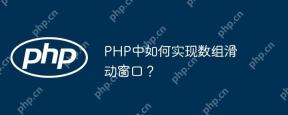
Implementing an array sliding window in PHP can be done by functions slideWindow and slideWindowAverage. 1. Use the slideWindow function to split an array into a fixed-size subarray. 2. Use the slideWindowAverage function to calculate the average value in each window. 3. For real-time data streams, asynchronous processing and outlier detection can be used using ReactPHP.
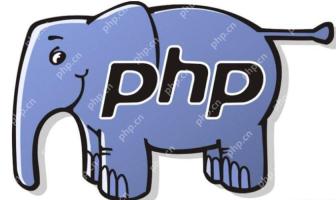
The __clone method in PHP is used to perform custom operations when object cloning. When cloning an object using the clone keyword, if the object has a __clone method, the method will be automatically called, allowing customized processing during the cloning process, such as resetting the reference type attribute to ensure the independence of the cloned object.
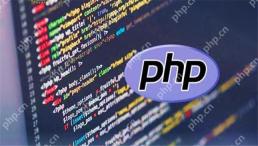
In PHP, goto statements are used to unconditionally jump to specific tags in the program. 1) It can simplify the processing of complex nested loops or conditional statements, but 2) Using goto may make the code difficult to understand and maintain, and 3) It is recommended to give priority to the use of structured control statements. Overall, goto should be used with caution and best practices are followed to ensure the readability and maintainability of the code.
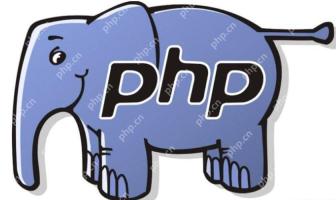
In PHP, data statistics can be achieved by using built-in functions, custom functions, and third-party libraries. 1) Use built-in functions such as array_sum() and count() to perform basic statistics. 2) Write custom functions to calculate complex statistics such as medians. 3) Use the PHP-ML library to perform advanced statistical analysis. Through these methods, data statistics can be performed efficiently.
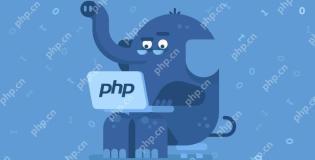
Yes, anonymous functions in PHP refer to functions without names. They can be passed as parameters to other functions and as return values of functions, making the code more flexible and efficient. When using anonymous functions, you need to pay attention to scope and performance issues.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
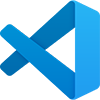
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
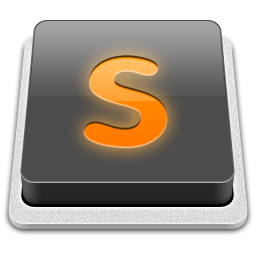
SublimeText3 Mac version
God-level code editing software (SublimeText3)
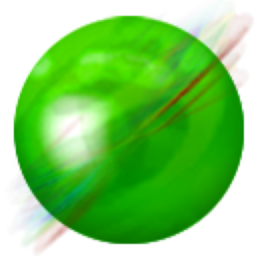
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
