One of the things that I find really cool about DIO bootcamps is that during the trail there are some code exercises to be carried out, with an editor nearby and some conditions; a bit of a HackerRank vibe like that. It's really cool because it helps to consolidate the knowledge acquired during the theoretical parts and it's not a more complex approach like the project challenge: it's something more simplified, to test your logical reasoning and your knowledge of the language. Just like HackerRank, they give you some ready-made snippets and you develop your logic based on that.
This week was crazy, so the only thing I managed to do was solve the two challenges proposed in the module "Exploring Telephony Services". As the sponsor of this bootcamp is Claro, many of the themes will have a telecom flavor.
Contracted Service Verification
Statement:
A telecommunications concessionaire offers four types of services: mobile telephony, fixed telephony, broadband and pay TV. To facilitate customer service, it is necessary to implement a program that checks whether a specific customer has contracted a certain service. For example, when a customer calls the call center and mentions a service, the attendant must be able to quickly check whether that service is contracted by the customer.
Prohibited:
Two strings: One with the service that the application will check (for example, mobile, fixed, broadband, TV). The second must contain the customer's name and which products they have, separated by a comma (Alice, mobile, fixed)
Expected output:
If the customer contracted the service described in the first entry, the application must display Yes. Otherwise, it must display No.
Examples:
Entrada | Saída |
---|---|
movel Alice,movel,fixa |
Sim |
fixa Bob,movel,tv |
Nao |
tv Carol,movel,fixa,tv |
Sim |
Initial code:
import java.util.Scanner; public class VerificacaoServico { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); // Entrada do serviço a ser verificado String servico = scanner.nextLine().trim(); // Entrada do nome do cliente e os serviços contratados String entradaCliente = scanner.nextLine().trim(); // Separando o nome do cliente e os serviços contratados String[] partes = entradaCliente.split(","); String nomeCliente = partes[0]; boolean contratado = false; // TODO: Verifique se o serviço está na lista de serviços contratados scanner.close(); } }
Resolution:
This is a relatively simple challenge. The application receives a string delimited by commas that is transformed into an array and we need to find out if there is a string within it that matches another user input, which is the service we want to check if the client has. Easy, right?
For me, who has a history of JavaScript and C#, just use a checker method (like Array.includes() or List.Contains()). Right? Wrong.
In Java, there is no method like this in the Array class. This may be due to the implementation being much closer to what happens in low-level languages (such as C), which establishes that they must be simple and efficient data structures. Apparently this type of query is not part of the essential functions of this structure.
Discovering this information was a shock. What does Java expect me to do? That I write a loop for and check manually if each element matches the item I'm looking for? Brother, I work full time, I have a daughter under two years old and I'm still studying Java. I don't have time for that, man.
But I discovered that since Java 8 it is possible to convert the array into a list and this one has the .contains() method. So, to solve this problem, just convert the parts array into a list, and then check if the string passed in the service exists within this list.
If it exists, we print Yes and otherwise, we print No.
import java.util.Arrays; import java.util.Scanner; public class VerificacaoServico { public static void main(String[] args) { //... // TODO: Verifique se o serviço está na lista de serviços contratados contratado = Arrays.asList(partes).contains(servico); System.out.println(contratado ? "Sim" : "Nao"); scanner.close(); } }
With this the exercise is completed, but during the research I did I discovered that since Java 8 there is an abstraction that helps to work with collections of data in a simpler way and with a more functional approach, similar to what exists in JavaScript: streams.
Just like with lists, we can convert the vector into a stream and check if any of the elements present in it correspond to what was passed in the service:
import java.util.Arrays; import java.util.Scanner; public class VerificacaoServico { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); // Entrada do serviço a ser verificado String servico = scanner.nextLine().trim(); // Entrada do nome do cliente e os serviços contratados String entradaCliente = scanner.nextLine().trim(); // Separando o nome do cliente e os serviços contratados String[] partes = entradaCliente.split(","); String nomeCliente = partes[0]; boolean contratado = false; contratado = Arrays.stream(partes).anyMatch(servico::equals); System.out.println(contratado ? "Sim" : "Nao"); scanner.close(); } }
We could have checked if p and servico not only have the same value but also point to the same address of memory (that is, if they are in fact the same object). Normally, when dealing with strings, this comparison will return false even if the value is equal -- that is, a false negative. Therefore, comparison using servico::equals is more appropriate, because it only compares the values between the two elements, more or less like the JavaScript equality comparator (==).
With this change, we can judge that the exercise is complete. What remains is to run the tests and see if they pass:
Too good.
This exercise gave me another reason to complain about Java, which is the lambda syntax. The use of the single arrow (->) instead of the double arrow (=>) bothers me a lot.
Full Combo Hiring Verification
Statement:
Implement a system that checks whether a customer of a telecommunications company has contracted a complete combo of services. A complete combo includes the three main services offered by the company: mobile telephony, broadband and pay TV. The system must read a list of services contracted by the customer and determine whether all necessary services are included. If all three services are present, the system should return "Complete Combo". If any of the services are missing, the system should return "Incomplete Combo".
Prohibited:
A string containing the services contracted by the customer, separated by a comma. Possible values are mobile, broadband and TV.
Expected output:
A string containing Complete Combo if the client has all the services contracted, Incomplete Combo otherwise.
Examples:
Entrada | Saída |
---|---|
movel,banda larga,tv | Combo Completo |
movel,tv | Combo Incompleto |
banda larga,tv,movel | Combo Completo |
Código inicial:
import java.util.Scanner; public class VerificacaoComboCompleto { // Função para verificar se o cliente contratou um combo completo public static String verificarComboCompleto(String[] servicosContratados) { // Variáveis booleanas para verificar a contratação de cada serviço boolean movelContratado = false; boolean bandaLargaContratada = false; boolean tvContratada = false; // TODO: Itere sobre os serviços contratados for (String servico : servicosContratados) { } // TODO: Verifique se todos os serviços foram contratados if () { return "Combo Completo"; } else { return "Combo Incompleto"; } } public static void main(String[] args) { Scanner scanner = new Scanner(System.in); // Solicitando ao usuário a lista de serviços contratados String input = scanner.nextLine(); // Convertendo a entrada em uma lista de strings String[] servicosContratados = input.split(","); // Verificando se o cliente contratou um combo completo String resultado = verificarComboCompleto(servicosContratados); // Exibindo o resultado System.out.println(resultado); // Fechando o scanner scanner.close(); } }
Resolução:
De novo, esse é um desafio simples. Para chegar no resultado, apenas alguns passos precisam ser seguidos:
- Iterar sobre a array gerada pelo método main a partir da string inserida pelo usuário;
- Checar se os serviços disponibilizados (descritos nas variáveis booleanas logo acima) foram contratados;
- Em caso positivo, a variável correspondente deve ter seu valor alterado para true.
- Verificar se todos os serviços estão com o valor true. Apesar de ter mais passos, é bem mais direto que o anterior. Podemos começar a resolver esse trem de um jeito bem tosco, com uma série de ifs encadeados:
for (String servico : servicosContratados) { if(servico.equals("movel")) movelContratado = true; if(servico.equals("bandaLarga")) bandaLargaContratada = true; if(servico.equals("tv")) tvContratada = true; }
E preenchemos a condição do nosso if:
if (movelContratado && bandaLargaContratada && tvContratada) { return "Combo Completo"; } else { return "Combo Incompleto";
Assim como no primeiro, com essas adições o desafio pode ser considerado como completo, mas esses ifs, um seguido do outro me incomoda um pouco. Podemos alterar isso pra um switch pra ficar menos feio:
for (String servico : servicosContratados) { switch (servico) { case "movel": movelContratado = true; break; case "banda larga": bandaLargaContratada = true; break; case "tv": tvContratada = true; break; default: System.out.println("Serviço inválido."); break; } }
Há quem diga que os ifs são de mais fácil leitura e que o ganho que a otimização traria para um switch tão pequeno como esse é desprezível. Outros diriam que eu tenho pouca consistência interna, reclamando de checar manualmente strings em um exercício e fazendo sem um pio em outro.
Pra essas pessoas eu digo:
O código final ficaria então:
import java.util.Scanner; public class VerificacaoComboCompleto { // Função para verificar se o cliente contratou um combo completo public static String verificarComboCompleto(String[] servicosContratados) { // Variáveis booleanas para verificar a contratação de cada serviço boolean movelContratado = false; boolean bandaLargaContratada = false; boolean tvContratada = false; for (String servico : servicosContratados) { switch (servico) { case "movel": movelContratado = true; break; case "banda larga": bandaLargaContratada = true; break; case "tv": tvContratada = true; break; default: System.out.println("Serviço inválido."); break; } } if (movelContratado && bandaLargaContratada && tvContratada) { return "Combo Completo"; } else { return "Combo Incompleto"; } } public static void main(String[] args) { Scanner scanner = new Scanner(System.in); // Solicitando ao usuário a lista de serviços contratados String input = scanner.nextLine(); // Convertendo a entrada em uma lista de strings String[] servicosContratados = input.split(","); // Verificando se o cliente contratou um combo completo String resultado = verificarComboCompleto(servicosContratados); // Exibindo o resultado System.out.println(resultado); // Fechando o scanner scanner.close(); } }
Que, ao rodar a suite de testes, nos mostra que deu tudo certo:
O código desses (e dos demais) desafios está aqui.
Então é isso, pessoal. Até a próxima!
The above is the detailed content of Code Challenge - Exploring Telephony Services. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
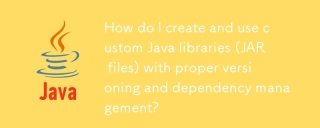
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
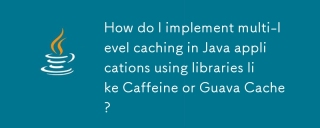
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
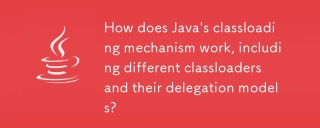
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
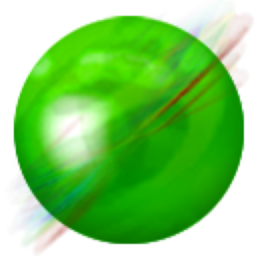
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
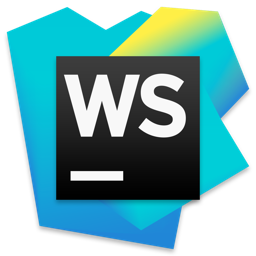
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.