


Understanding the Role of the JSON Library in Python and Its Use in Web Scraping
In Python, the "JSON" library is mainly used to process the JSON data format. JSON (JavaScript Object Notation) is a lightweight data exchange format that is easy for people to read and write, and easy for machines to parse and generate. Python's "JSON" library provides a set of simple methods to encode and decode JSON data, making it easy for Python programs to exchange data with other programs or web services.
A simple example of how to use Python's "JSON" library in web scraping
First, you need to send an HTTP request to the target website and get a response in JSON format. This can usually be done using the requests library.
Then, you can use the json library to parse this response and convert it into a Python dictionary or list so that you can easily access and manipulate the data.
Sample code:
import requests import json # Sending HTTP GET request url = 'http://www.example.com/api/data' response = requests.get(url) # Check the response status code if response.status_code == 200: # Parsing JSON Response data = json.loads(response.text) # Now you can operate on data just like a normal Python dictionary print(data) else: print('Failed to retrieve data:', response.status_code)
In this example, the json.loads() method is used to parse a JSON formatted string into a Python dictionary. If you have a Python dictionary or list and want to convert it into a JSON formatted string, you can use the json.dumps() method.
In conclusion, the Python "JSON" library is very useful in web scraping as it allows you to easily interact with web services that provide JSON responses.
How to handle JSON parsing errors?
Handling JSON parsing errors usually involves several steps to ensure that the program can handle the error situation gracefully, rather than crashing or producing unforeseen behavior. Here are some common ways to handle JSON parsing errors:
1.Error Capture
Use the try-except statement block to capture exceptions that may occur when parsing JSON. In Python, if you use json.loads() to parse a JSON string, you may encounter json.JSONDecodeError.
import json try: data = json.loads(some_json_string) except json.JSONDecodeError as e: print(f"JSON parsing error: {e}") # You can add more error handling logic here
2. Verify JSON format
Before you try to parse JSON, verify that it is well-formed. This can be done with simple string manipulation or using regular expressions, but it is usually safer to just try to parse it and catch the exception.
3. Use safe parsing functions
If you are dealing with JSON data from an untrusted source, consider writing a wrapper function that encapsulates the JSON parsing logic and provides a default behavior or return value when parsing fails.
4. Logging errors
For applications in production environments, it is important to log JSON parsing errors. This can help you track down issues and understand when and where errors occur.
5. Provide user feedback
If your application is a user interface application, make sure to provide clear feedback to the user when JSON parsing fails. This could be an error message dialog or a status update informing the user that the current operation could not be completed.
6. Check the JSON data source
If the JSON data is being obtained from an external source (such as an API), make sure that the source is reliable and that you are receiving data in the expected format. Sometimes, a source may change the format of its response, causing parsing errors.
7. Use libraries or tools for debugging
Use a library like Python's pprint to print and inspect the JSON string you are trying to parse. This can help you understand the structure of your data and identify issues that may be causing parsing failures.
8. Write robust unit tests
Write unit tests for your JSON parsing logic to ensure that it handles a variety of edge cases and potential bad inputs.
By following these steps, you can handle JSON parsing errors more effectively and ensure that your application remains stable in the face of bad data.
Should I use a proxy when using JSON web scraping?
It is not necessary to use a proxy when using JSON for web scraping. However, using a proxy can bring some benefits, such as improving access speed and stability, and helping to deal with the anti-crawler strategy of the target website. By setting up a proxy, you can simulate requests from multiple IP addresses, reduce the risk of being blocked, and increase the success rate of crawling data. In addition, the proxy can disperse requests and reduce the load on a single IP, thereby improving the request response speed.
In Python, you can set up proxies in a variety of ways, such as using the proxies parameter of the requests library, or using the ProxyHandler in the Urllib library. These methods all allow users to specify the address and port of the proxy server to use when sending network requests.
conclusion
In web scraping, the use of the JSON library is very extensive. Many web services provide responses in JSON format, so when you use Python for web scraping, you often need to parse these JSON responses to get the required data.
The above is the detailed content of Understanding the Role of the JSON Library in Python and Its Use in Web Scraping. For more information, please follow other related articles on the PHP Chinese website!
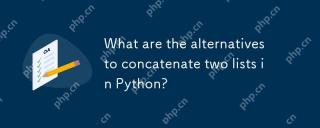
There are many methods to connect two lists in Python: 1. Use operators, which are simple but inefficient in large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use the = operator, which is both efficient and readable; 4. Use itertools.chain function, which is memory efficient but requires additional import; 5. Use list parsing, which is elegant but may be too complex. The selection method should be based on the code context and requirements.
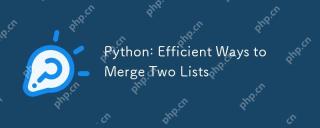
There are many ways to merge Python lists: 1. Use operators, which are simple but not memory efficient for large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use itertools.chain, which is suitable for large data sets; 4. Use * operator, merge small to medium-sized lists in one line of code; 5. Use numpy.concatenate, which is suitable for large data sets and scenarios with high performance requirements; 6. Use append method, which is suitable for small lists but is inefficient. When selecting a method, you need to consider the list size and application scenarios.
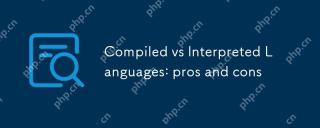
Compiledlanguagesofferspeedandsecurity,whileinterpretedlanguagesprovideeaseofuseandportability.1)CompiledlanguageslikeC arefasterandsecurebuthavelongerdevelopmentcyclesandplatformdependency.2)InterpretedlanguageslikePythonareeasiertouseandmoreportab
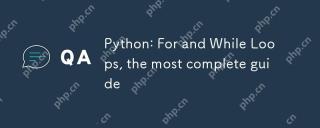
In Python, a for loop is used to traverse iterable objects, and a while loop is used to perform operations repeatedly when the condition is satisfied. 1) For loop example: traverse the list and print the elements. 2) While loop example: guess the number game until you guess it right. Mastering cycle principles and optimization techniques can improve code efficiency and reliability.
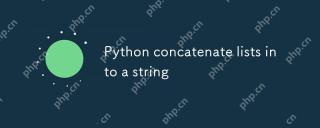
To concatenate a list into a string, using the join() method in Python is the best choice. 1) Use the join() method to concatenate the list elements into a string, such as ''.join(my_list). 2) For a list containing numbers, convert map(str, numbers) into a string before concatenating. 3) You can use generator expressions for complex formatting, such as ','.join(f'({fruit})'forfruitinfruits). 4) When processing mixed data types, use map(str, mixed_list) to ensure that all elements can be converted into strings. 5) For large lists, use ''.join(large_li
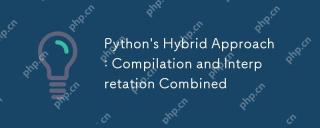
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.
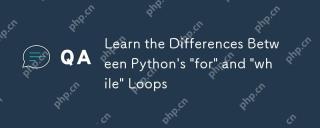
ThekeydifferencesbetweenPython's"for"and"while"loopsare:1)"For"loopsareidealforiteratingoversequencesorknowniterations,while2)"while"loopsarebetterforcontinuinguntilaconditionismetwithoutpredefinediterations.Un
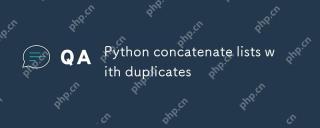
In Python, you can connect lists and manage duplicate elements through a variety of methods: 1) Use operators or extend() to retain all duplicate elements; 2) Convert to sets and then return to lists to remove all duplicate elements, but the original order will be lost; 3) Use loops or list comprehensions to combine sets to remove duplicate elements and maintain the original order.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
