


Beautiful Soup is a Python library used to scrape data from web pages. It creates a parse tree for parsing HTML and XML documents, making it easy to extract the desired information.
Beautiful Soup provides several key functionalities for web scraping:
- Navigating the Parse Tree: You can easily navigate the parse tree and search for elements, tags, and attributes.
- Modifying the Parse Tree: It allows you to modify the parse tree, including adding, removing, and updating tags and attributes.
- Output Formatting: You can convert the parse tree back into a string, making it easy to save the modified content.
To use Beautiful Soup, you need to install the library along with a parser such as lxml or html.parser. You can install them using pip
#Install Beautiful Soup using pip. pip install beautifulsoup4 lxml
Handling Pagination
When dealing with websites that display content across multiple pages, handling pagination is essential to scrape all the data.
- Identify the Pagination Structure: Inspect the website to understand how pagination is structured (e.g., next page button or numbered links).
- Iterate Over Pages: Use a loop to iterate through each page and scrape the data.
- Update the URL or Parameters: Modify the URL or parameters to fetch the next page's content.
import requests from bs4 import BeautifulSoup base_url = 'https://example-blog.com/page/' page_number = 1 all_titles = [] while True: # Construct the URL for the current page url = f'{base_url}{page_number}' response = requests.get(url) soup = BeautifulSoup(response.content, 'html.parser') # Find all article titles on the current page titles = soup.find_all('h2', class_='article-title') if not titles: break # Exit the loop if no titles are found (end of pagination) # Extract and store the titles for title in titles: all_titles.append(title.get_text()) # Move to the next page page_number += 1 # Print all collected titles for title in all_titles: print(title)
Extracting Nested Data
Sometimes, the data you need to extract is nested within multiple layers of tags. Here's how to handle nested data extraction.
- Navigate to Parent Tags: Find the parent tags that contain the nested data.
- Extract Nested Tags: Within each parent tag, find and extract the nested tags.
- Iterate Through Nested Tags: Iterate through the nested tags to extract the required information.
import requests from bs4 import BeautifulSoup url = 'https://example-blog.com/post/123' response = requests.get(url) soup = BeautifulSoup(response.content, 'html.parser') # Find the comments section comments_section = soup.find('div', class_='comments') # Extract individual comments comments = comments_section.find_all('div', class_='comment') for comment in comments: # Extract author and content from each comment author = comment.find('span', class_='author').get_text() content = comment.find('p', class_='content').get_text() print(f'Author: {author}\nContent: {content}\n')
Handling AJAX Requests
Many modern websites use AJAX to load data dynamically. Handling AJAX requires different techniques, such as monitoring network requests using browser developer tools and replicating those requests in your scraper.
import requests from bs4 import BeautifulSoup # URL to the API endpoint providing the AJAX data ajax_url = 'https://example.com/api/data?page=1' response = requests.get(ajax_url) data = response.json() # Extract and print data from the JSON response for item in data['results']: print(item['field1'], item['field2'])
Risks of Web Scraping
Web scraping requires careful consideration of legal, technical, and ethical risks. By implementing appropriate safeguards, you can mitigate these risks and conduct web scraping responsibly and effectively.
- Terms of Service Violations: Many websites explicitly prohibit scraping in their Terms of Service (ToS). Violating these terms can lead to legal actions.
- Intellectual Property Issues: Scraping content without permission may infringe on intellectual property rights, leading to legal disputes.
- IP Blocking: Websites may detect and block IP addresses that exhibit scraping behavior.
- Account Bans: If scraping is performed on websites requiring user authentication, the account used for scraping might get banned.
Beautiful Soup is a powerful library that simplifies the process of web scraping by providing an easy-to-use interface for navigating and searching HTML and XML documents. It can handle various parsing tasks, making it an essential tool for anyone looking to extract data from the web.
The above is the detailed content of How Beautiful Soup is used to extract data out of the Public Web. For more information, please follow other related articles on the PHP Chinese website!
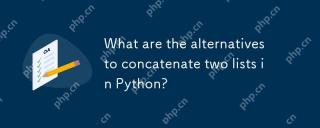
There are many methods to connect two lists in Python: 1. Use operators, which are simple but inefficient in large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use the = operator, which is both efficient and readable; 4. Use itertools.chain function, which is memory efficient but requires additional import; 5. Use list parsing, which is elegant but may be too complex. The selection method should be based on the code context and requirements.
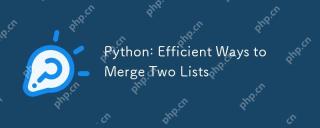
There are many ways to merge Python lists: 1. Use operators, which are simple but not memory efficient for large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use itertools.chain, which is suitable for large data sets; 4. Use * operator, merge small to medium-sized lists in one line of code; 5. Use numpy.concatenate, which is suitable for large data sets and scenarios with high performance requirements; 6. Use append method, which is suitable for small lists but is inefficient. When selecting a method, you need to consider the list size and application scenarios.
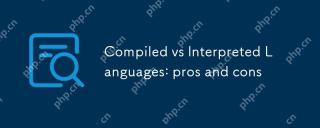
Compiledlanguagesofferspeedandsecurity,whileinterpretedlanguagesprovideeaseofuseandportability.1)CompiledlanguageslikeC arefasterandsecurebuthavelongerdevelopmentcyclesandplatformdependency.2)InterpretedlanguageslikePythonareeasiertouseandmoreportab
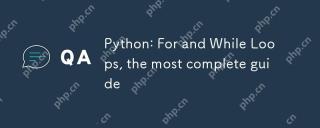
In Python, a for loop is used to traverse iterable objects, and a while loop is used to perform operations repeatedly when the condition is satisfied. 1) For loop example: traverse the list and print the elements. 2) While loop example: guess the number game until you guess it right. Mastering cycle principles and optimization techniques can improve code efficiency and reliability.
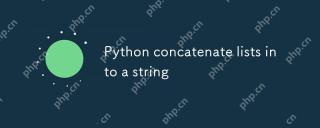
To concatenate a list into a string, using the join() method in Python is the best choice. 1) Use the join() method to concatenate the list elements into a string, such as ''.join(my_list). 2) For a list containing numbers, convert map(str, numbers) into a string before concatenating. 3) You can use generator expressions for complex formatting, such as ','.join(f'({fruit})'forfruitinfruits). 4) When processing mixed data types, use map(str, mixed_list) to ensure that all elements can be converted into strings. 5) For large lists, use ''.join(large_li
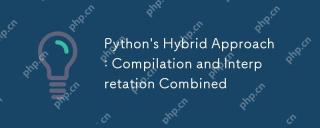
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.
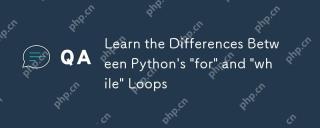
ThekeydifferencesbetweenPython's"for"and"while"loopsare:1)"For"loopsareidealforiteratingoversequencesorknowniterations,while2)"while"loopsarebetterforcontinuinguntilaconditionismetwithoutpredefinediterations.Un
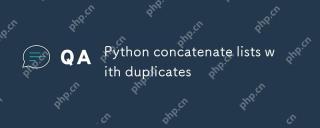
In Python, you can connect lists and manage duplicate elements through a variety of methods: 1) Use operators or extend() to retain all duplicate elements; 2) Convert to sets and then return to lists to remove all duplicate elements, but the original order will be lost; 3) Use loops or list comprehensions to combine sets to remove duplicate elements and maintain the original order.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
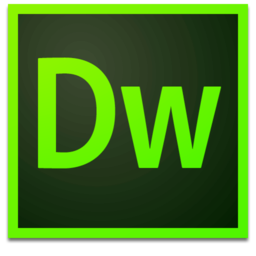
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
