You might be curious about how proxy servers work and how they serve data over the internet. In this blog, I am going to implement a proxy server using core NodeJs.I achieved this using a core NodeJs package called net which already comes with NodeJs.
How Proxy Works
a proxy server is an agent between the client and the server. When a
client sends a request to a server it is forwarded to a target proxy
server. The targeted proxy server processes the request and sends it
to the main server and the main server sends the back request to the
proxy server and the proxy server sends the request to the client.
Set up Proxy Server
Before, starting programming you know little about socket and NodeJs.You know what a is socket and how they work.
In NodeJs There are two methods to implement a proxy server. First I custom method and second in-build. Both are easy to understand.
To test your proxy server you can run your local HTTP serve on your local machine and then target the host machine.
- Now, I will define the net package and then write the target server and port number.
const net = require('net'); // Define the target host and port const targetHost = 'localhost'; // Specify the hostname of the target server. For Ex: (12.568.45.25) const targetPort = 80; // Specify the port of the target server
- Creating and listening TCP server.
const server = net.createServer((clientSocket) => { }); // Start listening for incoming connections on the specified port const proxyPort = 3000; // Specify the port for the proxy server server.listen(proxyPort, () => { console.log(`Reverse proxy server is listening on port ${proxyPort}`); });
- we have created a server using the net package. it's a TCP server which is running port number 3000 as we define in using the proxyPort variable. if the server starts it will show the Reverse proxy server is listening on port 3000 message on the console.
- when the client tries to connect the proxy server it runs the callback function which is inside createServer.
In server function callback we have one parameter clientSocket it's the user connection that we received for the connection.
accept and receive data from the user
const targetHost = 'localhost'; // Specify the hostname of the target server. For Ex: (12.568.45.25) const targetPort = 80; // Specify the port of the target server // Create a TCP server const server = net.createServer((clientSocket) => { // Establish a connection to the target host net.createConnection({host: targetHost, port: targetPort}, () => { // When data is received from the client, write it to the target server clientSocket.on("data", (data) => { targetSocket.write(data); }); // When data is received from the target server, write it back to the client targetSocket.on("data", (data) => { clientSocket.write(data); }); }); });
- When a client tries to connect proxy server we will create a temporary connection to the server using create createConnection
-
createServer have 2 arguments
- 1st Host where we want to connect in this case we have to connect to our server host which is defined in targetHost variable.
- 2nd Port where we want to connect in this case we have to connect to our server port which is defined in targetPort variable.
- Now, When user send data I will pass this data to server. using this code
clientSocket.on("data", (data) => { targetSocket.write(data); });
- Then, the Server sends data I will send this data to the user, using this code
targetSocket.on("data", (data) => { clientSocket.write(data); });
- Congratulations! you successfully created your proxy server.
Error Handling
While exploring the functionality of the proxy server is exciting, ensuring reliability requires robust error-handling methods to handle unexpected issues gracefully. To handle those types of errors we have an event called an error. It's very easy to implement.
const server = net.createServer((clientSocket) => { // Establish a connection to the target host const targetSocket = net.createConnection({host: targetHost,port: targetPort}, () => { // Handle errors when connecting to the target server targetSocket.on('error', (err) => { console.error('Error connecting to target:', err); clientSocket.end(); // close connection }); // Handle errors related to the client socket clientSocket.on('error', (err) => { console.error('Client socket error:', err); targetSocket.end(); // close connection }); }); });
- when any error occurs we will console the error and then close the connection.
ALL Code
- replace host name and port number according to your preference.
const net = require('net'); // Define the target host and port const targetHost = 'localhost'; // Specify the hostname of the target server const targetPort = 80; // Specify the port of the target server // Create a TCP server const server = net.createServer((clientSocket) => { // Establish a connection to the target host const targetSocket = net.createConnection({ host: targetHost, port: targetPort }, () => { // When data is received from the target server, write it back to the client targetSocket.on("data", (data) => { clientSocket.write(data); }); // When data is received from the client, write it to the target server clientSocket.on("data", (data) => { targetSocket.write(data); }); }); // Handle errors when connecting to the target server targetSocket.on('error', (err) => { console.error('Error connecting to target:', err); clientSocket.end(); }); // Handle errors related to the client socket clientSocket.on('error', (err) => { console.error('Client socket error:', err); targetSocket.end(); }); }); // Start listening for incoming connections on the specified port const proxyPort = 3000; // Specify the port for the proxy server server.listen(proxyPort, () => { console.log(`Reverse proxy server is listening on port ${proxyPort}`); });
Built Method
To reduce client and server connection complexity we have built-in method pipe.I will replace this syntax
// Handle errors when connecting to the target server targetSocket.on("data", (data) => { clientSocket.write(data); }); // When data is received from the client, write it to the target server clientSocket.on("data", (data) => { targetSocket.write(data); });
Into this syntax
// Pipe data from the client to the target clientSocket.pipe(targetSocket); // When data is received from the client, write it to the target server targetSocket.pipe(clientSocket);
Here is all code
const net = require('net'); // Define the target host and port const targetHost = 'localhost'; const targetPort = 80; // Create a TCP server const server = net.createServer((clientSocket) => { // Establish a connection to the target host const targetSocket = net.createConnection({ host: targetHost, port: targetPort }, () => { // Pipe data from the client to the target clientSocket.pipe(targetSocket); // Pipe data from the target to the client targetSocket.pipe(clientSocket); }); // Handle errors targetSocket.on('error', (err) => { console.error('Error connecting to target:', err); clientSocket.end(); }); clientSocket.on('error', (err) => { console.error('Client socket error:', err); targetSocket.end(); }); }); // Start listening for incoming connections const proxyPort = 3000; server.listen(proxyPort, () => { console.log(`Reverse proxy server is listening on port ${proxyPort}`); });
A Working Proxy Server!
Follow Me On GitHub avinashtare, Thanks.
The above is the detailed content of How I Build a Scratch Proxy Server Using Node.js. For more information, please follow other related articles on the PHP Chinese website!
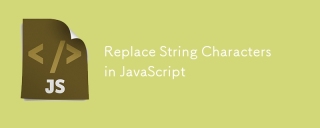
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
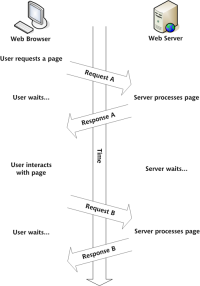
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
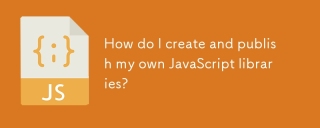
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.

The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
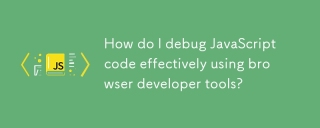
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
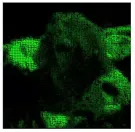
Bring matrix movie effects to your page! This is a cool jQuery plugin based on the famous movie "The Matrix". The plugin simulates the classic green character effects in the movie, and just select a picture and the plugin will convert it into a matrix-style picture filled with numeric characters. Come and try it, it's very interesting! How it works The plugin loads the image onto the canvas and reads the pixel and color values: data = ctx.getImageData(x, y, settings.grainSize, settings.grainSize).data The plugin cleverly reads the rectangular area of the picture and uses jQuery to calculate the average color of each area. Then, use
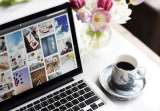
This article will guide you to create a simple picture carousel using the jQuery library. We will use the bxSlider library, which is built on jQuery and provides many configuration options to set up the carousel. Nowadays, picture carousel has become a must-have feature on the website - one picture is better than a thousand words! After deciding to use the picture carousel, the next question is how to create it. First, you need to collect high-quality, high-resolution pictures. Next, you need to create a picture carousel using HTML and some JavaScript code. There are many libraries on the web that can help you create carousels in different ways. We will use the open source bxSlider library. The bxSlider library supports responsive design, so the carousel built with this library can be adapted to any
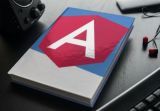
Data sets are extremely essential in building API models and various business processes. This is why importing and exporting CSV is an often-needed functionality.In this tutorial, you will learn how to download and import a CSV file within an Angular


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
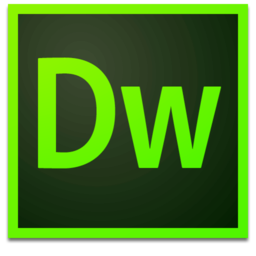
Dreamweaver Mac version
Visual web development tools
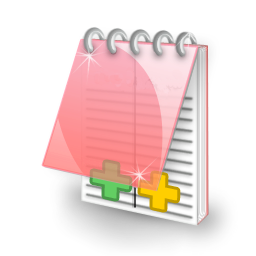
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.