C++ algorithm efficiency improvement: sharing of practical skills
Tips to improve the efficiency of C++ algorithms: Use appropriate data structures, such as std::vector and std::unordered_map. Avoid unnecessary copies, use references or pointers. Take advantage of compiler optimization flags such as -O3. Optimization algorithms, such as using pruning techniques.
Improvement of C++ algorithm efficiency: sharing of practical skills
Improvement of C++ algorithm efficiency is crucial, especially when processing large data sets or time-sensitive tasks. This article will share some practical tips to help you achieve better efficiency in your code.
1. Use the correct container
Choosing the appropriate container data structure can significantly affect algorithm efficiency. For example, if you need fast element lookup for random access, use std::vector
. For operations that require frequent insertion and deletion, consider using std::unordered_map
.
Practical case:
// 使用 std::unordered_map 加快查找速度 std::unordered_map<int, int> myMap; int value = myMap[key]; // 使用 std::vector 快速遍历 std::vector<int> myVector; for (int i = 0; i < myVector.size(); ++i) { int value = myVector[i]; }
2. Avoid unnecessary copies
Copying objects in C++ may lead to inefficiency Low, especially when working with large objects. By using a reference or pointer to refer to an object, you can avoid unnecessary copies.
Practical case:
// 通过引用传递对象,避免拷贝 void myFunction(std::vector<int>& myVector) { // 在 myFunction 内修改 myVector } // 通过指针传递对象,避免拷贝 void myFunction(std::vector<int>* myVector) { // 在 myFunction 内修改 *myVector }
3. Use compiler optimization
C++ compiler can usually optimize code and improve operation time efficiency. Here are some flags you can use to enable compiler optimizations:
-
-O0
: Turn off optimization (for debugging) -
-O1
: Basic optimization -
-O2
: Higher level optimization -
-O3
: Highest level optimization (may result in longer compilation Time)
Practical case:
Add the following flags in the compilation command:
g++ -std=c++11 -O3 myCode.cpp -o myCode
4. Optimization algorithm
The improvement of specific algorithm efficiency depends on the algorithm itself. The following are some general algorithm optimization techniques:
- Use pruning technology to reduce the search space
- Use the characteristics of the data structure (for example, the search efficiency of a binary tree is O(log n))
- Parallelize the algorithm to take advantage of multi-core processors
Practical example:
For a search algorithm to find a specific element, you can use the following Pruning Techniques:
// 剪枝技术:如果元素不在当前子树中,则不必进一步搜索 if (element > maxValueInCurrentSubtree) { return; }
Conclusion:
By applying these techniques, you can achieve significant algorithmic efficiency improvements in your C++ code. By using the right containers, avoiding unnecessary copies, taking advantage of compiler optimizations, and optimizing the algorithms themselves, you can build faster, more efficient applications.
The above is the detailed content of C++ algorithm efficiency improvement: sharing of practical skills. For more information, please follow other related articles on the PHP Chinese website!
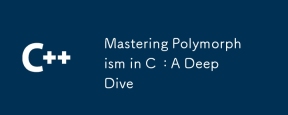
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
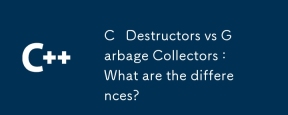
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
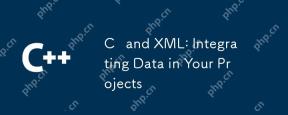
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
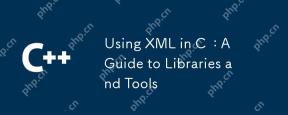
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
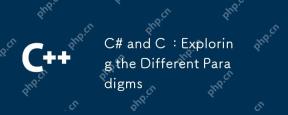
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
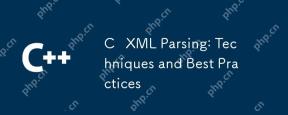
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
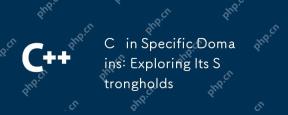
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
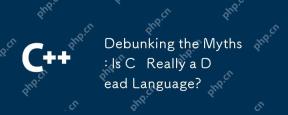
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
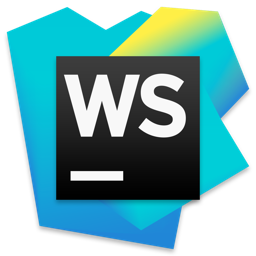
WebStorm Mac version
Useful JavaScript development tools
