Spin lock is a lightweight lock used to protect shared resources. It obtains it by continuously polling the status of the lock to avoid context switching. The advantages include high efficiency, responsiveness, and scalability, but the disadvantages are that it may cause CPU waste and is not suitable for long-term locking situations.
Spin lock in C++ multi-threaded programming
Introduction
The spin lock is a lightweight lock. When a thread Used when trying to access a shared resource, it avoids context switches by always polling the status of the lock.
Principle
The working principle of a spin lock is: when a thread tries to acquire a lock, it will continuously check the status of the lock. If the lock is released, the thread acquires it immediately. If the lock has been acquired by another thread, the thread will continue to poll the lock's status until it is released.
Advantages
- High efficiency: Spin lock is more efficient than other locking mechanisms (such as mutex locks) because it avoids expensive context switches.
- Responsive: When a thread continuously polls the status of a lock, it can quickly react to the release of the lock.
- Strong scalability: Spin locks perform well in multi-processor systems because each thread can spin on its own cache line.
Limitations
- May cause CPU waste: If a lock is frequently contested, continuously polling the lock status may cause Wastes a lot of CPU resources.
- Not applicable to long-term lock situations: If a lock is held for a long time, the spin lock may cause thread starvation.
Practical case
The following code example demonstrates how to use std::atomic<bool></bool>
in C++ to implement a spin lock:
#include <atomic> class Spinlock { private: std::atomic<bool> locked; public: Spinlock() : locked(false) {} void lock() { while (locked.exchange(true)) { /* 旋转直到锁被释放 */ } } void unlock() { locked.store(false); } }; int main() { Spinlock lock; // 创建多个线程来争用锁 std::vector<std::thread> threads; for (int i = 0; i < 10; i++) { threads.push_back(std::thread([&lock] { lock.lock(); // 访问共享资源 lock.unlock(); })); } // 等待所有线程完成 for (std::thread& thread : threads) { thread.join(); } return 0; }
Conclusion
Spin lock is a powerful synchronization primitive that can be used to protect shared resources in multi-threaded C++ programs. However, they can cause CPU waste when locks are frequently contested, so caution is needed when using them.
The above is the detailed content of What is the role of spinlocks in C++ multi-threaded programming?. For more information, please follow other related articles on the PHP Chinese website!
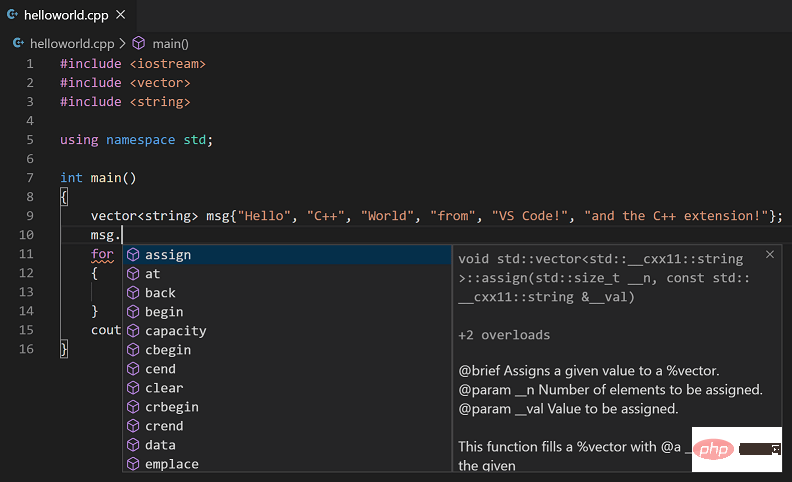
C++是一种广泛使用的面向对象的计算机编程语言,它支持您与之交互的大多数应用程序和网站。你需要编译器和集成开发环境来开发C++应用程序,既然你在这里,我猜你正在寻找一个。我们将在本文中介绍一些适用于Windows11的C++编译器的主要推荐。许多审查的编译器将主要用于C++,但也有许多通用编译器您可能想尝试。MinGW可以在Windows11上运行吗?在本文中,我们没有将MinGW作为独立编译器进行讨论,但如果讨论了某些IDE中的功能,并且是DevC++编译器的首选
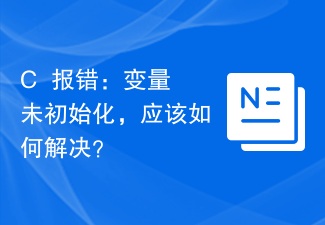
在C++程序开发中,当我们声明了一个变量但是没有对其进行初始化,就会出现“变量未初始化”的报错。这种报错经常会让人感到很困惑和无从下手,因为这种错误并不像其他常见的语法错误那样具体,也不会给出特定的代码行数或者错误类型。因此,下面我们将详细介绍变量未初始化的问题,以及如何解决这个报错。一、什么是变量未初始化错误?变量未初始化是指在程序中声明了一个变量但是没有
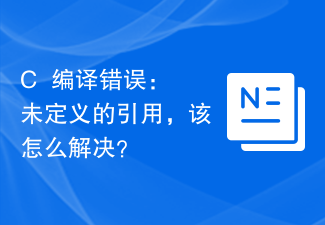
C++是一门广受欢迎的编程语言,但是在使用过程中,经常会出现“未定义的引用”这个编译错误,给程序的开发带来了诸多麻烦。本篇文章将从出错原因和解决方法两个方面,探讨“未定义的引用”错误的解决方法。一、出错原因C++编译器在编译一个源文件时,会将它分为两个阶段:编译阶段和链接阶段。编译阶段将源文件中的源码转换为汇编代码,而链接阶段将不同的源文件合并为一个可执行文
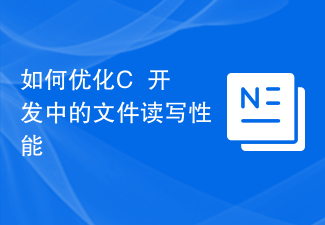
如何优化C++开发中的文件读写性能在C++开发过程中,文件的读写操作是常见的任务之一。然而,由于文件读写是磁盘IO操作,相对于内存IO操作来说会更为耗时。为了提高程序的性能,我们需要优化文件读写操作。本文将介绍一些常见的优化技巧和建议,帮助开发者在C++文件读写过程中提高性能。使用合适的文件读写方式在C++中,文件读写可以通过多种方式实现,如C风格的文件IO
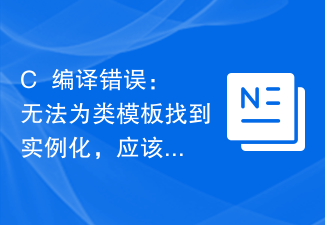
C++是一门强大的编程语言,它支持使用类模板来实现代码的复用,提高开发效率。但是在使用类模板时,可能会遭遇编译错误,其中一个比较常见的错误是“无法为类模板找到实例化”(error:cannotfindinstantiationofclasstemplate)。本文将介绍这个问题的原因以及如何解决。问题描述在使用类模板时,有时会遇到以下错误信息:e
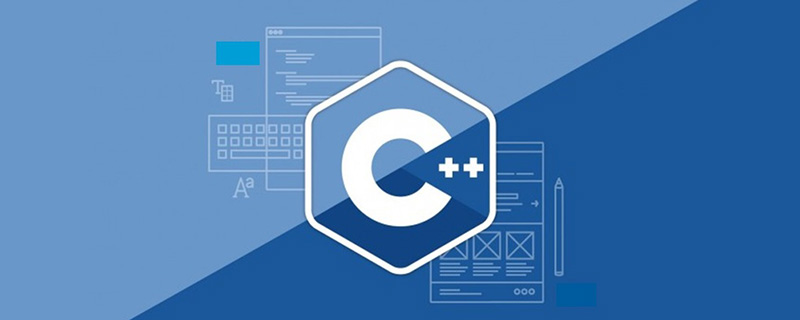
iostream头文件包含了操作输入输出流的方法,比如读取一个文件,以流的方式读取;其作用是:让初学者有一个方便的命令行输入输出试验环境。iostream的设计初衷是提供一个可扩展的类型安全的IO机制。
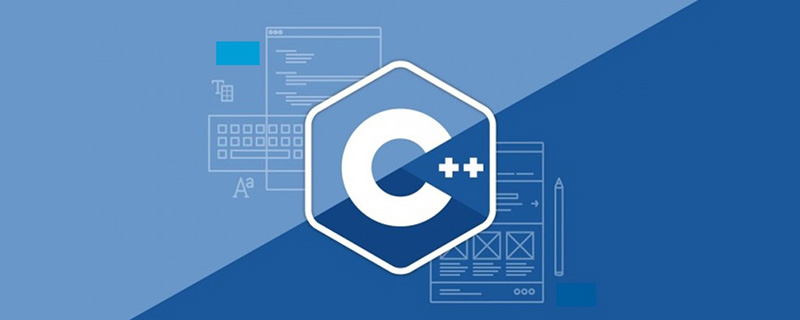
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。
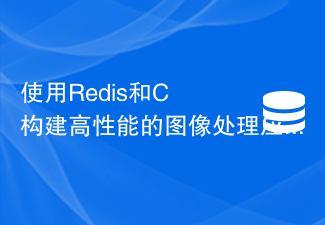
使用Redis和C++构建高性能的图像处理应用图像处理是现代计算机应用中的重要环节之一。由于图像处理的复杂性和计算量大,如何在保证高性能的同时提供稳定的服务是一个挑战。本文将介绍如何使用Redis和C++构建高性能的图像处理应用,并提供一些代码示例。Redis是一个开源的内存数据库,具有高性能和高可用性的特点。它支持各种数据结构,如字符串、哈希表、列表等,同


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
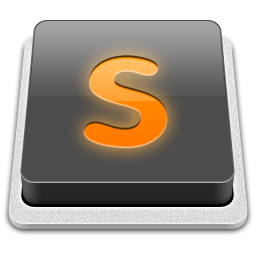
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
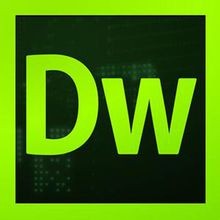
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
