A memory leak in C++ means that the program allocates memory but forgets to release it, causing the memory to not be reused. Debugging techniques include using debuggers (such as Valgrind, GDB), inserting assertions, and using memory leak detector libraries (such as Boost.LeakDetector, MemorySanitizer). It demonstrates the use of Valgrind to detect memory leaks through practical cases, and proposes best practices to avoid memory leaks, including: always freeing allocated memory, using smart pointers, using memory management libraries, and performing regular memory checks.
Debugging techniques for memory leaks in C++
In C++, a memory leak means that the program allocates memory but forgets to release it, causing the memory to not be reused. This causes the program's memory usage to increase, eventually leading to a crash.
Debugging techniques
The following techniques are available for debugging memory leaks:
-
Use debugger:
- Valgrind: A tool designed for memory error detection, detecting leaks and free-after-access errors.
-
GDB: Leaks can be detected using the
info leaks
command.
-
Insert assertion:
- Add an assertion in the destructor to check whether the destructor is called, indicating The memory has been released.
-
Use a memory leak detector library:
- such as
Boost.LeakDetector
andMemorySanitizer
, these libraries automatically detect and report leaks.
- such as
Practical Case
The following example shows how to use Valgrind to detect memory leaks:
#include <iostream> #include <stdlib.h> using namespace std; int main() { // 分配内存 int* ptr = (int*) malloc(sizeof(int)); // 使用内存 // 忘记释放内存 return 0; }
When compiling and running this program, Valgrind will Report a memory leak:
==4620== Memcheck, a memory error detector ==4620== Copyright (C) 2002-2017, and GNU GPL'd, by Julian Seward et al. ==4620== Using Valgrind-3.13.0 and LibVEX; rerun with -h for copyright info ==4620== Command: ./memleak ==4620== ==4620== malloc/free: in use at exit: 4 bytes in 1 blocks ==4620== malloc/free: 4 bytes in 1 blocks are definitely lost in loss record 1 of 1 ==4620== at 0x48439D7: malloc (in /usr/lib/x86_64-linux-gnu/valgrind/vgpreload_memcheck-amd64-linux.so) ==4620== by 0x400647: main (memleak.cpp:9)
This indicates that the program leaked 4 bytes of memory, located at line 9 of memleak.cpp
.
Avoiding memory leaks
Best practices to avoid memory leaks include:
-
Always free allocated memory: When it is no longer needed , use
delete
orfree
to release the memory pointed to by the pointer. -
Use smart pointers: Use smart pointers such as
std::unique_ptr
orstd::shared_ptr
, which automatically manage memory release. -
Use memory management libraries: Such as
Smart pointer factory
andMemory pool
. - Perform regular memory checks: Periodically check for memory leaks while your program is running so you can fix them before they become a problem.
The above is the detailed content of Debugging techniques for memory leaks in C++. For more information, please follow other related articles on the PHP Chinese website!
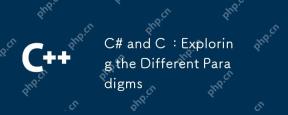
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
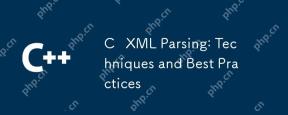
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
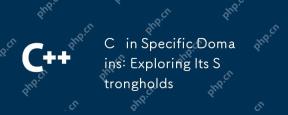
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
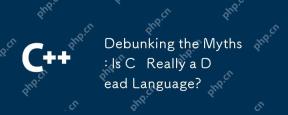
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
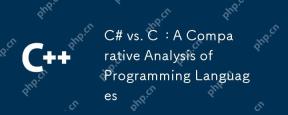
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
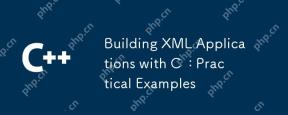
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
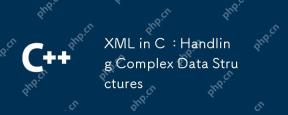
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
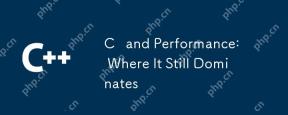
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
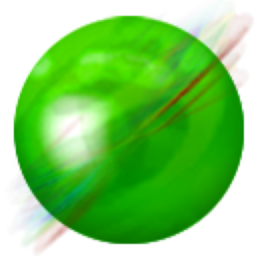
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
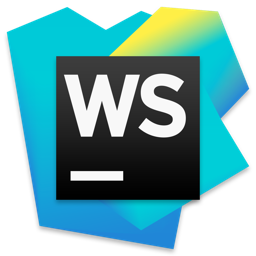
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
