


What are the considerations in cross-platform and heterogeneous system environments in C++ concurrent programming?
Concurrent programming in C++ across platforms and heterogeneous systems requires consideration of the following differences: Cross-platform considerations: Multithreading API differences (POSIX, Windows) Atomic operations semantic memory models (sequential consistency, loose consistency) Deadlocks and Starvation Issue Lock Implementation Performance Differences Heterogeneous System Considerations: Heterogeneous Processing Architectures (x86, ARM) Hardware Accelerators (GPUs) Network Topology and Latency Virtualization and Containerization Portability and Maintainability
Cross-platform and heterogeneous system considerations in C++ concurrent programming
In today's interconnected world, cross-platform and heterogeneous system environments have become common issues that developers need to deal with. When it comes to concurrent programming, developers must carefully consider the differences between these systems to achieve cross-platform compatibility and performance.
Cross-platform considerations
- Multi-threading API differences: POSIX, Windows and other operating systems provide different multi-threading APIs that need to be targeted at different platforms Adapt.
- Atomic operation semantics: Different platforms have different semantics for atomic operations (such as loading and storing), and their impact on inter-thread synchronization must be considered.
- Memory model: Cross-platform concurrent programming requires understanding the memory models of different platforms (for example, sequential consistency and loose consistency) to ensure the visibility and consistency of data between threads .
- Deadlock and starvation: Deadlock and starvation problems in multi-threaded applications may exhibit different symptoms on heterogeneous systems, and developers need to take appropriate precautions.
- Lock implementation: Lock implementations (such as mutex locks and read-write locks) on different platforms may have different performance characteristics and need to be optimized for specific systems.
Heterogeneous system considerations
- Heterogeneous processing architecture: x86, ARM and other CPU architectures have a great impact on the performance of concurrent programming. Developers are asked to optimize their code for different architectures.
- Hardware accelerators: Heterogeneous systems may contain hardware accelerators (such as GPUs), and the use of these accelerators in concurrent programming needs to be considered.
- Network topology: Network topology and latency are crucial in concurrent programming in distributed heterogeneous systems, and developers need to consider these factors to optimize communication and synchronization.
- Virtualization and containerization: Technologies such as virtual machines and containers will introduce additional complexity, affect concurrent programming on heterogeneous systems, and require specific processing.
- Portability: Concurrent code on heterogeneous systems must be easily portable and maintainable to deploy and run on different platforms and architectures.
Practical Case
Consider the following C++ code example for implementing a thread-safe queue in cross-platform and heterogeneous systems:
#include <atomic> #include <queue> template<typename T> class ThreadSafeQueue { private: std::atomic_bool locked = false; std::queue<T> data; public: void push(const T& item) { while (locked.load()) {} locked.store(true); data.push(item); locked.store(false); } T pop() { while (locked.load()) {} locked.store(true); T item = data.front(); data.pop(); locked.store(false); return item; } };
This implementation uses the C++ standard library Atomic operations and queue types provide thread safety across platforms and heterogeneous system environments.
The above is the detailed content of What are the considerations in cross-platform and heterogeneous system environments in C++ concurrent programming?. For more information, please follow other related articles on the PHP Chinese website!
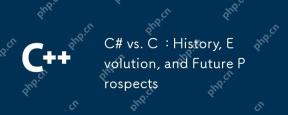
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
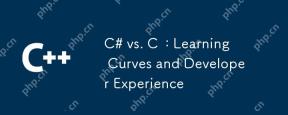
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
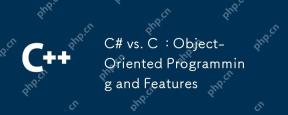
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
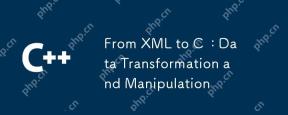
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
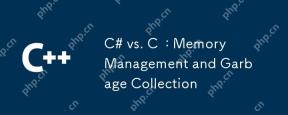
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
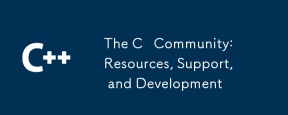
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
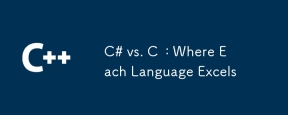
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 English version
Recommended: Win version, supports code prompts!
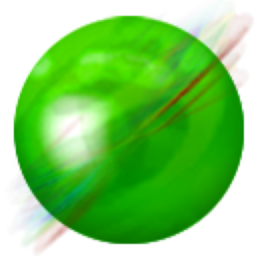
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool