


How to choose the best golang framework for different application scenarios
Choose the best Go framework based on application scenarios: consider application type, language features, performance requirements, and ecosystem. Common Go frameworks: Gin (Web application), Echo (Web service), Fiber (high throughput), gorm (ORM), fasthttp (speed). Practical case: building REST API (Fiber) and interacting with database (gorm). Choose a framework: choose fasthttp for key performance, Gin/Echo for flexible web applications, and gorm for database interaction.
How to choose the best Go framework for different application scenarios
Introduction
The Go language is known for its speed, concurrency, and powerful standard library. It is widely used to develop a variety of applications, from web servers to distributed systems. To simplify application development and provide common functionality, the Go community has developed a wide range of frameworks. This guide is designed to help you choose the most appropriate Go framework for your specific application scenario.
Framework considerations
When choosing a Go framework, you need to consider the following key factors:
- Application type: The framework should support the type of application you are developing, such as a web application, API service, or CLI tool.
- Supported Language Features: The framework should support the Go language features you intend to use in your application, such as generics or concurrency.
- Performance Requirements: The performance requirements you have for your application will influence the framework you choose.
- Ecosystem and Support: An active community, rich documentation, and third-party library support are critical to the long-term success of the framework.
Common Go framework
- **Gin: Used to build fast, flexible web applications.
- **Echo: Another popular web framework known for its lightweight and scalability.
- **Fiber: A high-performance web framework optimized for extremely high throughput and low latency.
- **gorm: A powerful ORM (Object Relational Mapping) framework for interacting with databases.
- **fasthttp: A high-performance HTTP server and client library focused on speed and scalability.
Practical case
Building a simple REST API (Fiber)
package main import ( "log" "github.com/gofiber/fiber/v2" "github.com/gofiber/fiber/v2/middleware/logger" ) func main() { // 创建一个 Fiber 应用程序 app := fiber.New() // 使用 Logger 中间件记录请求 app.Use(logger.New()) // 定义一个处理 GET 请求的路由 app.Get("/api/v1/users", func(c *fiber.Ctx) error { return c.SendString("Hello, World!") }) // 监听端口 3000 log.Fatal(app.Listen("0.0.0.0:3000")) }
With database Interaction (gorm)
package main import ( "fmt" "gorm.io/driver/sqlite" "gorm.io/gorm" ) type User struct { ID uint Username string Email string } func main() { // 连接到 SQLite 数据库 db, err := gorm.Open(sqlite.Open("database.db"), &gorm.Config{}) if err != nil { log.Fatal(err) } // 创建一个新的用户 user := User{Username: "johndoe", Email: "johndoe@example.com"} db.Create(&user) // 查找所有用户 users := []User{} db.Find(&users) // 打印用户信息 fmt.Println("Users:", users) }
Choose the appropriate framework
In short, choosing the best Go framework depends on the specific requirements of the application scenario. For performance-critical applications, fasthttp is a good choice. For flexible and scalable web applications, Gin or Echo are a good choice. Gorm is a powerful ORM framework for applications that need to interact with databases. By considering framework considerations and evaluating real-world use cases, you can make informed choices and build successful Go applications.
The above is the detailed content of How to choose the best golang framework for different application scenarios. For more information, please follow other related articles on the PHP Chinese website!
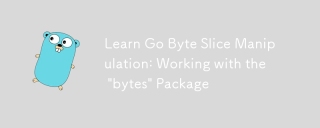
ThebytespackageinGoisessentialformanipulatingbytesliceseffectively.1)Usebytes.Jointoconcatenateslices.2)Employbytes.Bufferfordynamicdataconstruction.3)UtilizeIndexandContainsforsearching.4)ApplyReplaceandTrimformodifications.5)Usebytes.Splitforeffici
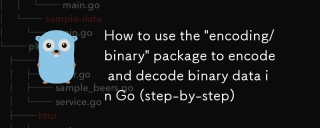
Tousethe"encoding/binary"packageinGoforencodinganddecodingbinarydata,followthesesteps:1)Importthepackageandcreateabuffer.2)Usebinary.Writetoencodedataintothebuffer,specifyingtheendianness.3)Usebinary.Readtodecodedatafromthebuffer,againspeci
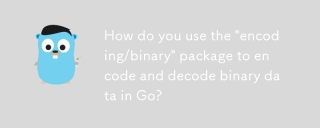
The encoding/binary package provides a unified way to process binary data. 1) Use binary.Write and binary.Read functions to encode and decode various data types such as integers and floating point numbers. 2) Custom types can be handled by implementing the binary.ByteOrder interface. 3) Pay attention to endianness selection, data alignment and error handling to ensure the correctness and efficiency of the data.

Go's strings package is not suitable for all use cases. It works for most common string operations, but third-party libraries may be required for complex NLP tasks, regular expression matching, and specific format parsing.
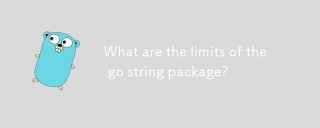
The strings package in Go has performance and memory usage limitations when handling large numbers of string operations. 1) Performance issues: For example, strings.Replace and strings.ReplaceAll are less efficient when dealing with large-scale string replacements. 2) Memory usage: Since the string is immutable, new objects will be generated every operation, resulting in an increase in memory consumption. 3) Unicode processing: It is not flexible enough when handling complex Unicode rules, and may require the help of other packages or libraries.
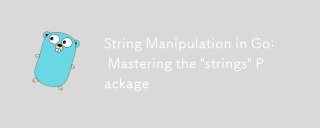
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
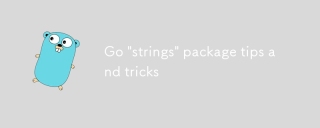
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
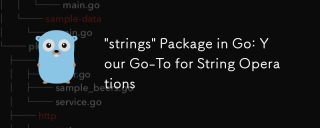
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
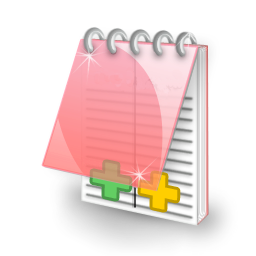
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
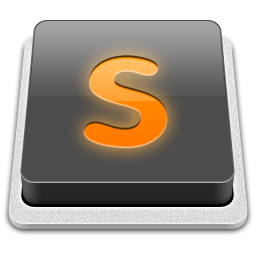
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
