Manage C++ memory leaks in embedded systems by identifying leaks using a memory analysis tool such as Valgrind. Automatically release resources using the RAII design pattern. Automatically manage object lifecycle using smart pointers. Track object references using a reference count and release the object when the reference count reaches 0.
Managing memory leaks in C++ in embedded systems
Introduction
Memory leak means that the memory allocated during the running of the program is no longer accessed or used. It is a serious problem that can lead to application inefficiency, instability, and even operating system crashes. Memory management is particularly important in embedded systems because resources are limited and the impact of memory leaks may be more serious.
Identifying memory leaks
One way to identify memory leaks is to use a memory analysis tool. These tools can monitor memory allocation and deallocation and help determine the source of memory leaks. Valgrind is a popular open source memory analysis tool that can be used to detect memory leaks in C++ programs.
Manage memory leaks
Effective ways to manage memory leaks are as follows:
- Use RAII: Resource acquisition i.e. Initialization (RAII) is a design pattern for automatically allocating resources when an object is created and releasing them when the object is destroyed. This helps prevent memory leaks because resources will be automatically released when no longer needed.
- Using smart pointers: A smart pointer is a C++ template that encapsulates a raw pointer and automatically manages the pointer's life cycle. When a smart pointer goes out of scope, it automatically releases the object it points to, thus preventing memory leaks.
- Using reference counting: Reference counting is a technique for tracking the number of times an object is referenced. When an object's reference count drops to 0, the object is released. This prevents useless objects from remaining in memory, causing memory leaks.
Practical case
Consider the following C++ code example:
class MyClass { public: int* data; MyClass() { data = new int; } ~MyClass() { delete data; } }; int main() { MyClass* obj = new MyClass; // 由于忘记释放 obj,导致内存泄漏 return 0; }
If you forget to release obj
, it will cause memory loss leakage. To prevent this, you can use smart pointers:
class MyClass { public: std::unique_ptr<int> data; MyClass() { data = std::make_unique<int>(); } }; int main() { std::unique_ptr<MyClass> obj = std::make_unique<MyClass>(); // obj 在超出范围时会自动释放,无需手动调用 delete return 0; }
By using smart pointers, you can eliminate memory leaks caused by forgetting to release objects.
The above is the detailed content of Managing memory leaks in C++ in embedded systems. For more information, please follow other related articles on the PHP Chinese website!
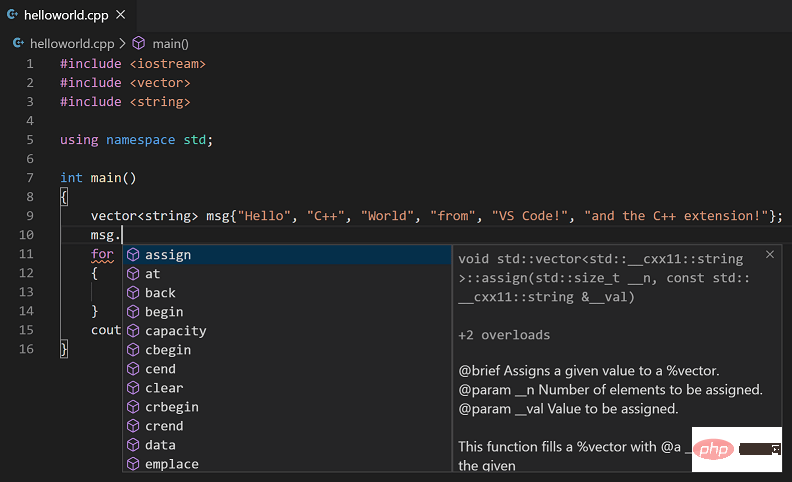
C++是一种广泛使用的面向对象的计算机编程语言,它支持您与之交互的大多数应用程序和网站。你需要编译器和集成开发环境来开发C++应用程序,既然你在这里,我猜你正在寻找一个。我们将在本文中介绍一些适用于Windows11的C++编译器的主要推荐。许多审查的编译器将主要用于C++,但也有许多通用编译器您可能想尝试。MinGW可以在Windows11上运行吗?在本文中,我们没有将MinGW作为独立编译器进行讨论,但如果讨论了某些IDE中的功能,并且是DevC++编译器的首选
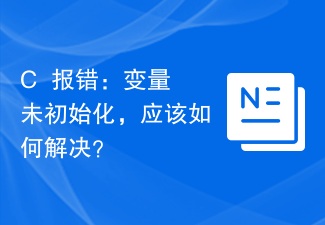
在C++程序开发中,当我们声明了一个变量但是没有对其进行初始化,就会出现“变量未初始化”的报错。这种报错经常会让人感到很困惑和无从下手,因为这种错误并不像其他常见的语法错误那样具体,也不会给出特定的代码行数或者错误类型。因此,下面我们将详细介绍变量未初始化的问题,以及如何解决这个报错。一、什么是变量未初始化错误?变量未初始化是指在程序中声明了一个变量但是没有
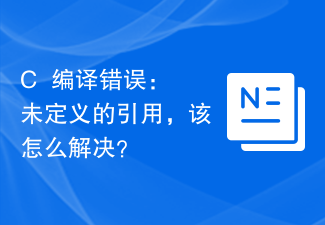
C++是一门广受欢迎的编程语言,但是在使用过程中,经常会出现“未定义的引用”这个编译错误,给程序的开发带来了诸多麻烦。本篇文章将从出错原因和解决方法两个方面,探讨“未定义的引用”错误的解决方法。一、出错原因C++编译器在编译一个源文件时,会将它分为两个阶段:编译阶段和链接阶段。编译阶段将源文件中的源码转换为汇编代码,而链接阶段将不同的源文件合并为一个可执行文
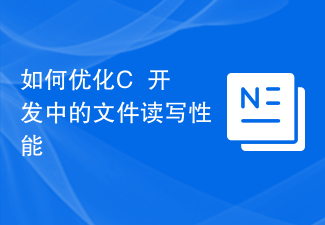
如何优化C++开发中的文件读写性能在C++开发过程中,文件的读写操作是常见的任务之一。然而,由于文件读写是磁盘IO操作,相对于内存IO操作来说会更为耗时。为了提高程序的性能,我们需要优化文件读写操作。本文将介绍一些常见的优化技巧和建议,帮助开发者在C++文件读写过程中提高性能。使用合适的文件读写方式在C++中,文件读写可以通过多种方式实现,如C风格的文件IO
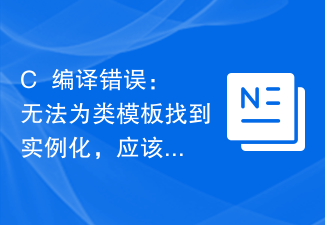
C++是一门强大的编程语言,它支持使用类模板来实现代码的复用,提高开发效率。但是在使用类模板时,可能会遭遇编译错误,其中一个比较常见的错误是“无法为类模板找到实例化”(error:cannotfindinstantiationofclasstemplate)。本文将介绍这个问题的原因以及如何解决。问题描述在使用类模板时,有时会遇到以下错误信息:e
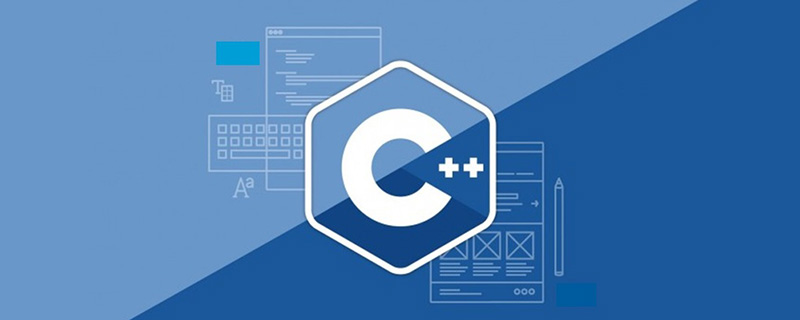
iostream头文件包含了操作输入输出流的方法,比如读取一个文件,以流的方式读取;其作用是:让初学者有一个方便的命令行输入输出试验环境。iostream的设计初衷是提供一个可扩展的类型安全的IO机制。
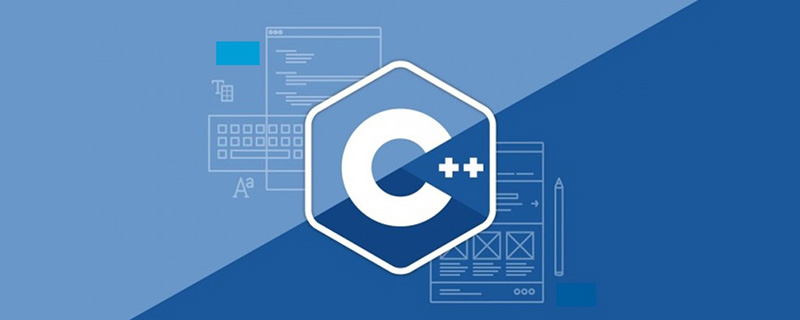
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。
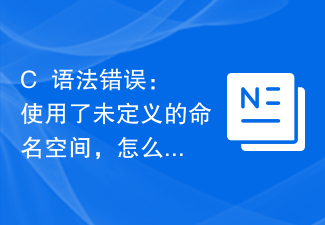
C++是一种广泛使用的高级编程语言,它有很高的灵活性和可扩展性,但同时也需要开发者严格掌握其语法规则才能避免出现错误。其中,常见的错误之一就是“使用了未定义的命名空间”。本文将介绍该错误的含义、出现原因和解决方法。一、什么是使用了未定义的命名空间?在C++中,命名空间是一种组织可重用代码的方式,以便保持代码的模块性和可读性。使用命名空间的方式可以使同名的函数


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
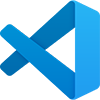
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
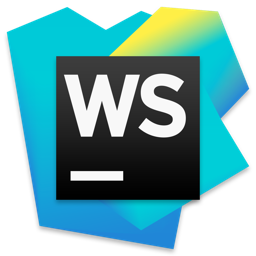
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Atom editor mac version download
The most popular open source editor