Effective use of STL exception handling: Use try blocks in blocks of code that may throw exceptions. Use a catch block to handle specific exception types, or a catch(...) block to handle all exceptions. Custom exceptions can be derived to provide more specific error information. In practical applications, STL's exception handling can be used to handle situations such as file read errors. Follow best practices, handle exceptions only when necessary, and keep exception handling code simple.
#How to effectively handle exceptions in C++ using STL?
Exception handling is crucial for handling runtime errors and restoring the execution flow. The C++ Standard Library (STL) provides a rich exception handling mechanism to enable developers to handle exceptions effectively.
Basic usage of exceptions
To handle exceptions, you need to perform the following steps:
- In the code block that may cause an exception, place The code is placed in
try
blocks. - Use
catch
blocks to handle specific exception types. - If the exception type is unknown, you can use the
catch(...)
block to handle all exceptions.
Example: Divide by zero
try { int x = 0; int y = 5; int result = y / x; // 引发异常 } catch (const std::runtime_error& e) { std::cerr << "运行时错误:" << e.what() << "\n"; }
Custom exception
You can use std:: exception
class derives custom exceptions.
class MyException : public std::exception { public: explicit MyException(const char* message) : std::exception(message) {} };
Exception handling practical cases
In the following cases, STL's exception handling is used to handle file read errors:
try { std::ifstream file("data.txt"); if (!file.is_open()) { throw std::runtime_error("无法打开文件"); } // ... 其他文件操作 ... } catch (const std::runtime_error& e) { std::cerr << "文件错误:" << e.what() << "\n"; }
Best Practice
- Handle exceptions only when really needed.
- Keep exception handling code simple.
- Use specific exception types instead of generic
catch()
blocks. - Don't throw an exception in the destructor.
The above is the detailed content of How to handle exceptions efficiently in C++ using STL?. For more information, please follow other related articles on the PHP Chinese website!
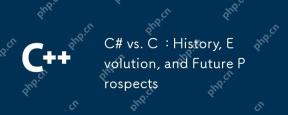
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
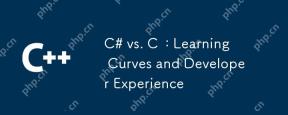
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
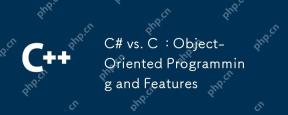
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
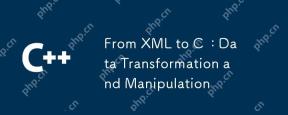
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
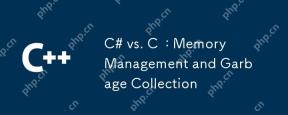
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
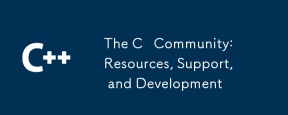
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
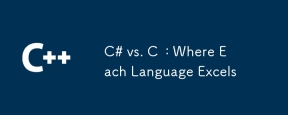
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 English version
Recommended: Win version, supports code prompts!
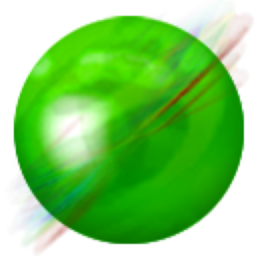
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool