C++ Lambda Expressions: Powerful Tools for Specific Scenarios
Introduction
Lambda An expression is an anonymous function introduced in C++ that allows you to create short, inline function objects. They're great for handling simple tasks that don't require declarations or separate naming.
Lambda syntax
Lambda expressions adopt the following syntax:
[capture-list](parameters) -> return-type { body }
- capture-list: Specify to capture Variables.
- parameters: Specify the parameters of the function.
- return-type: Specifies the return type of the function.
- body: Define the behavior of the function.
Uses
Lambda expressions are particularly useful in the following scenarios:
- Function object callbacks:Passed to other functions or objects as callback functions.
-
STL algorithms: Passed as arguments to standard library algorithms, such as
std::sort
andstd::find
. - Event handling: Respond to user input or system events (for example, in a GUI framework).
- Closures: Capture variables to create closures that persist in the scope outside the function.
Practical case
1. As a callback function
The following code uses lambda expressions to convert strings For uppercase:
#include <iostream> #include <string> using namespace std; int main() { string str = "hello"; transform(str.begin(), str.end(), str.begin(), [](char c) { return toupper(c); }); cout << str << endl; // 输出:HELLO return 0; }
2. As STL algorithm parameter
The following code uses lambda expression to find the first number greater than 5:
#include <iostream> #include <vector> using namespace std; int main() { vector<int> nums = {1, 3, 5, 7, 9}; auto it = find_if(nums.begin(), nums.end(), [](int n) { return n > 5; }); if (it != nums.end()) { cout << "找到了第一个大于 5 的数字:" << *it << endl; // 输出:7 } else { cout << "没有找到大于 5 的数字" << endl; } return 0; }
3. As a closure
The following code demonstrates how to create a closure using a lambda expression:
#include <iostream> using namespace std; int main() { int x = 10; auto f = [x](int y) { return x + y; }; cout << f(5) << endl; // 输出:15 return 0; }
Note: Compare to named functions , Lambda expressions have the following limitations:
- They do not have independent namespaces.
- They cannot be overloaded.
- They cannot have default parameters.
When using lambda expressions, carefully weigh their advantages and limitations to determine whether they are the best choice for a specific scenario.
The above is the detailed content of In what scenarios are C++ lambda expressions particularly useful?. For more information, please follow other related articles on the PHP Chinese website!
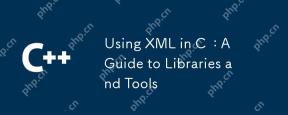
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
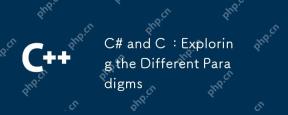
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
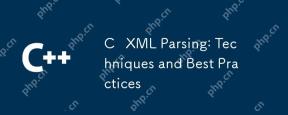
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
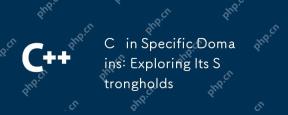
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
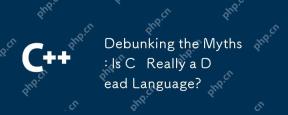
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
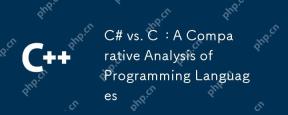
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
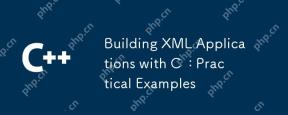
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
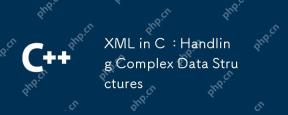
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
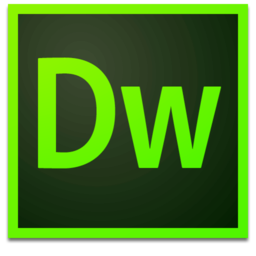
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
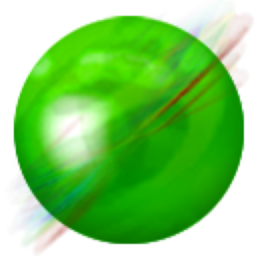
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
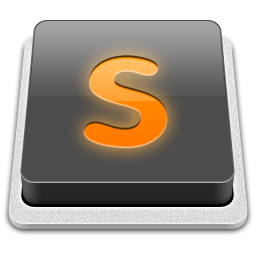
SublimeText3 Mac version
God-level code editing software (SublimeText3)
