How does C++ exception handling support custom error handling routines?
C++ Exception handling allows the creation of custom error handling routines to handle runtime errors by throwing exceptions and catching them using try-catch blocks. 1. Create a custom exception class derived from the exception class and override the what() method; 2. Use the throw keyword to throw an exception; 3. Use the try-catch block to catch exceptions and specify the exception types that can be handled.
C++ Exception Handling: Support for custom error handling routines
In C++, exception handling is a process that handles runtime A powerful mechanism for errors. It allows you to create custom error handling routines to handle error situations in an elegant and efficient manner.
Exception class
In C++, exceptions are represented by the exception
class or its derived classes. To throw a custom exception, create your own derived class and override the what()
method. This method returns a string describing the error.
class MyCustomException : public std::exception { public: const char* what() const noexcept override { return "This is my custom exception."; } };
Throw an exception
Use the throw
keyword to throw an exception. It accepts an exception object as parameter:
throw MyCustomException();
Catch the exception
Catch the exception using the try-catch
block. Each catch
clause specifies an exception type that can be handled. If an exception of matching type occurs, the code in this clause will be executed:
try { // 可能抛出异常的代码 } catch (MyCustomException& e) { // 处理 MyCustomException 异常 } catch (std::exception& e) { // 处理所有其他类型的异常 }
Practical Case
Let us consider a file that is opened and read from it function. If the file cannot be opened, the function should throw our custom exception:
#include <fstream> #include <iostream> using namespace std; // 自定义异常类 class FileOpenException : public std::exception { public: const char* what() const noexcept override { return "Could not open the file."; } }; // 打开文件并读取其内容的函数 string read_file(const string& filename) { ifstream file(filename); if (!file.is_open()) { throw FileOpenException(); } string contents; string line; while (getline(file, line)) { contents += line + '\n'; } file.close(); return contents; } int main() { try { string contents = read_file("file.txt"); cout << contents << endl; } catch (FileOpenException& e) { cout << "Error: " << e.what() << endl; } catch (std::exception& e) { cout << "An unexpected error occurred." << endl; } return 0; }
In the above example, the read_file()
function throws the FileOpenException
exception, Launched when a file cannot be opened. In main()
function we use try-catch
block to catch exceptions and handle them accordingly.
The above is the detailed content of How does C++ exception handling support custom error handling routines?. For more information, please follow other related articles on the PHP Chinese website!

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
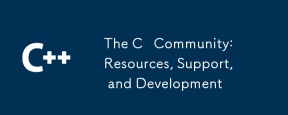
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
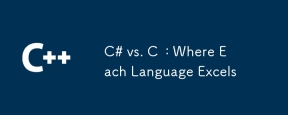
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
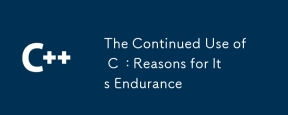
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
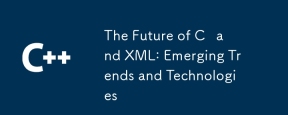
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
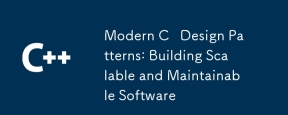
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
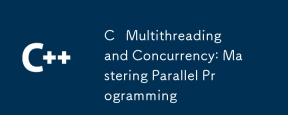
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
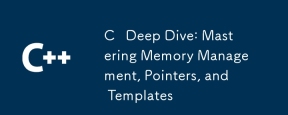
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
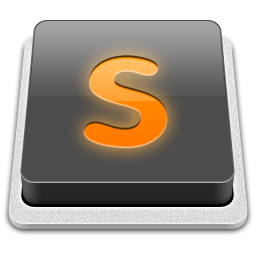
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
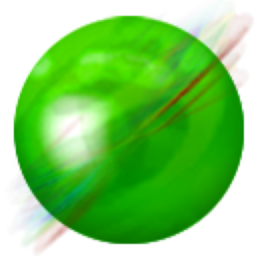
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment