Performance optimization tips for Go concurrent programming include: using a Goroutine pool to avoid the overhead of creating and destroying goroutines. Use channels to pass data instead of shared memory to prevent data races. Avoid using heavy locks and consider using lock-free data structures to reduce contention. Execute tasks in parallel and take advantage of Go's concurrency features.
Performance Optimization Tips in Go Concurrent Programming
In Go, concurrent programming is widely used to improve application performance. However, when implementing concurrency, you need to pay attention to some key performance optimization tips to avoid unnecessary performance overhead.
1. Using Goroutine Pool
There may be overhead when creating a new goroutine. By using a goroutine pool, you avoid the cost of repeatedly creating and destroying goroutines.
package main import "sync" var wg sync.WaitGroup var pool = sync.Pool{ New: func() interface{} { return new(func() {}) }, } func main() { for i := 0; i < 10000; i++ { fn := pool.Get().(func()) go fn() wg.Add(1) go func() { defer wg.Done() pool.Put(fn) }() } wg.Wait() }
2. Use channels to pass data instead of shared memory
Sharing memory between goroutines may cause data races and unpredictable behavior. Instead, passing data using channels is safer and more scalable.
package main import ( "fmt" "sync" "time" ) var wg sync.WaitGroup var ch = make(chan int) func main() { for i := 0; i < 10000; i++ { go func(i int) { defer wg.Done() ch <- i }(i) } for i := 0; i < 10000; i++ { fmt.Println(<-ch) } close(ch) wg.Wait() }
3. Avoid using heavy locks
Locks are crucial in concurrent programming, but overuse can lead to performance degradation. Consider using lock-free data structures (such as atomic values or lock-free queues) to reduce contention.
package main import ( "sync/atomic" "unsafe" ) var ( count int32 ptr unsafe.Pointer ) func main() { for i := 0; i < 10000; i++ { atomic.AddInt32(&count, 1) atomic.StorePointer(&ptr, nil) } }
4. Execute tasks in parallel
Take full advantage of the concurrency features of Go by using goroutine
to execute tasks in parallel instead of serial execution.
package main import ( "fmt" "sync" ) func main() { var wg sync.WaitGroup wg.Add(3) go func() { for i := 0; i < 10000; i++ { fmt.Println(i) } wg.Done() }() go func() { for i := 10000; i < 20000; i++ { fmt.Println(i) } wg.Done() }() go func() { for i := 20000; i < 30000; i++ { fmt.Println(i) } wg.Done() }() wg.Wait() }
The above is the detailed content of Performance optimization techniques in Go concurrent programming. For more information, please follow other related articles on the PHP Chinese website!
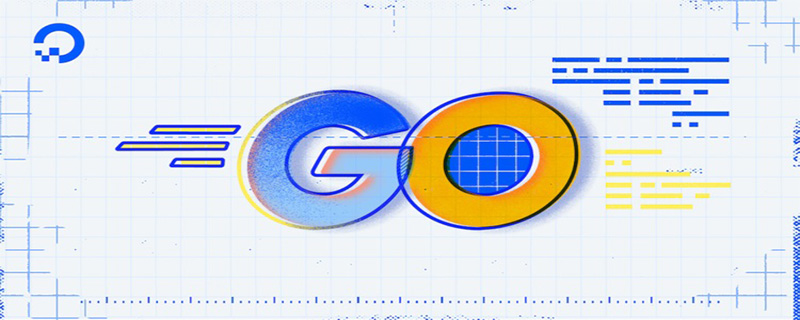
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
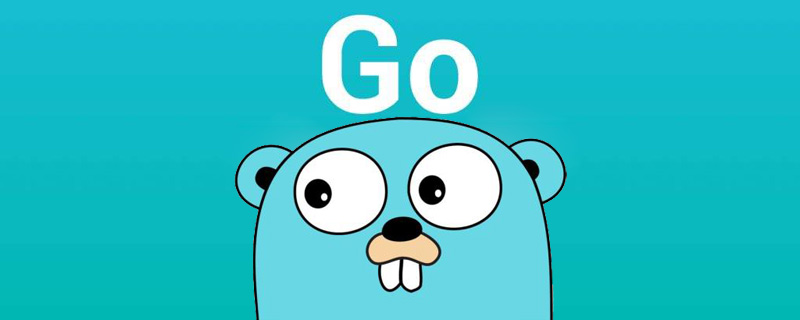
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
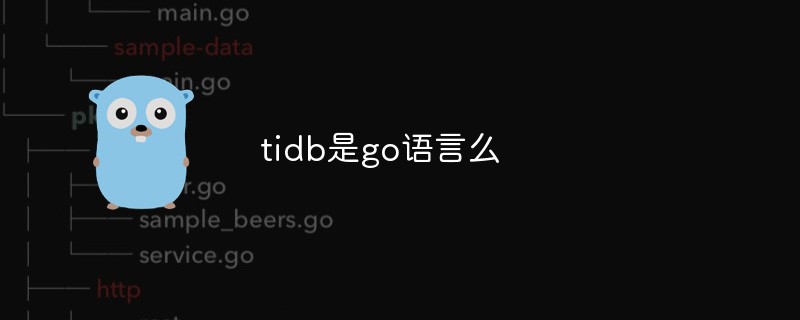
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
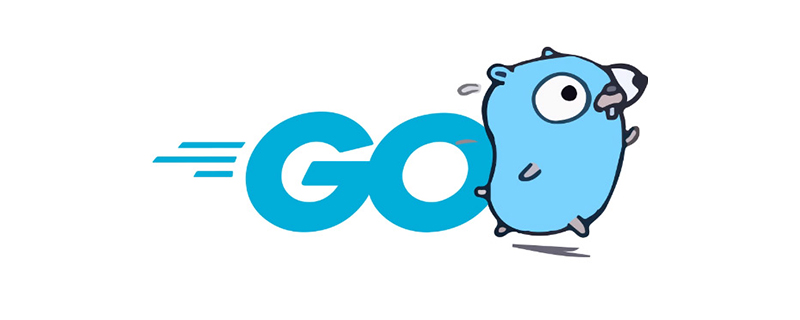
go语言能编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言。对Go语言程序进行编译的命令有两种:1、“go build”命令,可以将Go语言程序代码编译成二进制的可执行文件,但该二进制文件需要手动运行;2、“go run”命令,会在编译后直接运行Go语言程序,编译过程中会产生一个临时文件,但不会生成可执行文件。
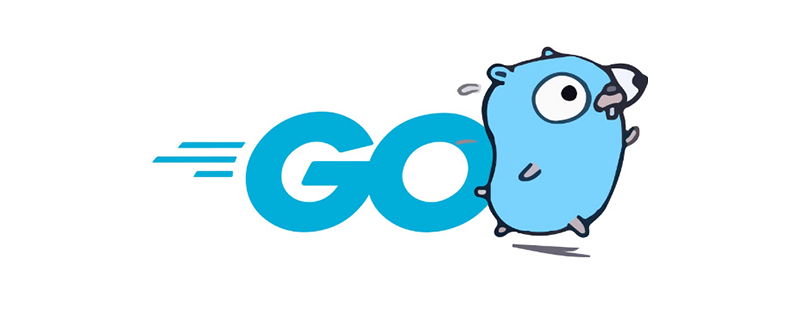
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
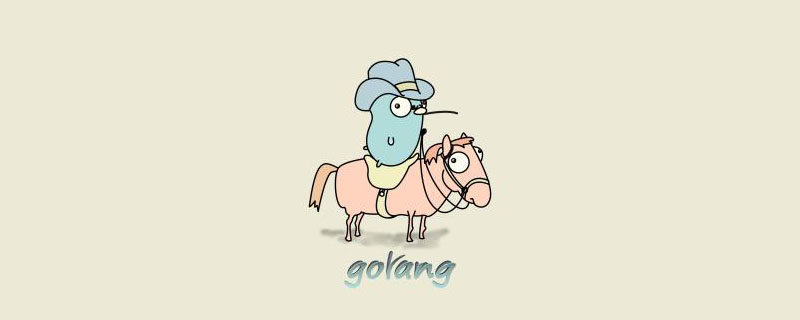
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
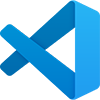
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
