How do smart pointers simplify memory management in C++?
Smart pointers simplify C++ memory management and provide two types: std::unique_ptr: a pointer to a unique object, which automatically destroys the object when it goes out of scope. std::shared_ptr: Pointer to a shared object, the object will be destroyed only when all pointers go out of scope. By using smart pointers, the pointed objects can be automatically released, avoiding the complexity and errors caused by manual memory management.
Smart pointers: The simple way to manage memory in C++
Managing memory in C++ can be complex and error-prone Task. Smart pointers are lightweight objects that simplify this process by managing memory behind the scenes.
Smart pointer type
-
std::unique_ptr
: Pointer to a unique object. When the pointer goes out of scope, the object is automatically destroyed. -
std::shared_ptr
: Pointer to a shared object, the object will only be destroyed when all pointers go out of scope.
Usage
The smart pointer type is similar to a regular pointer, but does not require manual release:
auto p = std::make_unique<MyObject>(); // 创建唯一指针 std::vector<std::shared_ptr<MyObject>> pointers; // 创建共享指针集合
When the pointer goes out of scope , the pointed object will be automatically destroyed:
{ std::unique_ptr<MyObject> p = std::make_unique<MyObject>(); // ... 使用 p ... } // p 指出对象将在此处被销毁
Practical case
Consider a function that returns a pointer to an object:
MyObject* createObject() { return new MyObject(); // 返回裸指针 }
Using a smart pointer, the function can return a pointer that automatically manages the memory:
std::unique_ptr<MyObject> createObject() { return std::make_unique<MyObject>(); // 返回智能指针 }
This ensures that the object is deleted when the pointer goes out of scope, eliminating the need for manual memory management.
The above is the detailed content of How do smart pointers simplify memory management in C++?. For more information, please follow other related articles on the PHP Chinese website!
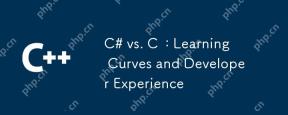
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
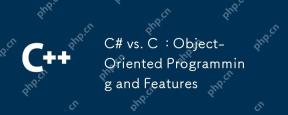
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
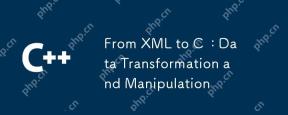
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
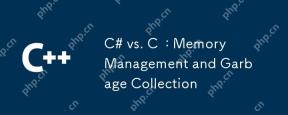
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
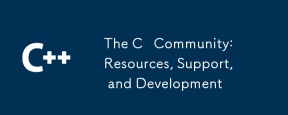
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
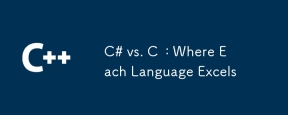
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
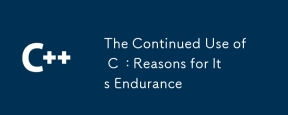
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
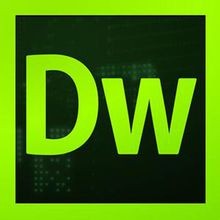
Dreamweaver CS6
Visual web development tools
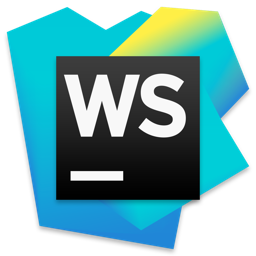
WebStorm Mac version
Useful JavaScript development tools
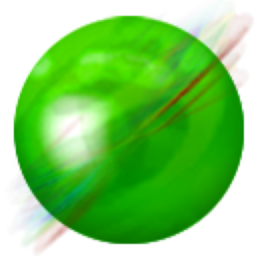
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor