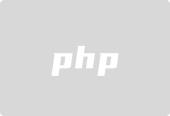
Comparison and selection of thread synchronization mechanisms in C++ concurrent programming
Introduction
In multi-threaded programming, thread synchronization is crucial to prevent data races and ensure thread safety. C++ provides several thread synchronization mechanisms, each with its own advantages and disadvantages. This article will compare these mechanisms and guide readers in choosing the one best suited for their specific application.
Thread synchronization mechanism
Mutex (mutex, mutually exclusive object)
- Provides access to critical sections mutually exclusive access.
- Advantages: Simple and easy to use, high efficiency.
- Disadvantages: prone to deadlock, because once the thread acquires the mutex, it will hold it until it is released.
Conditional variable (conditional variable)
- Used with a mutex to allow threads to wait when specific conditions are met.
- Advantages: Deadlock can be avoided because the thread will release the mutex when the condition is not met.
- Disadvantages: More complex than mutex and slightly less efficient.
Semaphore
- Control access to shared resources.
- Advantages: You can control the availability of resources and prevent threads from accessing shared resources too much.
- Disadvantages: More complex and less efficient than mutexes and condition variables.
Read-write lock (read-write lock)
- is specially designed for scenarios that support both read and write access.
- Advantages: Allow multiple threads to read shared data at the same time, while only allowing one thread to write data.
- Disadvantages: More complex than mutexes and condition variables, slightly less efficient.
Atomic operations
- Provide atomic access to a single variable or memory location.
- Advantages: High efficiency, no need for any other synchronization mechanism.
- Disadvantages: Only suitable for simple scenarios and does not support complex synchronization requirements.
Selection criteria
When selecting an appropriate synchronization mechanism, the following factors should be considered:
-
Critical section Complexity: More complex critical sections require more complex synchronization mechanisms.
-
Possibility of deadlock: If deadlock is a problem, you need to use mechanisms that can avoid deadlock (such as condition variables).
-
Concurrency level: If your application involves a large number of threads, you will need to use a more scalable mechanism (such as read-write locks or semaphores).
-
Efficiency: Consider the overhead of the mechanism and its impact on application performance.
Practical case
Mutex:
std::mutex m;
void myFunction() {
std::lock_guard<std::mutex> lock(m);
// 临界区代码
}
Condition variable:
std::mutex m;
std::condition_variable cv;
bool ready = false;
void wait() {
std::unique_lock<std::mutex> lock(m);
cv.wait(lock, []{ return ready; });
}
void notify() {
std::lock_guard<std::mutex> lock(m);
ready = true;
cv.notify_all();
}
Semaphore:
std::counting_semaphore<int> semaphore(5);
void myFunction() {
semaphore.acquire();
// 临界区代码
semaphore.release();
}
Read-write lock:
std::shared_timed_mutex m;
void read() {
std::shared_lock<std::shared_timed_mutex> lock(m);
// 读操作
}
void write() {
std::unique_lock<std::shared_timed_mutex> lock(m);
// 写操作
}
The above is the detailed content of Comparison and selection of thread synchronization mechanisms in C++ concurrent programming?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn