


C++ cross-compilation and memory optimization to efficiently build cross-platform applications
C++ cross-compilation allows code to be compiled on heterogeneous platforms. Memory optimization includes using smart pointers, optimizing data structures, and reducing dynamic allocation. Practical use cases demonstrate cross-platform Fibonacci number calculations, cross-compilation managed through CMake, and memory optimization using smart pointers and optimization algorithms.
C++ cross-compilation and memory optimization: creating efficient cross-platform applications
Introduction
Cross-platform development With its increasing popularity, C++ has become an ideal choice for building cross-platform applications due to its strong performance and portability. This article will explore C++ cross-compilation and memory optimization techniques to help developers build efficient, portable cross-platform applications.
Cross-compilation
Cross-compilation allows developers to compile code on different platforms for the target platform. For example, compile as a Linux application on macOS. To cross-compile, you need a cross-compiler, which supports different architectures and toolchains. The cross-compiler can be specified by setting environment variables or using a compilation management tool such as CMake.
Memory Optimization
Optimizing memory can significantly improve the performance and reliability of your application. C++ provides powerful memory management tools such as pointers and references, as well as smart pointers in the Standard Template Library (STL) for efficient memory management. Other memory optimization techniques include:
- Reduce unnecessary dynamic memory allocation
- Use memory pools and object pools
- Optimize data structures and algorithms to reduce memory usage
Practical Case
To illustrate cross-compilation and memory optimization, let us write a simple C++ application that runs on Linux and Windows platforms and calculates Bonacci Sequence.
//Fibonacci.cpp #include <iostream> using namespace std; int fib(int n) { if (n <= 1) return n; return fib(n-1) + fib(n-2); } int main() { int n; cout << "Enter a number to calculate its Fibonacci number: "; cin >> n; cout << "Fibonacci number of " << n << " is: " << fib(n) << endl; return 0; }
Cross-compilation
- Use
CMake
as the cross-compilation management tool. - Specify the cross-compiler and target platform in the CMakeLists.txt file.
set(CMAKE_CROSSCOMPILING ON) set(CMAKE_TOOLCHAIN_FILE "path/to/cross-compiler/toolchain.cmake") set(CMAKE_SYSTEM_NAME "Linux")
Memory optimization
- Use smart pointers to manage dynamically allocated memory to prevent memory leaks and wild pointers.
- Optimization
fib
The function uses recursion to reduce unnecessary memory allocation. - Use
std::vector
instead of a native array to take advantage of its automatic memory management and sizing capabilities.
#include <memory> #include <vector> std::vector<int> fib_cache(2, 0); // 备忘录优化 int fib(int n) { if (n <= 1) return n; auto& result = fib_cache[n]; if (!result) // 未计算过 result = fib(n-1) + fib(n-2); return result; } int main() { int n; cout << "Enter a number to calculate its Fibonacci number: "; cin >> n; cout << "Fibonacci number of " << n << " is: " << fib(n) << endl; return 0; }
The above is the detailed content of C++ cross-compilation and memory optimization to efficiently build cross-platform applications. For more information, please follow other related articles on the PHP Chinese website!
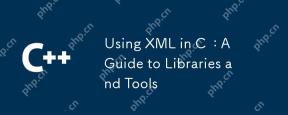
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
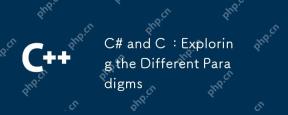
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
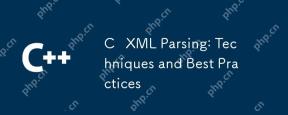
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
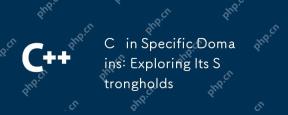
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
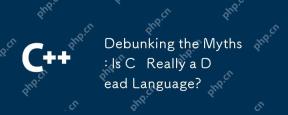
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
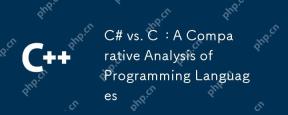
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
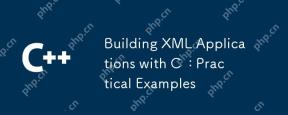
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
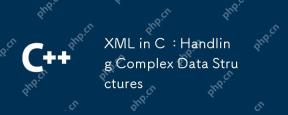
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
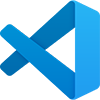
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
