Memory pool is a technology that optimizes memory allocation by pre-allocating memory blocks to reduce allocation and release overhead. In C++, memory pools can be implemented using std::pmr::memory_resource and std::pmr::polymorphic_allocator classes, such as the MemoryPool class, which does this by specifying the memory pool size through a constructor and reducing the available memory when allocating memory. Memory pool function. In addition, the practical case shows an example of using the memory pool to optimize integer allocation. By using the memory pool to allocate 1000 integers, the performance of memory allocation and deallocation can be improved.
Memory pool in C++
Introduction
The memory pool is a kind of management tool Memory allocation optimization techniques. It can pre-allocate a batch of memory and divide it into smaller chunks so that these chunks can be allocated and freed quickly. This can greatly reduce the overhead of memory allocation and deallocation, thereby improving application performance.
Implementation
In C++, you can use the std::pmr::memory_resource
and std::pmr::polymorphic_allocator
classes To implement the memory pool.
#include <memory_resource> class MemoryPool : public std::pmr::memory_resource { public: // 构造函数,指定内存池的大小 MemoryPool(size_t size) : _data(new char[size]), _available(size) {} // 分配内存 void* do_allocate(size_t size, size_t alignment) override { if (_available >= size) { void* ptr = _data + (_size - _available); _available -= size; return ptr; } return nullptr; } // 释放内存 void do_deallocate(void* ptr, size_t size, size_t alignment) override { _available += size; } private: char* _data; // 内存池数据 size_t _size; // 内存池大小 size_t _available; // 可用内存大小 };
Practical case
The following is an example of using the memory pool to optimize memory allocation:
#include "MemoryPool.h" int main() { // 创建一个 1 MB 大小的内存池 std::pmr::polymorphic_allocator<int> allocator(new MemoryPool(1024 * 1024)); // 使用内存池分配 1000 个整数 int* arr = allocator.allocate(1000); // 使用完整数后释放内存 allocator.deallocate(arr, 1000); return 0; }
In this example, using the memory pool to allocate 1000 integers can Significantly improves the performance of memory allocation and deallocation.
The above is the detailed content of How to implement memory pool in C++?. For more information, please follow other related articles on the PHP Chinese website!
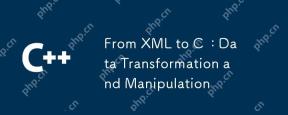
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
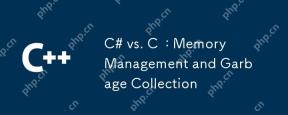
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
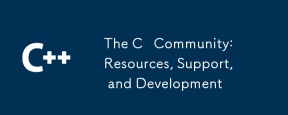
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
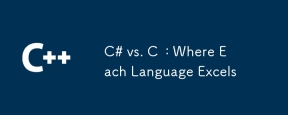
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
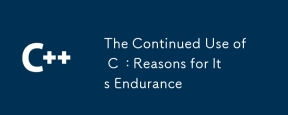
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
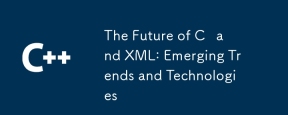
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
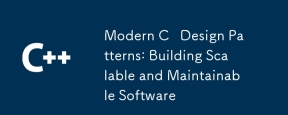
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
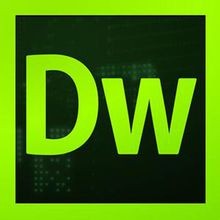
Dreamweaver CS6
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
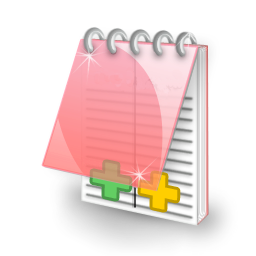
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.