The template method pattern defines the skeleton of the algorithm, and the specific steps are implemented by subclasses, allowing subclasses to customize specific steps without changing the overall structure. This pattern is used to: 1. Define the skeleton of the algorithm. 2. Defer the specific behavior of the algorithm to subclasses. 3. Allow subclasses to customize certain steps of the algorithm without changing the overall structure of the algorithm.
Template Method Pattern in PHP
Introduction
The Template Method Pattern is a design pattern that The skeleton of the algorithm is defined, and the specific steps are implemented by subclasses. This allows subclasses to customize specific steps without changing the overall structure of the algorithm.
UML Diagram
+----------------+ | AbstractClass | +----------------+ | + templateMethod() | +----------------+ +----------------+ | ConcreteClass1 | +----------------+ | + concreteMethod1() | +----------------+ +----------------+ | ConcreteClass2 | +----------------+ | + concreteMethod2() | +----------------+
Code Example
AbstractClass.php
abstract class AbstractClass { public function templateMethod() { $this->step1(); $this->step2(); $this->hookMethod(); } protected abstract function step1(); protected abstract function step2(); protected function hookMethod() {} }
ConcreteClass1.php
class ConcreteClass1 extends AbstractClass { protected function step1() { echo "ConcreteClass1: Step 1<br>"; } protected function step2() { echo "ConcreteClass1: Step 2<br>"; } }
ConcreteClass2.php
class ConcreteClass2 extends AbstractClass { protected function step1() { echo "ConcreteClass2: Step 1<br>"; } protected function step2() { echo "ConcreteClass2: Step 2<br>"; } protected function hookMethod() { echo "ConcreteClass2: Hook Method<br>"; } }
Practical case
Assume we have For a student management system, we need to create two pages: the "Student List" page and the "Student Details" page. The two pages use the same layout, but have different content.
StudentManager.php
class StudentManager { public function showStudentList() { $students = // 获取学生数据 $view = new StudentListView(); $view->setStudents($students); $view->render(); } public function showStudentDetail($id) { $student = // 获取学生数据 $view = new StudentDetailView(); $view->setStudent($student); $view->render(); } }
StudentListView.php
class StudentListView extends AbstractView { private $students; public function setStudents($students) { $this->students = $students; } public function render() { $this->showHeader(); $this->showStudents(); $this->showFooter(); } protected function showStudents() { echo "<h1 id="学生列表">学生列表</h1>"; echo "<ul>"; foreach ($this->students as $student) { echo "<li>" . $student->getName() . "</li>"; } echo "</ul>"; } }
StudentDetailView.php
class StudentDetailView extends AbstractView { private $student; public function setStudent($student) { $this->student = $student; } public function render() { $this->showHeader(); $this->showStudent(); $this->showFooter(); } protected function showStudent() { echo "<h1 id="学生详情">学生详情</h1>"; echo "<p>姓名:" . $this->student->getName() . "</p>"; echo "<p>年龄:" . $this->student->getAge() . "</p>"; } }
The above is the detailed content of How to use template method pattern in PHP?. For more information, please follow other related articles on the PHP Chinese website!
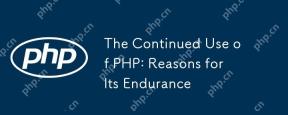
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
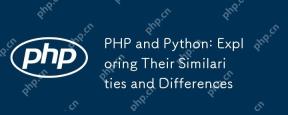
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
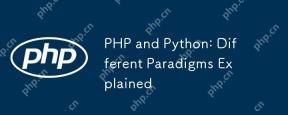
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
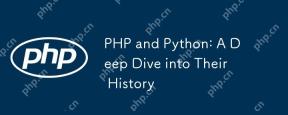
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
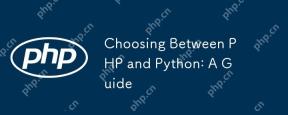
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
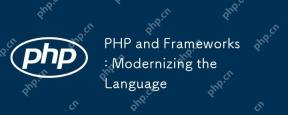
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
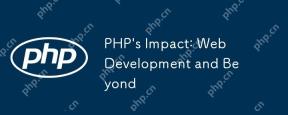
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
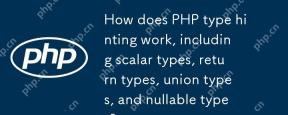
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
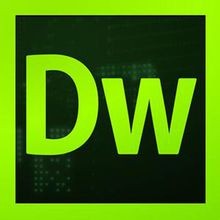
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
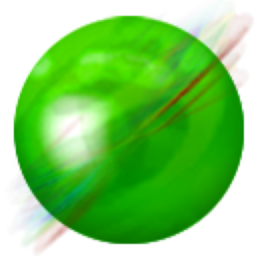
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment