


Custom exception classes in PHP allow developers to create application-specific exception types, adding additional information and handling logic. By inheriting from the Exception class, a custom exception class can contain properties (such as error codes) and methods (such as getting error details). This enhances PHP's exception handling mechanism, provides a more flexible and customizable exception handling method, and improves the robustness, readability, and maintainability of applications.
PHP Exception Handling: Detailed Explanation of Custom Exception Classes
Exception handling is an important and commonly used mechanism in PHP, which can help developers better Handle errors gracefully and provide meaningful feedback. Custom exception classes are a powerful way to extend this mechanism, allowing us to create application-specific exception types and add additional information and handling logic.
Creation of custom exception classes
Creating a custom exception class is similar to creating a regular class, but it needs to inherit from the Exception
class:
class MyCustomException extends Exception { // 在这里定义额外的属性和方法 }
Add Properties and Methods
Custom exception classes can contain additional properties and methods to provide specific information about the exception or to perform specific processing logic. For example, we can add an errorCode
attribute to identify the type of exception:
class MyCustomException extends Exception { private $errorCode; public function __construct($message, $errorCode) { parent::__construct($message); $this->errorCode = $errorCode; } public function getErrorCode() { return $this->errorCode; } }
Practical case: Validator exception
Suppose we have a validator class responsible for validating user input . We can create a custom exception class to handle validation errors:
class ValidationException extends Exception { private $errors; public function __construct(array $errors) { parent::__construct('Validation failed'); $this->errors = $errors; } public function getErrors() { return $this->errors; } }
In the validation logic, we can use this exception class to encapsulate the validation errors:
if (// 验证失败) { $errors = [// 验证错误列表]; throw new ValidationException($errors); }
In this way, we can Other parts of use $exception->getErrors()
to get details of validation errors.
Throwing and catching custom exceptions
Throwing and catching custom exceptions are the same as ordinary exceptions. We can throw an exception using the throw
keyword and catch it using the try...catch
block:
try { // 代码可能引发异常 } catch (MyCustomException $e) { // 处理自定义异常 }
In the catch block we can access the custom Exception properties and calling its methods to obtain more information about the exception and perform specific processing logic.
By creating a custom exception class, we can enhance PHP's exception handling mechanism and provide a more flexible and customizable exception handling method. This helps improve the robustness, readability, and maintainability of your application.
The above is the detailed content of PHP exception handling: detailed explanation of custom exception classes. For more information, please follow other related articles on the PHP Chinese website!
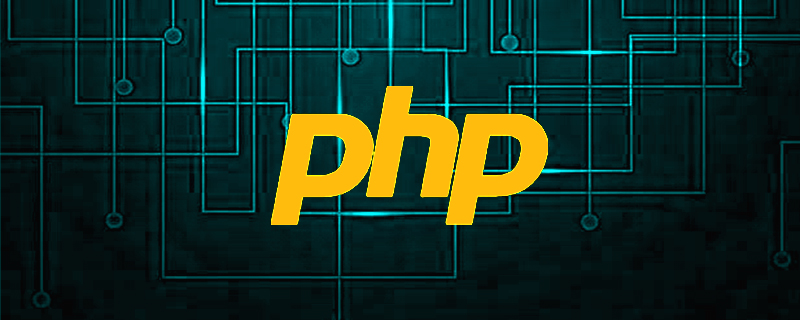
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
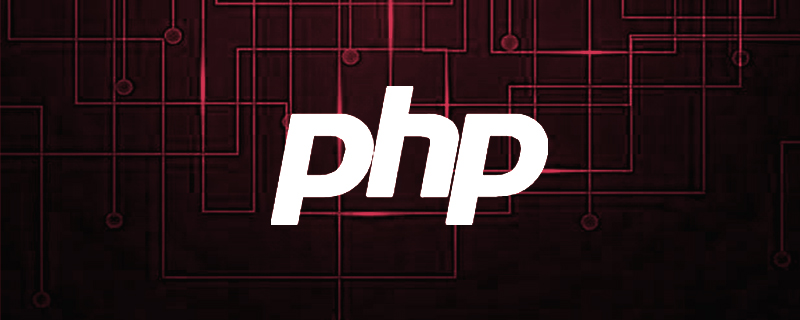
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
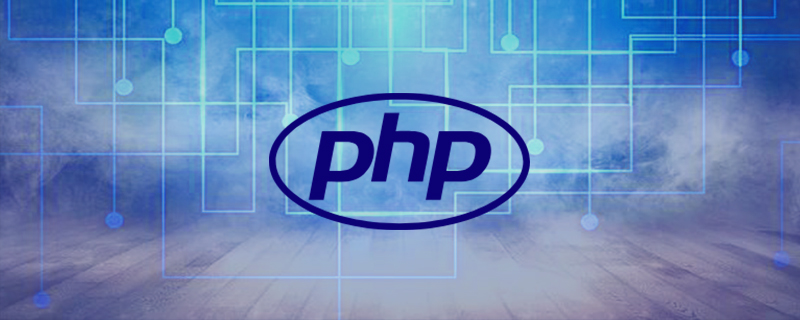
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
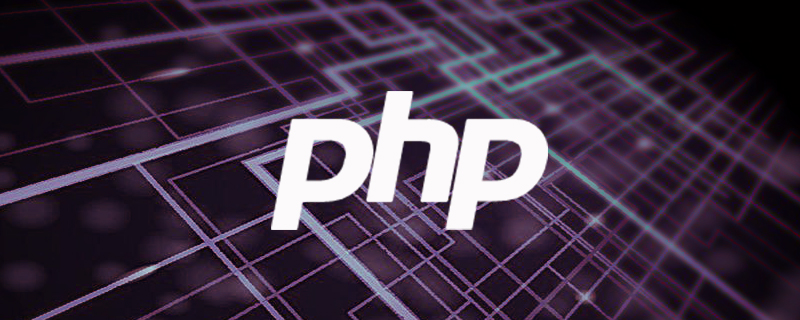
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
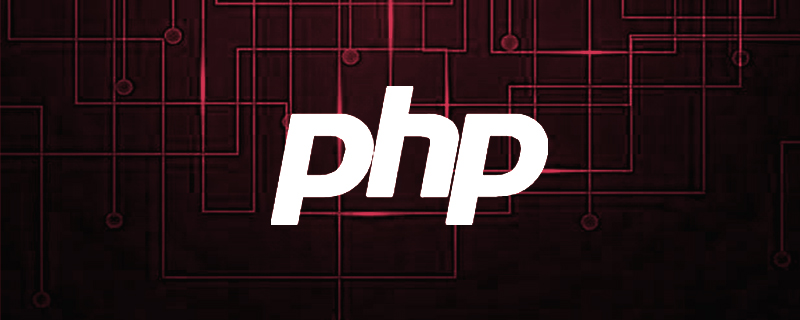
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
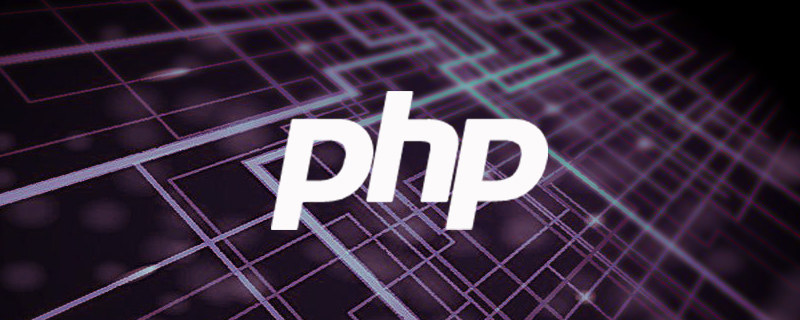
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
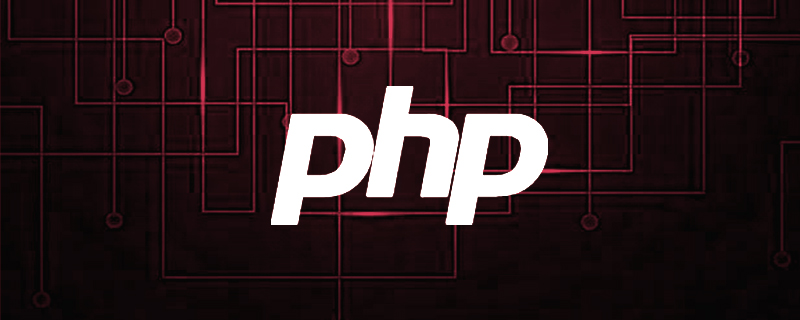
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
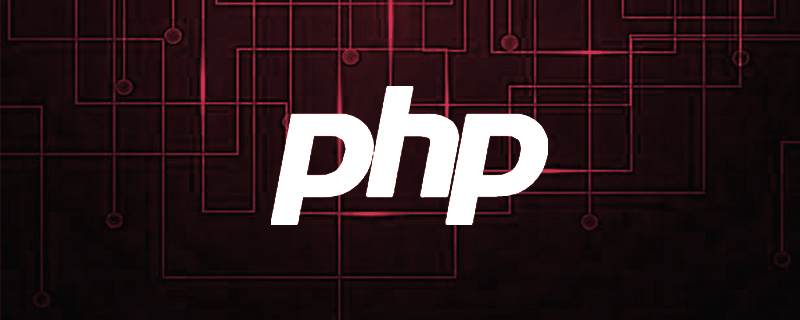
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
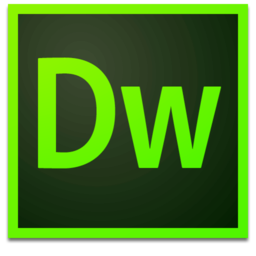
Dreamweaver Mac version
Visual web development tools
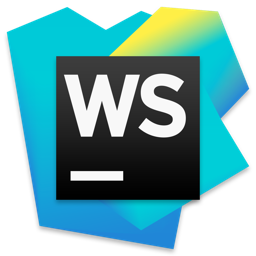
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
