


This article describes how to build a Golang RESTful API. First, build a RESTful API by importing the necessary libraries, defining the data model, and creating routes. Second, create and apply middleware for authentication using go-chi/chigot and go-chi/chi/middleware. The article further gives a practical case example to demonstrate the practical application of the solution.
How to build a Golang RESTful API and use middleware for authentication
1. Build a RESTful API
Import required Library:
import ( "encoding/json" "fmt" "log" "net/http" "github.com/gorilla/mux" )
Define data model:
type Product struct { ID int `json:"id"` Name string `json:"name"` Price float64 `json:"price"` }
Create route:
router := mux.NewRouter() router.HandleFunc("/products", GetProducts).Methods("GET") router.HandleFunc("/products/{id}", GetProduct).Methods("GET") router.HandleFunc("/products", CreateProduct).Methods("POST") router.HandleFunc("/products/{id}", UpdateProduct).Methods("PUT") router.HandleFunc("/products/{id}", DeleteProduct).Methods("DELETE")
Definition Controller:
func GetProducts(w http.ResponseWriter, r *http.Request) { // ... } func GetProduct(w http.ResponseWriter, r *http.Request) { // ... } func CreateProduct(w http.ResponseWriter, r *http.Request) { // ... } func UpdateProduct(w http.ResponseWriter, r *http.Request) { // ... } func DeleteProduct(w http.ResponseWriter, r *http.Request) { // ... }
2. Use middleware for authentication
Install middleware:
go get github.com/go-chi/chi go get github.com/go-chi/chi/middleware
Create middleware :
func AuthMiddleware(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { // ... 检查认证信息 ... if !authorized { http.Error(w, "Unauthorized", http.StatusUnauthorized) return } // ... 继续处理请求 ... next.ServeHTTP(w, r) }) }
Application middleware:
router.Use(AuthMiddleware)
Practical case
Example:
func main() { // 初始化数据库连接 db, err := sql.Open("sqlite3", "mydb.db") if err != nil { log.Fatal(err) } // 创建路由器 router := mux.NewRouter() // 定义路由并应用身份验证中间件 router.HandleFunc("/products", GetProducts).Methods("GET").Use(AuthMiddleware) router.HandleFunc("/products/{id}", GetProduct).Methods("GET").Use(AuthMiddleware) router.HandleFunc("/products", CreateProduct).Methods("POST").Use(AuthMiddleware) router.HandleFunc("/products/{id}", UpdateProduct).Methods("PUT").Use(AuthMiddleware) router.HandleFunc("/products/{id}", DeleteProduct).Methods("DELETE").Use(AuthMiddleware) // 启动服务器 http.Handle("/", router) log.Fatal(http.ListenAndServe(":8080", nil)) }
The above is the detailed content of How to build a Golang RESTful API and use middleware for authentication?. For more information, please follow other related articles on the PHP Chinese website!
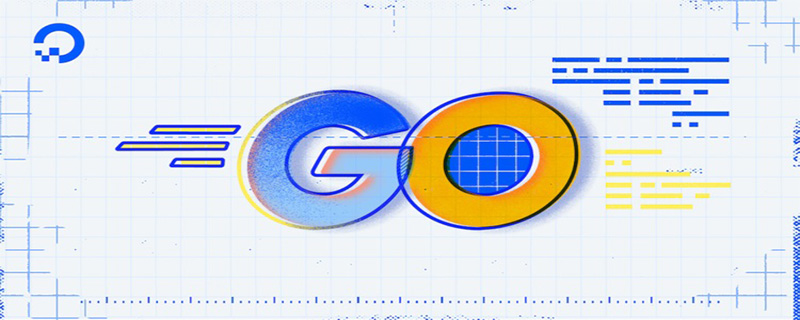
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
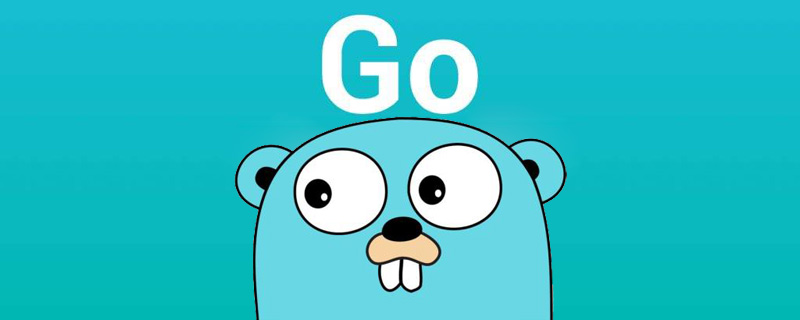
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
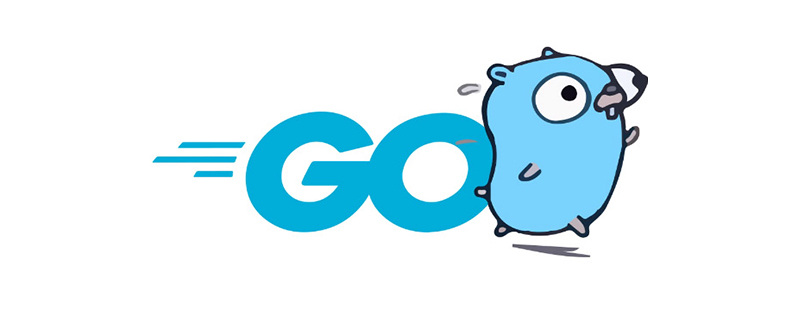
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
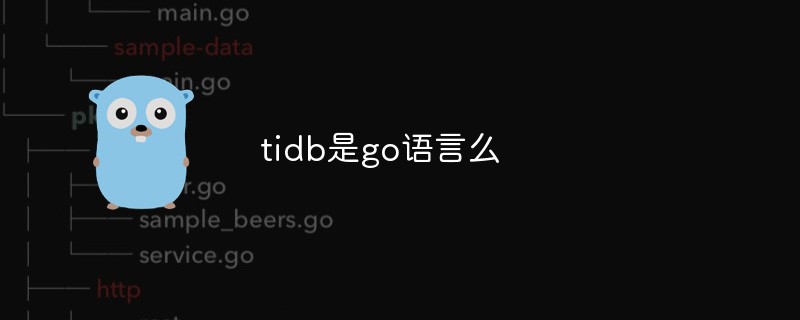
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
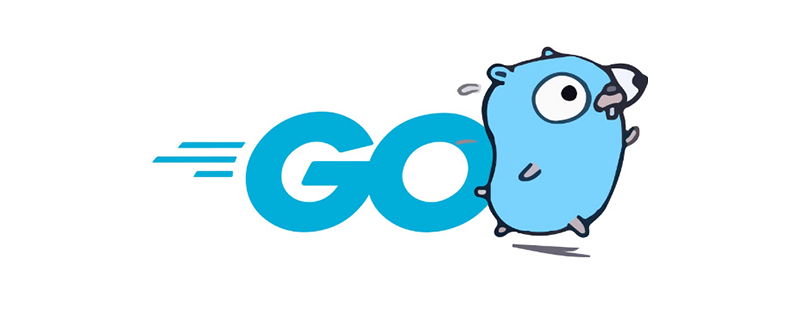
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
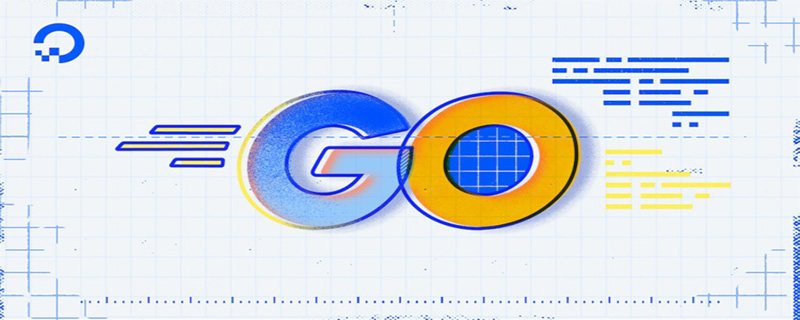
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
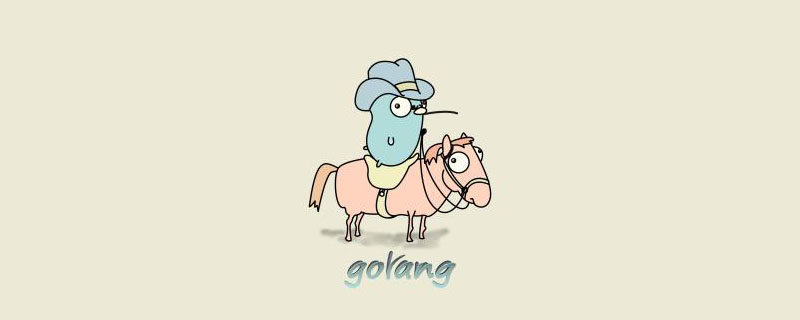
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
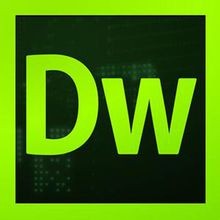
Dreamweaver CS6
Visual web development tools
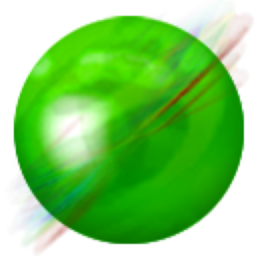
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
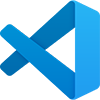
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
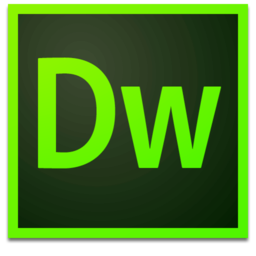
Dreamweaver Mac version
Visual web development tools
