


C++ Metaprogramming plays an important role in metadata management and dynamic property access: Metadata Management: Use templates and compile-time calculations to manage metadata for class properties, accessible at runtime. Dynamic property access: Use decltype to implement dynamic property access, allowing you to get and set an object's properties at runtime.
The role of C++ metaprogramming in metadata management and dynamic attribute access
Metaprogramming is an intermediate and advanced programming technology in C++. Allows a program to manipulate its own code and generate new code. It has powerful applications in metadata management and dynamic attribute access.
Metadata management
Metadata is data about data. In C++, templates and compile-time calculations can be used for metadata management. For example, we can define a structure to describe the properties of a class:
template<typename T> struct AttributeMetadata { std::string name; std::string type; bool is_required; };
We can then use metaprogramming techniques to generate metadata for a class with specific properties:
class MyClass { std::string name; int age; bool is_active; }; static const AttributeMetadata<MyClass> attributeMetadata[] = { {"name", "std::string", false}, {"age", "int", false}, {"is_active", "bool", false} };
Now, we can Access this metadata at runtime:
for (const auto& attribute : attributeMetadata) { std::cout << "Name: " << attribute.name << std::endl; std::cout << "Type: " << attribute.type << std::endl; std::cout << "Required: " << (attribute.is_required ? "Yes" : "No") << std::endl; }
Dynamic Property Access
Metaprogramming can also implement dynamic property access, allowing getting and setting an object's properties at runtime. We can use the decltype auto introduced in C++11, which allows us to infer the type of an expression:
class MyDynamicObject { public: template<typename T> T getAttribute(const std::string& name) { return decltype(this->*name)(); } template<typename T> void setAttribute(const std::string& name, const T& value) { (this->*name) = value; } };
Now, we can get and set properties dynamically like this:
MyDynamicObject obj; std::string name = obj.getAttribute<std::string>("name"); obj.setAttribute("age", 25);
Practical Case
In the following practical case, we use metaprogramming to manage log configuration:
template<typename T> struct LogLevel { static const char* value; }; struct Debug : LogLevel<Debug> { static const char* value = "DEBUG"; }; struct Info : LogLevel<Info> { static const char* value = "INFO"; }; struct Warning : LogLevel<Warning> { static const char* value = "WARNING"; }; struct Error : LogLevel<Error> { static const char* value = "ERROR"; }; class Logger { public: template<typename L> void log(const char* message) { std::cout << "[" << LogLevel<L>::value << "] " << message << std::endl; } };
Using metaprogramming, we can use different log levels to obtain logs:
int main() { Logger logger; logger.log<Debug>("This is a debug message"); logger.log<Info>("This is an info message"); logger.log<Warning>("This is a warning message"); logger.log<Error>("This is an error message"); return 0; }
Output:
[DEBUG] This is a debug message [INFO] This is an info message [WARNING] This is a warning message [ERROR] This is an error message
The above is the detailed content of The role of C++ metaprogramming in metadata management and dynamic property access?. For more information, please follow other related articles on the PHP Chinese website!
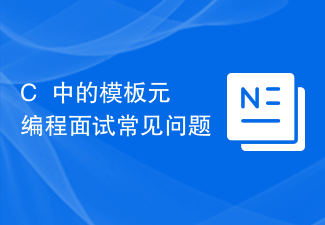
C++是一门广泛应用于各个领域的编程语言,其模板元编程是一种高级编程技术,可让程序员在编译时对类型和数值进行变换。在C++中,模板元编程是一个广泛讨论的话题,因此在面试中,与此相关的问题也是相当常见的。以下是一些可能会被问到的C++中的模板元编程面试常见问题。什么是模板元编程?模板元编程是一种在编译时操作类型和数值的技术。它使用模板和元函数来根据类型和值生成
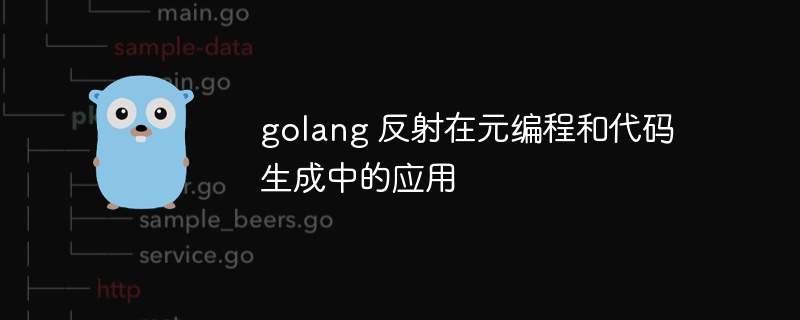
反射在Go语言中的元编程和代码生成中十分有用:元编程:允许程序在运行时创建新类型、函数和变量,修改现有类型结构。代码生成:可以动态生成代码片段,并在运行时执行它们,例如生成实现特定接口的函数。
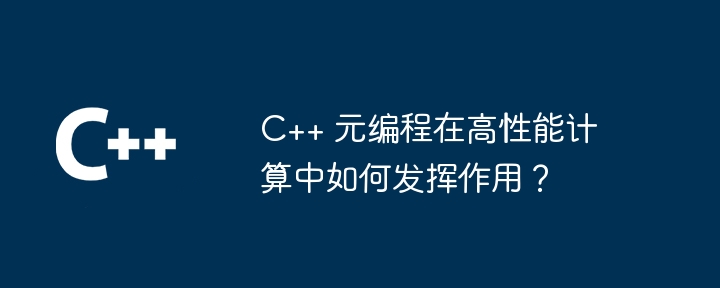
C++元编程在HPC中发挥着至关重要的作用,通过其操作和生成代码的能力,它为优化代码性能和可维护性提供了强大的工具。具体应用包括:SIMD矢量化:创建针对特定SIMD处理器定制的代码,以利用处理器能力,提升性能。代码生成:使用模板动态创建和优化代码,提高代码的可维护性。内省:在运行时查看和修改代码结构,增强代码的可调试性和灵活性。元数据编程:处理数据和元数据之间的关系,实现数据驱动的编程。
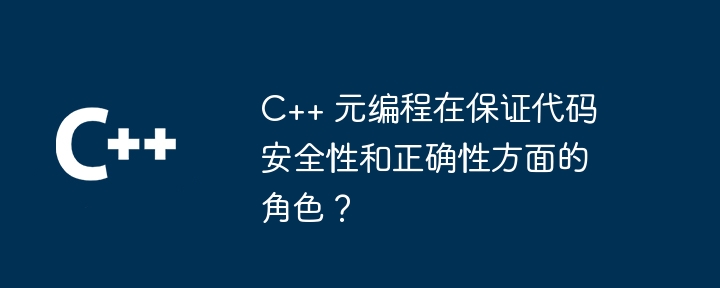
元编程可显著提高C++代码的安全性、正确性和可维护性。其基于以下能力:检查代码中的类型信息,以实现静态断言。使用模板形而上学生成类型安全的代码。在错误处理中静态检查错误条件。
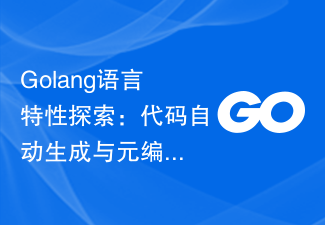
Golang语言特性探索:代码自动生成与元编程引言:Golang作为一门现代化的编程语言,具有简洁、高效和并发性强等诸多优势。除了这些基本特性之外,Golang还提供了一些高级的特性,如代码自动生成和元编程。本文将深入探讨这两个特性,并通过代码示例来展示它们的使用。一、代码自动生成代码自动生成是一种通过编写模板代码来生成特定代码的技术。这种技术可以减少重复性
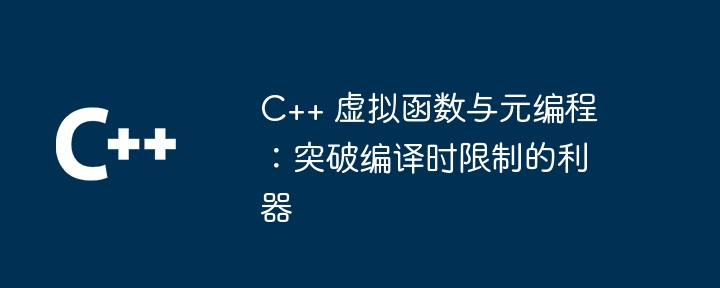
虚拟函数和元编程是C++中克服编译时限制的利器,可实现复杂且可扩展的代码。虚拟函数支持多态,元编程允许在编译时操作和生成代码。通过结合使用它们,我们可以创建通用数据结构、动态生成代码等等,从而编写出更加灵活、高效的C++代码。
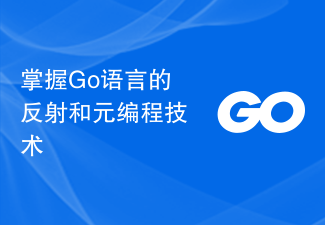
掌握Go语言的反射和元编程技术简介:随着计算机科技的不断发展,我们对于编程语言的要求也越来越高。Go语言作为一门现代化的编程语言,其简洁性、高效性和可靠性都受到广大开发者的认可。Go语言不仅提供了丰富的标准库,还支持强大的反射(reflection)和元编程(metaprogramming)技术,使得我们能够在运行时动态地获取和操作程序的结构信息。掌握Go语
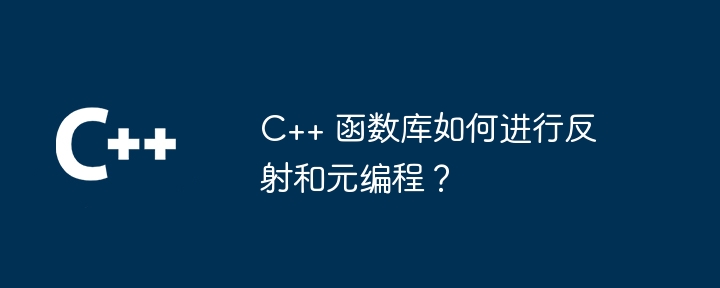
C++中的反射和元编程技术允许开发者在运行时检查和操作类型信息,并通过编译时技术生成或修改代码。反射使用typeid关键字返回指定类型的类型信息,而元编程使用模板元编程或预处理器宏实现。元编程可生成元组、进行类型转换等操作,实战案例中可用于运行时类型检查,通过检查对象类型调用不同的方法。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor
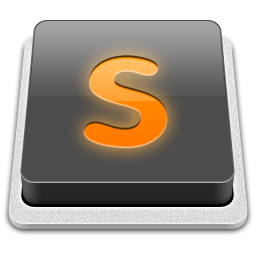
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
