


Best practices for data modeling and persistence in PHP frameworks
In the PHP framework, best practices for data modeling include using appropriate data types, applying constraints, considering indexes, and using ER diagrams. Best practices for persistence include using ORM tools, DAOs, handling transactions, and enforcing data validation. Following these practices ensures maintainable, efficient, and scalable code and maintains data integrity and reliability.
Best practices for data modeling and persistence in the PHP framework
In the PHP framework, data modeling and persistence ization are crucial aspects that determine how effectively an application can store and manage data. Following best practices is critical to ensuring maintainable, efficient, and scalable code.
Data Modeling
- Use appropriate data types: For each attribute choose an appropriate data type that is suitable for storing the data (e.g. integers, floating point numbers, strings, etc.).
- Apply constraints: Define constraints for each table and column, such as primary key, unique key, non-null, etc. to ensure data integrity.
- Consider indexes: Create indexes on frequently queried columns to improve query performance.
- Use Entity Relationship Diagram (ER): Use ER diagram to visualize the data model and determine the relationships between entities.
Persistence
- Use ORM tools: For example, Doctrine, Eloquent, these tools automatically process data through object-relational mapping Persistence.
- Use Data Access Object (DAO): DAO is a class specifically responsible for interacting with the database, which can isolate business logic and data access code.
- Processing transactions: Use transactions to ensure the consistency of data operations and prevent partial success or failure scenarios.
- Forced data validation: Use data validation classes or annotations to ensure that the data complies with business rules before persistence.
Practical case
Eloquent ORM example
Model class:
namespace App\Models; use Illuminate\Database\Eloquent\Model; class User extends Model { protected $table = 'users'; public $timestamps = false; protected $fillable = ['name', 'email', 'password']; }
Data access and persistence:
$user = new User(); $user->name = 'John Doe'; $user->email = 'johndoe@example.com'; $user->password = 'secret'; $user->save();
Using DAO:
DAO class:
namespace App\Dao; use PDO; class UserDao { private $db; public function __construct(PDO $db) { $this->db = $db; } public function create(array $data) { $stmt = $this->db->prepare('INSERT INTO users (name, email, password) VALUES (?, ?, ?)'); $stmt->execute([$data['name'], $data['email'], $data['password']]); } }
Data access and persistence:
$dao = new UserDao($db); $dao->create(['name' => 'John Doe', 'email' => 'johndoe@example.com', 'password' => 'secret']);
Following these best practices can significantly improve the efficiency and maintainability of data modeling and persistence in the PHP framework. By carefully applying these concepts, developers can create robust, scalable applications that maintain data integrity and reliability.
The above is the detailed content of Best practices for data modeling and persistence in PHP frameworks. For more information, please follow other related articles on the PHP Chinese website!
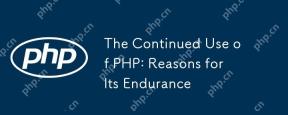
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
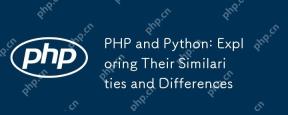
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
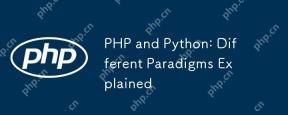
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
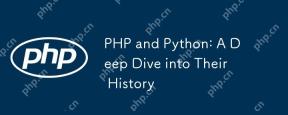
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
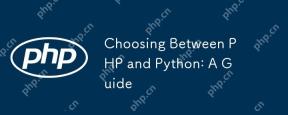
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
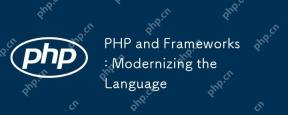
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
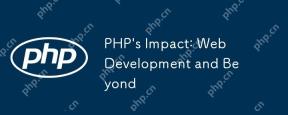
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
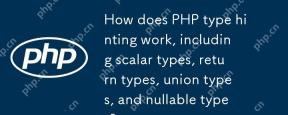
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
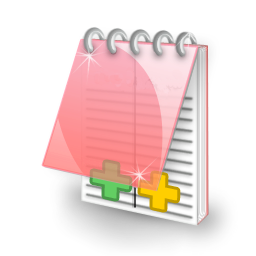
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool