The Java framework provides exception and error handling mechanisms to help write robust and reliable applications. Exceptions represent unexpected events during program execution and can be handled through try-catch blocks, while errors represent serious problems that the application cannot recover from and need to be handled through Thread.UncaughtExceptionHandler. Best practices include using appropriate exception types, catching necessary exceptions, providing meaningful error messages, and using logging to record errors.
Exception and error handling in the Java framework
The Java framework provides a series of mechanisms to handle exceptions and errors in applications . Understanding these mechanisms is critical to writing robust and reliable applications.
Exception
- What is an exception? An exception is an unexpected event that occurs during program execution and causes the program to deviate from the normal execution flow.
-
Exception handling in Java: Java uses the
Exception
class and its subclasses to represent exceptions. Exceptions can be handled via atry-catch
block or athrows
statement. -
Practical case: Consider an application that reads files. If the file does not exist,
FileNotFoundException
will be thrown. Applications can catch this exception and handle the file non-existence case via atry-catch
block.
try { // 读取文件代码 } catch (FileNotFoundException e) { // 处理文件不存在的情况 }
Error
- What is an error? An error is a serious problem from which the application cannot recover. Unlike exceptions, errors usually represent an incorrect state of the application or a programming error.
-
Error handling in Java: Errors are represented through the
Error
class and its subclasses. They cannot be caught by atry-catch
block, but can be handled by aThread.UncaughtExceptionHandler
. - Practical case: Consider a network connection error. This is an error that the application cannot recover from. It can be handled by registering an uncaught exception handler with your application.
Thread.setDefaultUncaughtExceptionHandler(new MyUncaughtExceptionHandler()); class MyUncaughtExceptionHandler implements Thread.UncaughtExceptionHandler { @Override public void uncaughtException(Thread t, Throwable e) { // 处理网络连接错误 } }
Best Practices
- Use appropriate exception types: Define specific exception types for different situations. This helps improve code readability and maintainability.
- Catch necessary exceptions: Only catch exceptions related to application logic. Uncaught exceptions terminate the application.
- Provide meaningful error messages: Include enough information in exception and error messages to help debug problems.
- Use logging to record errors: Errors should be logged to a log file for further analysis and troubleshooting.
The above is the detailed content of How does the java framework handle exceptions and errors?. For more information, please follow other related articles on the PHP Chinese website!
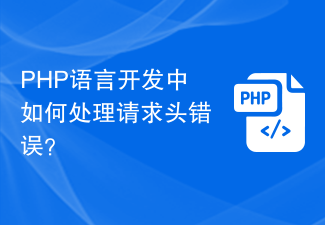
在PHP语言开发中,请求头错误通常是由于HTTP请求中的一些问题导致的。这些问题可能包括无效的请求头、缺失的请求体以及无法识别的编码格式等。而正确处理这些请求头错误是保证应用程序稳定性和安全性的关键。在本文中,我们将讨论一些处理PHP请求头错误的最佳实践,帮助您构建更加可靠和安全的应用程序。检查请求方法HTTP协议规定了一组可用的请求方法(例如GET、POS
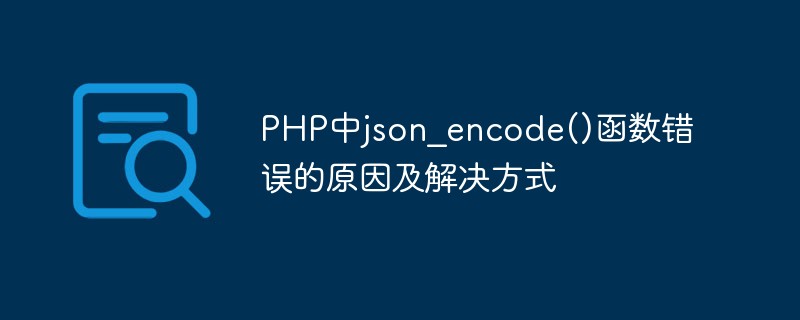
随着Web应用程序的不断发展,数据交互成为了一个非常重要的环节。其中,JSON(JavaScriptObjectNotation)是一种轻量级的数据交换格式,广泛用于前后端数据交互。在PHP中,json_encode()函数可以将PHP数组或对象转换为JSON格式字符串,json_decode()函数可以将JSON格式字符串转换为PHP数组或对象。然而,
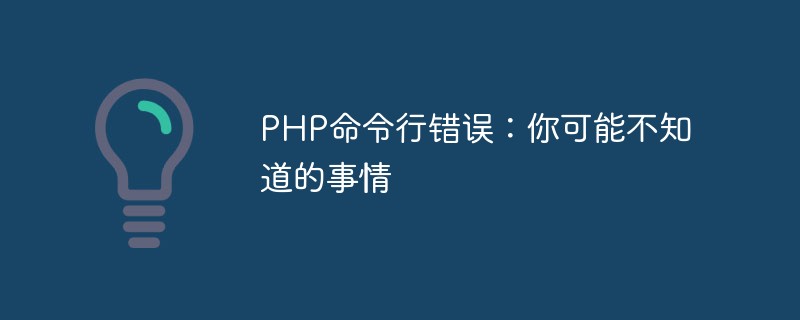
本文将介绍关于PHP命令行错误的一些你可能不知道的事情。PHP作为一门流行的服务器端语言,一般运行在Web服务器上,但它也可以在命令行上直接运行,比如在Linux或者MacOS系统下,我们可以在终端中输入“php”命令来直接运行PHP脚本。不过,就像在Web服务器中一样,当我们在命令行中运行PHP脚本时,也会遇到一些错误。以下是一些你可能不知道的有关PHP命
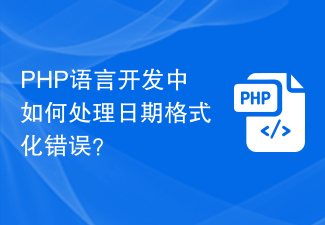
在PHP语言开发中,日期格式化错误是一个常见的问题。正确的日期格式对于程序员来说十分重要,因为它决定着代码的可读性、可维护性和正确性。本文将分享一些处理日期格式化错误的技巧。了解日期格式在处理日期格式化错误之前,我们必须先了解日期格式。日期格式是由各种字母和符号组成的字符串,用于表示特定的日期和时间格式。在PHP中,常见的日期格式包括:Y:四位数年份(如20
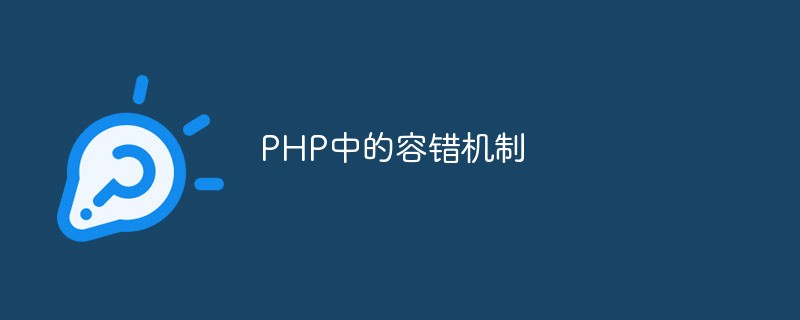
在编写程序时总会存在各种各样的错误和异常。任何编程语言都需要有良好的容错机制,PHP也不例外。PHP有许多内置的错误和异常处理机制,可以让开发者更好地管理其代码,并正确地处理各种问题。下面就让我们一起来了解一下PHP中的容错机制。错误级别PHP中有四个错误级别:致命错误、严重错误、警告和通知。每个错误级别都有一个不同的符号表示,以帮助识别和处理错误:E_ER
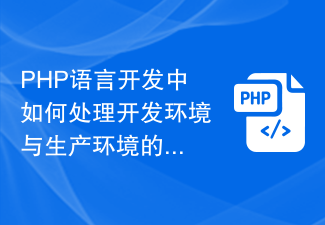
随着互联网的快速发展,开发人员的任务也随之多样化和复杂化。特别是对于PHP语言开发人员而言,在开发过程中面临的最常见问题之一就是在开发环境和生产环境中,数据不一致的错误问题。因此,在开发PHP应用程序时,如何处理这些错误是开发人员必须面对的一个重要问题。开发环境和生产环境的区别首先需要明确的是,开发环境和生产环境是不同的,它们有着不同的设置和配置。在开发环境
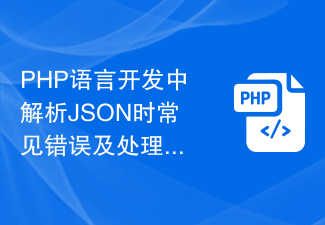
在PHP语言开发中,常常需要解析JSON数据,以便进行后续的数据处理和操作。然而,在解析JSON时,很容易遇到各种错误和问题。本文将介绍常见的错误和处理方法,帮助PHP开发者更好地处理JSON数据。一、JSON格式错误最常见的错误是JSON格式不正确。JSON数据必须符合JSON规范,即数据必须是键值对的集合,并使用大括号({})和中括号([])来包含数据。
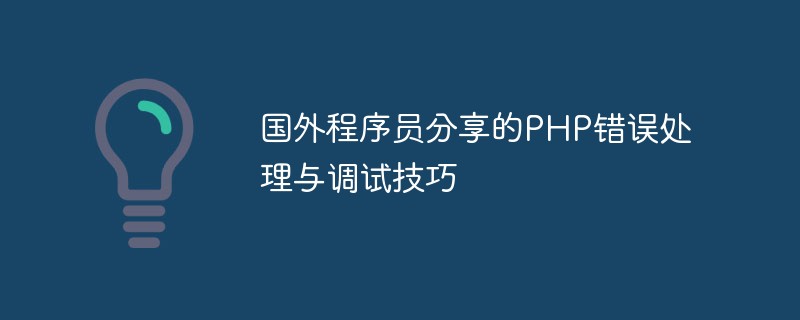
PHP(HypertextPreprocessor)是一种广泛用于Web开发的脚本语言。在开发PHP应用程序时,错误处理和调试被认为是非常重要的一块。国外程序员在经验中积累了许多PHP错误处理和调试技巧,下面介绍一些比较常见和实用的技巧。错误报告级别修改在PHP中,通过修改错误报告级别可以显示或禁止显示特定类型的PHP错误。通过设置错误报告级别为“E_AL


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
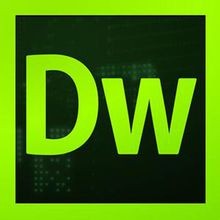
Dreamweaver CS6
Visual web development tools
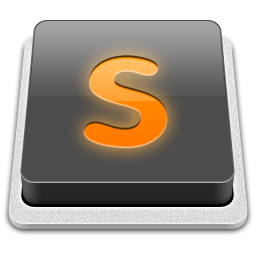
SublimeText3 Mac version
God-level code editing software (SublimeText3)
