Error handling and recovery strategies in Go concurrent programming
There are two methods of error handling in concurrent programming: active error handling (propagation of errors) and passive error handling (error pipeline). There are two recovery strategies: recovery (catch panic) and retry (multiple executions). Practical cases demonstrate the use of these four methods.
Error handling and recovery strategies in Go concurrent programming
Error handling
In Go concurrent programming, error handling is crucial . There are two main approaches to handling errors:
- Active Error Handling (Active Error Propagation): This approach relies on explicitly passing errors from one function to another function. If a function encounters an error, it returns that error, and the calling function needs to handle the error.
- Passive error handling (error pipe): This method uses channels to deliver errors. The function sends the error to the channel, and the calling function receives the error from the channel. This approach provides greater flexibility but increases code complexity.
Recovery strategy
In addition to error handling, there are also recovery strategies in concurrent programming. Recovery strategies are actions taken when a function fails to execute properly due to an error. There are two common recovery strategies:
-
Recovery: This strategy captures panics and resumes execution by using the built-in
recover
function. - Retry (Retry): This strategy attempts to perform the operation multiple times until it succeeds or reaches a predefined number of retries.
Practical case
Active error handling
func CalculateAverage(numbers []int) (float64, error) { if len(numbers) == 0 { return 0, errors.New("empty slice") } sum := 0 for _, number := range numbers { sum += number } return float64(sum) / float64(len(numbers)), nil } func main() { numbers := []int{1, 2, 3, 4, 5} average, err := CalculateAverage(numbers) if err != nil { fmt.Println(err) return } fmt.Println(average) }
Passive error handling
type ErrorChannel chan error func CalculateAverageWithChannel(numbers []int) ErrorChannel { ch := make(ErrorChannel) go func() { if len(numbers) == 0 { ch <- errors.New("empty slice") return } sum := 0 for _, number := range numbers { sum += number } ch <- nil close(ch) }() return ch } func main() { numbers := []int{1, 2, 3, 4, 5} ch := CalculateAverageWithChannel(numbers) for err := range ch { if err != nil { fmt.Println(err) return } fmt.Println("Average calculated successfully") } }
Recovery
func CalculateAverageWithRecovery(numbers []int) float64 { defer func() { if r := recover(); r != nil { fmt.Println("Error occurred:", r) } }() if len(numbers) == 0 { panic("empty slice") } sum := 0 for _, number := range numbers { sum += number } return float64(sum) / float64(len(numbers)) } func main() { numbers := []int{1, 2, 3, 4, 5} average := CalculateAverageWithRecovery(numbers) fmt.Println(average) }
Retry
func CalculateAverageWithRetry(numbers []int) (float64, error) { var err error = errors.New("empty slice") maxRetries := 3 for i := 0; i < maxRetries; i++ { if len(numbers) == 0 { err = errors.New("empty slice") continue } sum := 0 for _, number := range numbers { sum += number } return float64(sum) / float64(len(numbers)), nil } return 0, err } func main() { numbers := []int{1, 2, 3, 4, 5} average, err := CalculateAverageWithRetry(numbers) if err != nil { fmt.Println(err) } else { fmt.Println(average) } }
The above is the detailed content of Error handling and recovery strategies in Go concurrent programming. For more information, please follow other related articles on the PHP Chinese website!
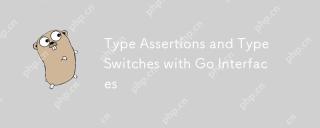
Gohandlesinterfacesandtypeassertionseffectively,enhancingcodeflexibilityandrobustness.1)Typeassertionsallowruntimetypechecking,asseenwiththeShapeinterfaceandCircletype.2)Typeswitcheshandlemultipletypesefficiently,usefulforvariousshapesimplementingthe
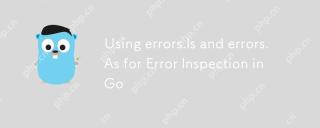
Go language error handling becomes more flexible and readable through errors.Is and errors.As functions. 1.errors.Is is used to check whether the error is the same as the specified error and is suitable for the processing of the error chain. 2.errors.As can not only check the error type, but also convert the error to a specific type, which is convenient for extracting error information. Using these functions can simplify error handling logic, but pay attention to the correct delivery of error chains and avoid excessive dependence to prevent code complexity.
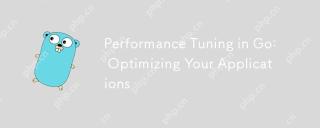
TomakeGoapplicationsrunfasterandmoreefficiently,useprofilingtools,leverageconcurrency,andmanagememoryeffectively.1)UsepprofforCPUandmemoryprofilingtoidentifybottlenecks.2)Utilizegoroutinesandchannelstoparallelizetasksandimproveperformance.3)Implement
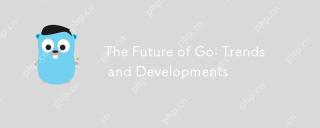
Go'sfutureisbrightwithtrendslikeimprovedtooling,generics,cloud-nativeadoption,performanceenhancements,andWebAssemblyintegration,butchallengesincludemaintainingsimplicityandimprovingerrorhandling.
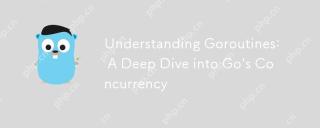
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
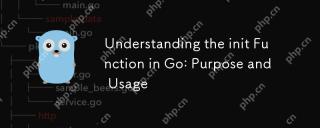
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
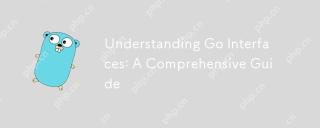
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
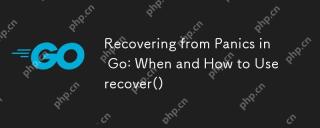
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use
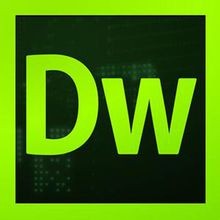
Dreamweaver CS6
Visual web development tools
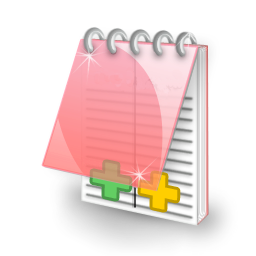
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
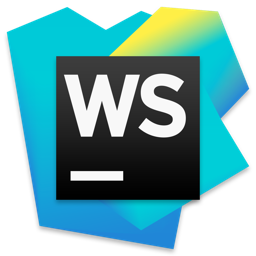
WebStorm Mac version
Useful JavaScript development tools
