WebSocket over TLS (WSS) uses the TLS protocol to encrypt Go WebSocket communications, ensuring data confidentiality and integrity. The specific steps are as follows: Create and configure the server, and use the cert.pem and key.pem files for TLS configuration. The client connects to the server using TLS configuration (possibly with certificate verification disabled). Data transmitted via WebSocket communication is encrypted using TLS.
Go WebSocket: How to use TLS encryption
Encryption becomes crucial when using WebSocket in Go for real-time two-way communication important. WebSocket over TLS (WSS) uses the TLS (Transport Layer Security) protocol to secure communications between client and server, ensuring data confidentiality and integrity.
Practical Case
To demonstrate how to use TLS to encrypt Go WebSocket, we create a simple server and client:
Server code:
package main import ( "crypto/tls" "net/http" "time" "github.com/gorilla/websocket" ) func main() { // 创建用于 TLS 配置的 cert.pem 和 key.pem 文件 cert, _ := tls.LoadX509KeyPair("cert.pem", "key.pem") config := &tls.Config{Certificates: []tls.Certificate{cert}} listener, _ := tls.Listen("tcp", ":8443", config) http.HandleFunc("/ws", func(w http.ResponseWriter, r *http.Request) { upgrader := websocket.Upgrader{ ReadBufferSize: 1024, WriteBufferSize: 1024, } conn, _ := upgrader.Upgrade(w, r, nil) for { // ... 处理 WebSocket 连接 ... } }) http.Serve(listener, nil) }
Client code:
package main import ( "crypto/tls" "fmt" "log" "net/http" "time" "github.com/gorilla/websocket" ) func main() { config := &tls.Config{InsecureSkipVerify: true} dialer := &websocket.Dialer{ TLSClientConfig: config, HandshakeTimeout: 10 * time.Second, } conn, _, err := dialer.Dial("wss://localhost:8443/ws", nil) if err != nil { log.Fatal("Error connecting to the WebSocket server:", err) } for { // ... 处理 WebSocket 连接 ... } }
Configure TLS certificate
Please note that the server code needs to use cert.pem and key. pem file to configure the TLS certificate. You can use OpenSSL or a similar tool to generate a self-signed certificate, or use a certificate signed by a trusted certificate authority.
Secure WebSocket Connection
Clients connect to the WebSocket server using a TLS configuration that disables certificate verification via InsecureSkipVerify. In a production environment, the server's certificate should be verified using a valid certificate issued by a trusted certificate authority.
With this configuration, WebSocket connections will use TLS encryption, ensuring the confidentiality and integrity of transmitted data.
The above is the detailed content of How does Go WebSocket use TLS encryption?. For more information, please follow other related articles on the PHP Chinese website!
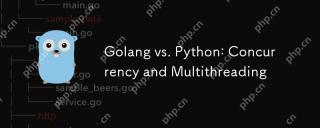
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
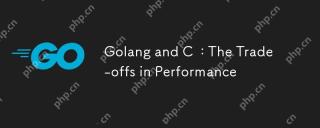
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
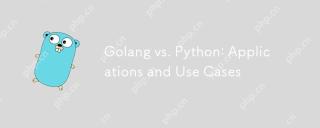
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
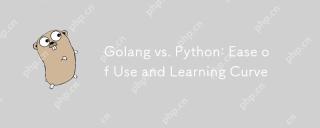
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
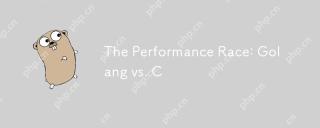
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
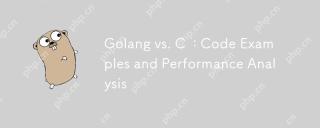
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
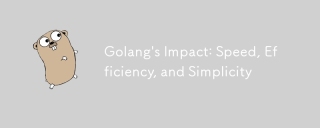
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
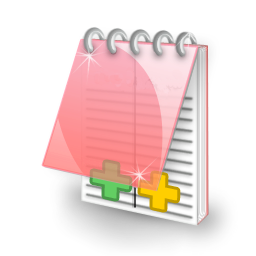
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.