To parse time strings with time zones in Go, you can use the time package: use the time.Parse() function to parse time strings and specify the time format and string. For different time zones, use the time.ParseInLocation() function, specifying the format string, time string, and time zone location. To convert time zones, use the time.In() function, specifying the new time zone location. Practical analysis of API responses with time zones, using time.Parse() and the standard format time.RFC3339.
#How to use Golang to parse a time string with time zone?
In Golang, you can use the time
package to parse time strings with time zones. The following are the specific steps:
- Use the time.Parse() function to parse the time string
t, err := time.Parse("2006-01-02 15:04:05 MST", "2018-10-29 14:45:00 MST") if err != nil { log.Fatal(err) }
-
time.Parse ()
The function takes two parameters: time format string and time string. - The time format string uses Go's time layout rules.
- The time string must conform to the specified format.
- Parsing time strings with different time zones
In order to parse time strings with different time zones, you can use time.ParseInLocation()
Function:
loc, err := time.LoadLocation("America/Los_Angeles") if err != nil { log.Fatal(err) } t, err := time.ParseInLocation("2006-01-02 15:04:05", "2018-10-29 14:45:00", loc) if err != nil { log.Fatal(err) }
-
time.ParseInLocation()
The function takes three parameters: time format string, time string and a time zone Location. - The time zone location can be obtained using the
time.LoadLocation()
function.
- Handling time zone conversion
If you need to convert the parsed time to a different time zone, you can use time. In()
Function:
loc, err := time.LoadLocation("America/New_York") if err != nil { log.Fatal(err) } t = t.In(loc)
-
time.In()
The function takes a time zone location as a parameter and returns a time converted to that time zone.
Practical case: Parsing API responses with time zones
Consider a scenario where a time string with time zones is obtained from an API response. The string is in the following format:
2018-10-29T14:45:00Z
To parse this time string:
t, err := time.Parse(time.RFC3339, "2018-10-29T14:45:00Z") if err != nil { log.Fatal(err) }
time.RFC3339
is a standard time format that represents a date and time with a time zone.
The above is the detailed content of How to parse time string with time zone using Golang?. For more information, please follow other related articles on the PHP Chinese website!
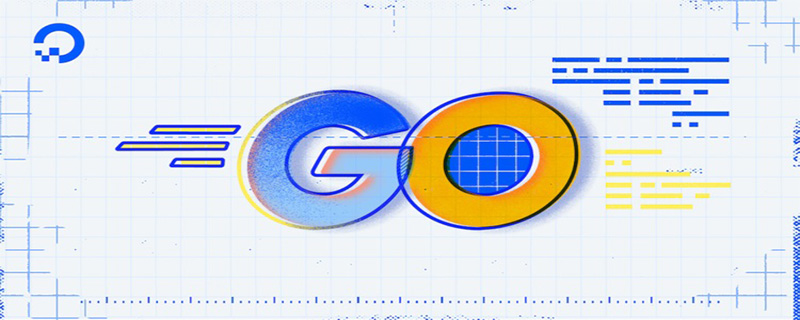
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
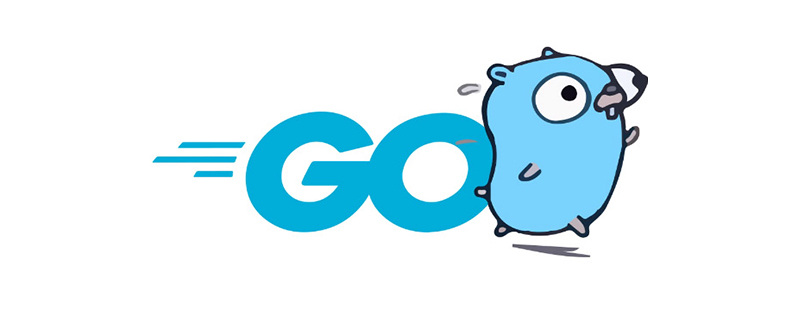
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
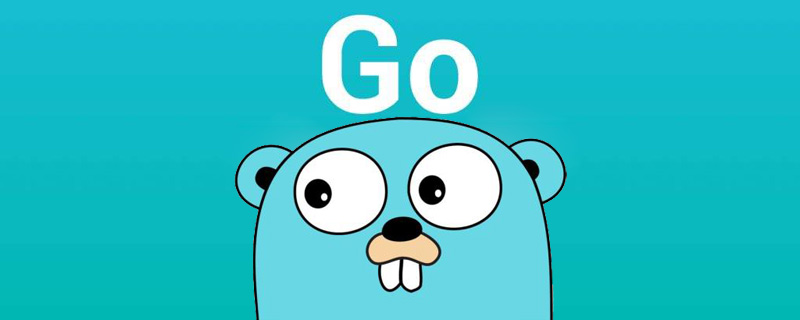
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
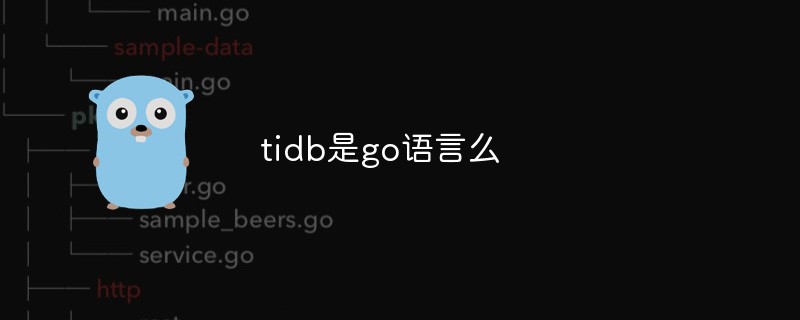
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
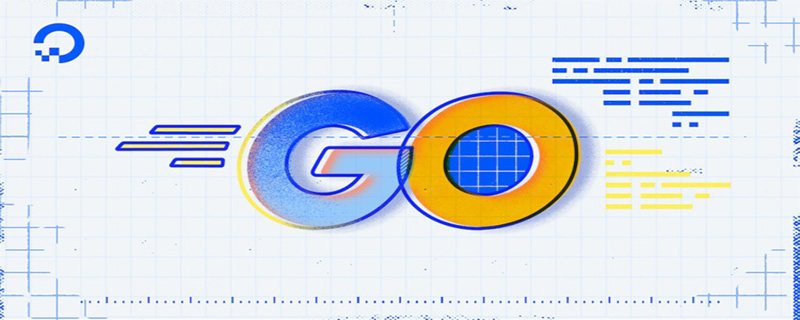
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
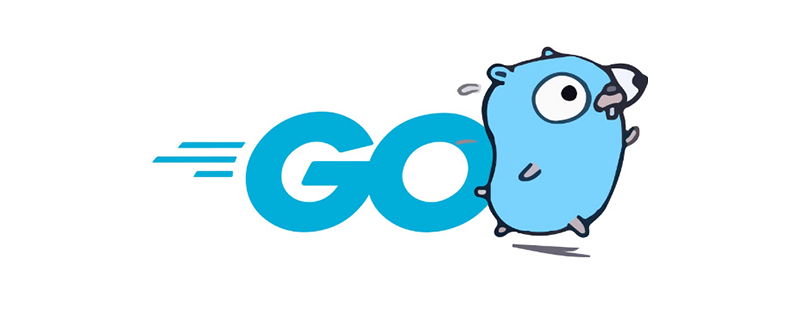
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
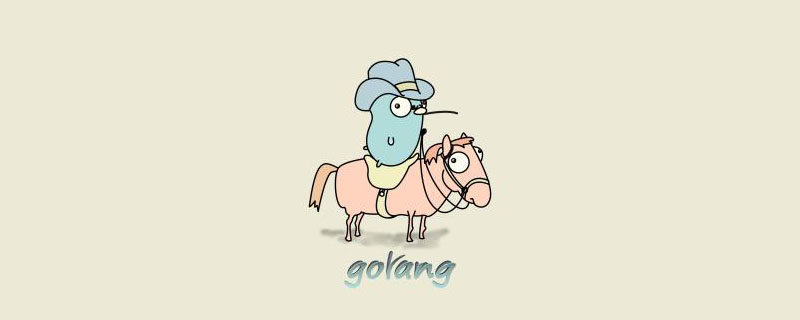
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
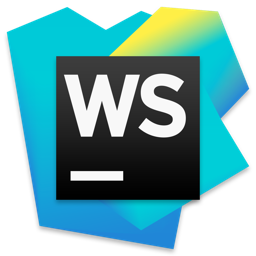
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
