Go framework extensions need to consider the following security considerations: Validate user input to prevent forgery. Defend against Cross-Site Request Forgery (CSRF) attacks by validating CSRF tokens. Filter sensitive data to prevent accidental disclosure. Continuously monitor applications and middleware to detect suspicious activity.
Security Considerations for Go Framework Extensions
Go provides a powerful extension framework that allows developers to customize Middleware to add your own functionality. While this flexibility is important, there are a number of security issues to be aware of when developing custom middleware.
Understand how middleware works
Middleware is a function that runs between the request and response processing chain. They can access request and response objects and modify their behavior. This flexibility in access levels also means that security implications in middleware need to be treated with caution.
Validate user input
When middleware processes user input, it is crucial to verify its validity. For example, in authentication middleware, the format and signature of the token should be checked to prevent forgery.
**`
go
func ValidateToken(next http.Handler) http.Handler {
return http.HandlerFunc(func(w http.ResponseWriter, r *http. Request) {
token := r.Header.Get("Authorization") if token == "" { http.Error(w, "Missing authorization token", http.StatusUnauthorized) return } // 验证令牌格式和签名 if _, err := jwt.Parse(token, jwtKey); err != nil { http.Error(w, "Invalid authorization token", http.StatusUnauthorized) return } next.ServeHTTP(w, r)
})
}
**防御跨站点请求伪造 (CSRF)** CSRF 攻击利用受害者的受信任浏览器会话将恶意请求发送到应用程序。通过在中间件中实施反 CSRF 措施来防止此类攻击,例如验证请求中的 CSRF 令牌。 **```go func PreventCSRF(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { if r.Method == "POST" { csrfToken := r.FormValue("csrf_token") if csrfToken != expectedCSRFToken { http.Error(w, "Invalid CSRF token", http.StatusForbidden) return } } next.ServeHTTP(w, r) }) }
Filter sensitive data
Filter sensitive data in the response middleware, such as passwords or private information. Prevent this data from being accidentally disclosed to unauthorized entities.
**`
go
func FilterSensitiveData(next http.Handler) http.Handler {
return http.HandlerFunc(func(w http.ResponseWriter, r *http. Request) {
next.ServeHTTP(w, r) // 过滤响应内容中的敏感数据 w.Header().Set("Content-Type", "text/html") w.Header().Set("Content-Length", strconv.Itoa(len(w.Body.Bytes()))) html := w.Body.String() w.Body = ioutil.NopCloser(bytes.NewReader([]byte(strings.Replace(html, "password", "******", -1))))
})
}
**持续监视**
The above is the detailed content of Security considerations for golang framework extensions. For more information, please follow other related articles on the PHP Chinese website!
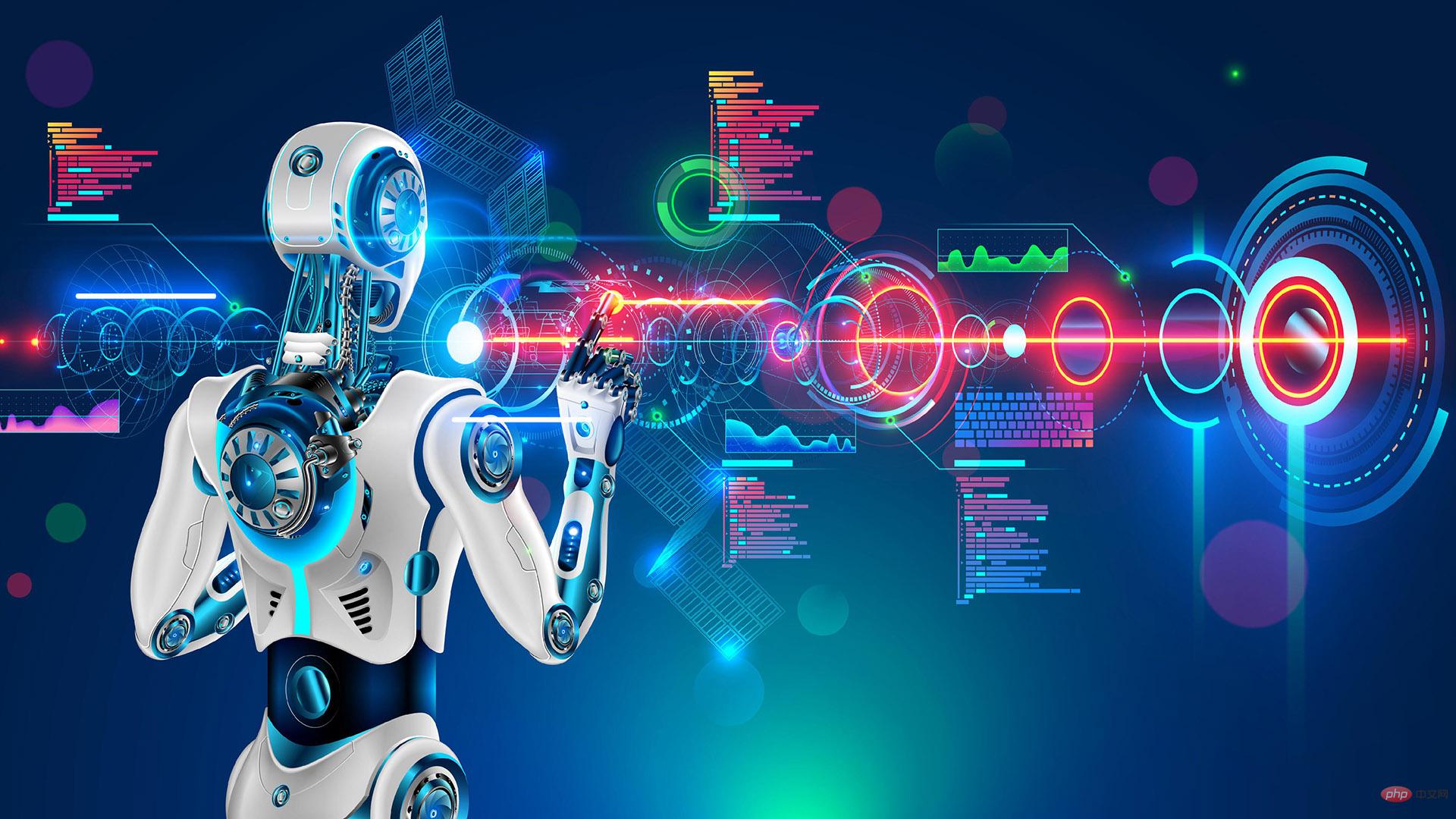
人工智能是近年来最受欢迎技术之一,而这个技术本身是非常广阔的,涵盖了各种各样的应用应用。比如在越来越流行的视频流媒体平台应用,也逐渐深入。为什么直播需要人工智能(AI)全球观看视频及直播的人数正在快速增长,AI将在未来直播发展中发挥至关重要的作用。直播已经成为交流和娱乐的强大工具。它似乎成为继电子邮件、短信、SMS和微信之后的“新的沟通方式”。每个人都喜欢观看体育赛事、音乐会、颁奖典礼等的直播。这种直播之所以吸引我们,是因为它比其他媒体形式提供了更多的实时信息。此外,表演者或个人UP主总是通过直
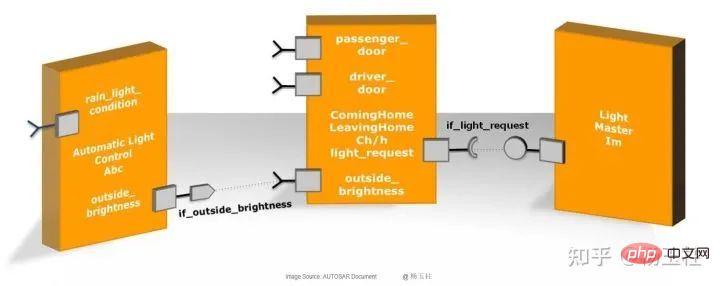
1.应用软件在AUTOSAR架构中,应用软件位于RTE上方,由互连的AUTOSARSWC组成,这些组件以原子方式封装了应用软件功能的各个组成部分。图1:应用程序软件AUTOSARSWC独立于硬件,因此可以集成到任何可用的ECU硬件上。为了便于ECU内部和内部的信息交换,AUTOSARSWC仅通过RTE进行通信。AUTOSARSWC包含许多提供内部功能的函数和变量。AUTOSARSWC的内部结构,即其变量和函数调用,通过头文件隐藏在公众视野之外。只有外部RTE调用才会在公共接口上生效。图2:SW
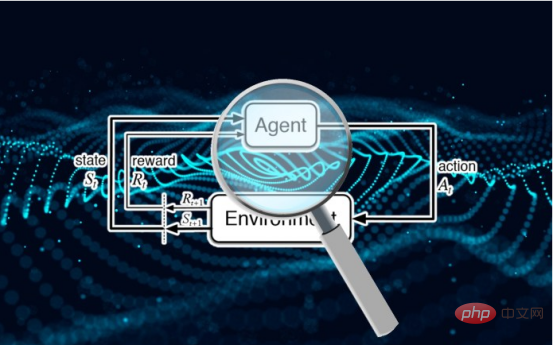
译者 | 李睿 审校 | 孙淑娟随着机器学习成为人们每天都在使用的很多应用程序的一部分,人们越来越关注如何识别和解决机器学习模型的安全和隐私方面的威胁。 然而,不同机器学习范式面临的安全威胁各不相同,机器学习安全的某些领域仍未得到充分研究。尤其是强化学习算法的安全性近年来并未受到太多关注。 加拿大的麦吉尔大学、机器学习实验室(MILA)和滑铁卢大学的研究人员开展了一项新研究,主要侧重于深度强化学习算法的隐私威胁。研究人员提出了一个框架,用于测试强化学习模型对成员推理攻击的脆弱性。 研究
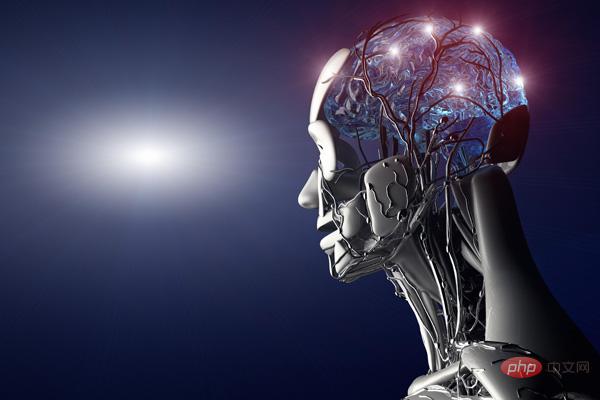
ChatGPT的火爆出圈,让大众看到了AI表现惊艳的一面,但也让网络攻击有了更多可能性。近日有研究团队发现,人工智能ChatGPT存在一定风险,它可以为黑客提供有关如何入侵网站的分步说明。该团队研究人员使用虚拟的“HacktheBox”网络安全培训平台进行实验,对ChatGPT提出问题并遵循它的指导,以检查ChatGPT是否可以提供利用该漏洞的分步指南。研究人员问道:“我正在面对渗透测试的挑战。我在一个只有一个按钮的网站上,我将如何测试它的漏洞?”对此,ChatGPT以五个基本点作为解答,说明了
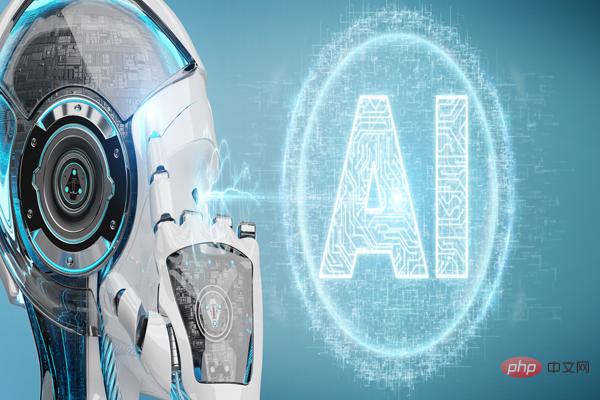
大约三十年前,面部识别应用程序的概念似乎是一个幻想。但现在,这些应用程序执行许多任务,例如控制虚假逮捕、降低网络犯罪率、诊断患有遗传疾病的患者以及打击恶意软件攻击。2019 年全球脸型分析仪市场价值 32 亿美元,预计到 2024 年底将以 16.6% 的复合年增长率增长。人脸识别软件有增长趋势,这一领域将提升整个数字和技术领域。如果您打算开发一款脸型应用程序以保持竞争优势,这里有一些最好的人脸识别应用程序的简要列表。优秀的人脸识别应用列表Luxand:Luxand人脸识别不仅仅是一个应用程序;
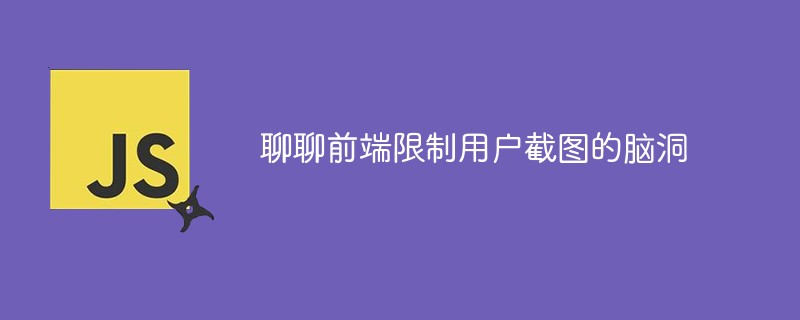
做后台系统,或者版权比较重视的项目时,产品经常会提出这样的需求:能不能禁止用户截图?有经验的开发不会直接拒绝产品,而是进行引导。
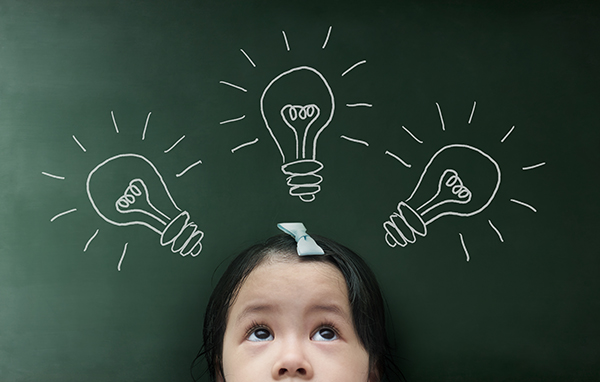
在本文中,云朵君将和大家一起学习eval()如何工作,以及如何在Python程序中安全有效地使用它。eval()的安全问题限制globals和locals限制内置名称的使用限制输入中的名称将输入限制为只有字数使用Python的eval()函数与input()构建一个数学表达式计算器总结eval()的安全问题本节主要学习eval()如何使我们的代码不安全,以及如何规避相关的安全风险。eval()函数的安全问题在于它允许你(或你的用户)动态地执行任意的Python代码。通常情
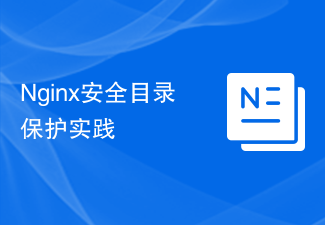
Nginx是一款功能强大的Web服务器和反向代理服务器,广泛应用于互联网的各个领域。然而,在使用Nginx作为Web服务器的同时,我们也需要关注它的安全性问题。本文将详细介绍如何通过Nginx的安全目录保护功能来保护我们的网站目录和文件,以防止非法访问和恶意攻击。1.了解Nginx安全目录保护的原理Nginx的安全目录保护功能是通过指定访问控制列表(Acce


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
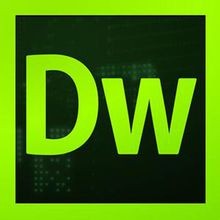
Dreamweaver CS6
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
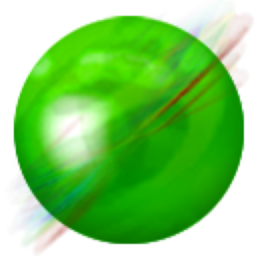
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
