Ways to prevent file upload corruption in Go include: using hashing algorithms to verify integrity, using streaming to avoid memory overflows, using chunked uploads to tolerate network interruptions, and using secure connections to keep data safe. A practical example shows how to detect file corruption by comparing the hashes of the original and received files.
Prevent file upload corruption in Go
Data corruption is a common mistake when handling file uploads. This is usually caused by errors during data transfer. To prevent this, it is crucial to take steps to protect uploaded data.
This article will introduce the best practices for preventing file upload corruption in Go, and provide a practical case to demonstrate how to implement these practices.
Best Practices
- Use a hash algorithm to verify file integrity: When uploading a file, use a hash algorithm (such as MD5 or SHA256) to calculate the hash value of the file. After receiving the file, the hash is calculated again and compared to the original hash. If they don't match, the file is corrupted.
- Use streaming to avoid memory overflow: For large files, processing the entire file at once may cause memory overflow. Use streaming processing to read and process files block by block to avoid taking up too much memory.
- Use chunked upload: For very large files, you can use chunked upload to split them into smaller chunks. This improves fault tolerance and avoids entire file uploads failing due to network outages or server failures.
- Use a secure connection: Make sure to use a secure connection (such as HTTPS) to transfer files to prevent data leakage or tampering.
Practical Case
package main import ( "crypto/md5" "fmt" "io" "io/ioutil" "net/http" "os" ) func main() { // 监听端口 8080 http.HandleFunc("/", handleUpload) http.ListenAndServe(":8080", nil) } func handleUpload(w http.ResponseWriter, r *http.Request) { // 获取上传的文件 file, _, err := r.FormFile("file") if err != nil { http.Error(w, "Error retrieving file", http.StatusBadRequest) return } // 计算原始文件的 MD5 哈希值 originalHash := md5.New() io.Copy(originalHash, file) originalHashString := fmt.Sprintf("%x", originalHash.Sum(nil)) // 创建临时文件保存上传的文件 tmpFile, err := ioutil.TempFile("", "file-upload") if err != nil { http.Error(w, "Error creating temporary file", http.StatusInternalServerError) return } defer os.Remove(tmpFile.Name()) // 将文件内容保存到临时文件中 if _, err := io.Copy(tmpFile, file); err != nil { http.Error(w, "Error saving file", http.StatusInternalServerError) return } // 计算接收文件的 MD5 哈希值 receivedHash := md5.New() receivedFile, err := os.Open(tmpFile.Name()) if err != nil { http.Error(w, "Error opening temporary file", http.StatusInternalServerError) return } io.Copy(receivedHash, receivedFile) receivedHashString := fmt.Sprintf("%x", receivedHash.Sum(nil)) // 比较哈希值 if originalHashString != receivedHashString { http.Error(w, "File was corrupted during upload", http.StatusBadRequest) return } // 成功上传文件 w.Write([]byte("File uploaded successfully")) }
Conclusion
By following the best practices in this article, you can effectively Prevent file upload corruption in Go and ensure data integrity and reliability.
The above is the detailed content of How to prevent file corruption when uploading Golang files?. For more information, please follow other related articles on the PHP Chinese website!
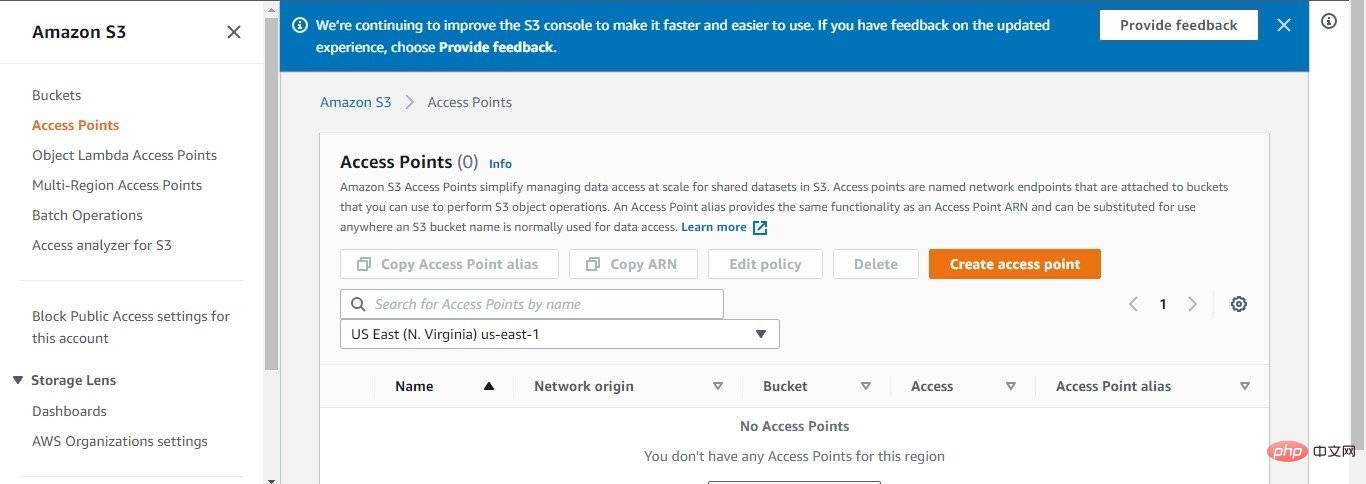
Amazon Simple Storage Service,简称Amazon S3,是一种使用 Web 界面提供存储对象的存储服务。Amazon S3 存储对象可以存储不同类型和大小的数据,从应用程序到数据存档、备份、云存储、灾难恢复等等。该服务具有可扩展性,用户只需为存储空间付费。Amazon S3 有四个基于可用性、性能率和持久性的存储类别。这些类包括 Amazon S3 Standard、Amazon S3 Standard Infrequent Access、Amazon S3 One
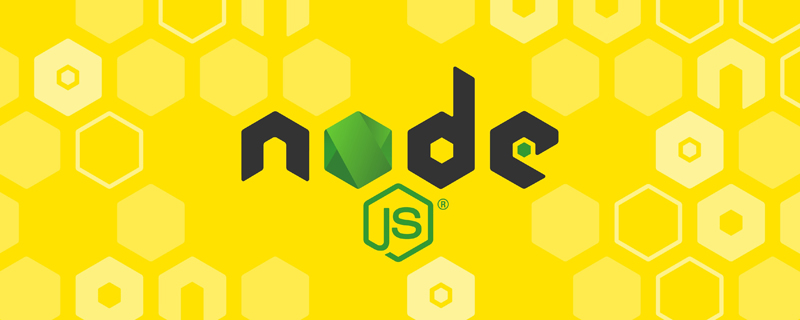
怎么处理文件上传?下面本篇文章给大家介绍一下node项目中如何使用express来处理文件的上传,希望对大家有所帮助!
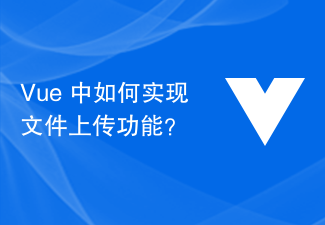
Vue作为目前前端开发最流行的框架之一,其实现文件上传功能的方式也十分简单优雅。本文将为大家介绍在Vue中如何实现文件上传功能。HTML部分在HTML文件中添加如下代码,创建上传表单:<template><div><formref="uploadForm"enc
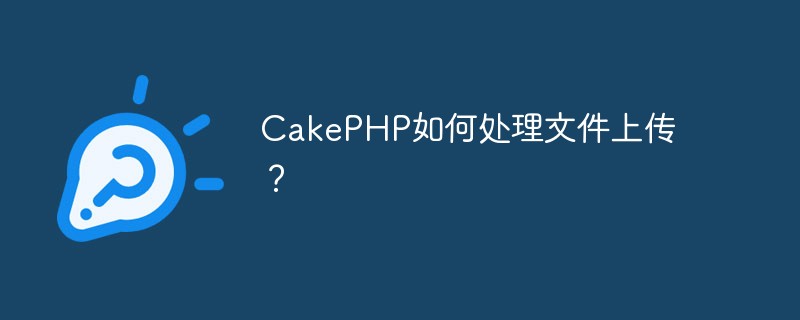
CakePHP是一个开源的Web应用程序框架,它基于PHP语言构建,可以简化Web应用程序的开发过程。在CakePHP中,处理文件上传是一个常见的需求,无论是上传头像、图片还是文档,都需要在程序中实现相应的功能。本文将介绍CakePHP中如何处理文件上传的方法和一些注意事项。在Controller中处理上传文件在CakePHP中,上传文件的处理通常在Cont
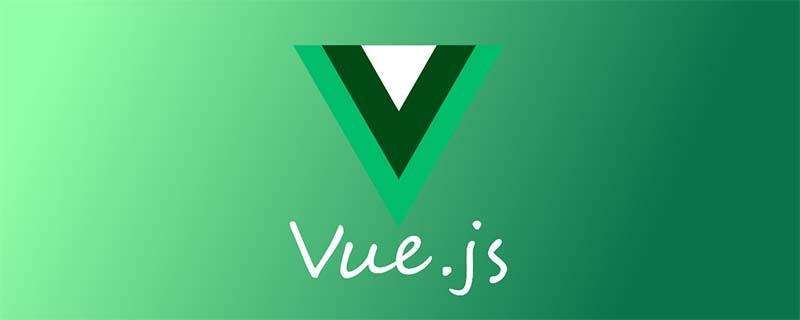
在实际开发项目过程中有时候需要上传比较大的文件,然后呢,上传的时候相对来说就会慢一些,so,后台可能会要求前端进行文件切片上传,很简单哈,就是把比如说1个G的文件流切割成若干个小的文件流,然后分别请求接口传递这个小的文件流。
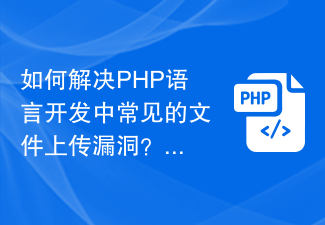
在Web应用程序的开发中,文件上传功能已经成为了基本的需求。这个功能允许用户向服务器上传自己的文件,然后在服务器上进行存储或处理。然而,这个功能也使得开发者更需要注意一个安全漏洞:文件上传漏洞。攻击者可以通过上传恶意文件来攻击服务器,从而导致服务器遭受不同程度的破坏。PHP语言作为广泛应用于Web开发中的语言之一,文件上传漏洞也是常见的安全问题之一。本文将介
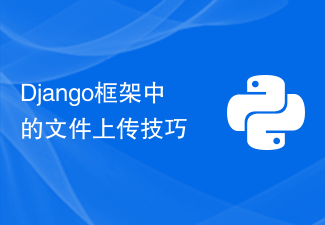
近年来,Web应用程序逐渐流行,而其中许多应用程序都需要文件上传功能。在Django框架中,实现上传文件功能并不困难,但是在实际开发中,我们还需要处理上传的文件,其他操作包括更改文件名、限制文件大小等问题。本文将分享一些Django框架中的文件上传技巧。一、配置文件上传项在Django项目中,要配置文件上传需要在settings.py文件中进
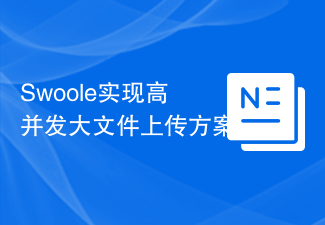
Swoole是一款基于PHP的高性能异步面向网络编程的框架,能够实现异步IO、多进程多线程、协程等特性,能够大幅提高PHP在网络编程方面的性能表现。在很多实时且高并发的应用场景下,Swoole已经成为了开发者的首选。本文将介绍如何使用Swoole实现高并发大文件上传的方案。一、传统方案的问题在传统的文件上传方案中,通常使用的是HTTP的POST请求方式,即将


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
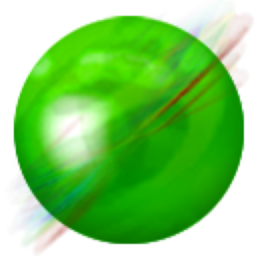
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
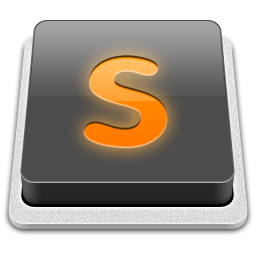
SublimeText3 Mac version
God-level code editing software (SublimeText3)
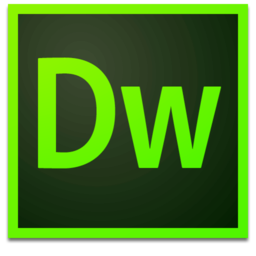
Dreamweaver Mac version
Visual web development tools
