Best practices for unit testing of Go framework source code: Use BDD style to write test cases to enhance readability. Use mock objects to simulate external dependencies and focus on the code under test. Cover all code branches to ensure the code runs correctly under all circumstances. Use coverage tools to monitor the scope of test cases and improve test reliability. Ensure the independence of test cases and facilitate problem isolation and debugging.
Go framework source code unit testing practice
Unit testing is a crucial link in software development and can help verify the code Correctness and robustness. For the Go framework source code, unit testing is particularly important because it can ensure the correct operation of the framework. This article will introduce the best practices for source code unit testing of the Go framework and demonstrate it through practical cases.
Best Practices
- Follow the BDD style: Write test cases using the BDD (Behavior-Driven Development) style, that is, Given-When -Then format. This helps improve the readability and maintainability of test cases.
- Use mock objects: Mock objects can simulate external dependencies, allowing you to focus on testing the correctness of the code under test without being affected by external factors.
- Cover all branches: Unit tests should cover all branches in the code, including true and false branches. This helps ensure that the code runs correctly under all circumstances.
- Use code coverage tools: Code coverage tools can help you monitor the coverage of test cases. Ensure a high level of coverage to improve test reliability.
- Keep test cases independent: Unit test cases should be independent of each other to avoid relying on other test cases. This helps isolate the problem and simplifies the debugging process.
Practical case
Let’s take the following simple Go function as an example:
func Sum(a, b int) int { return a + b }
Unit test
import ( "testing" ) func TestSum(t *testing.T) { tests := []struct { a, b, exp int }{ {1, 2, 3}, {-1, 0, -1}, {0, 5, 5}, } for _, test := range tests { t.Run("input: "+fmt.Sprintf("%d, %d", test.a, test.b), func(t *testing.T) { got := Sum(test.a, test.b) if got != test.exp { t.Errorf("expected: %d, got: %d", test.exp, got) } }) } }
In this test case, we used some best practices:
- Write test cases using BDD style.
- Use data-driven testing methods to manage different inputs and expected outputs.
- Create an independent subtest for each set of inputs.
Run the test
Run the unit test using the go test
command:
$ go test
The output should look similar to:
PASS ok command-line-arguments 0.535s
Conclusion
This article introduces the best practices for unit testing of Go framework source code and demonstrates it through practical cases. Following these best practices allows you to write robust and reliable unit tests, ensuring the correctness and reliability of your framework's code.
The above is the detailed content of Golang framework source code unit testing practice. For more information, please follow other related articles on the PHP Chinese website!
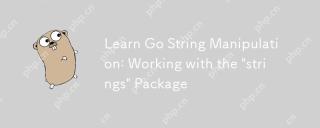
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
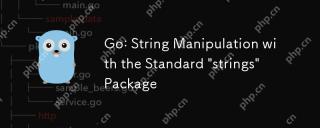
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
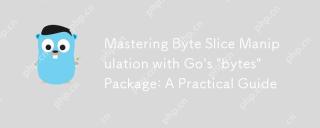
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
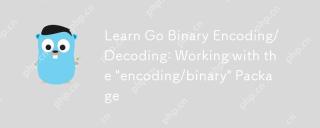
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
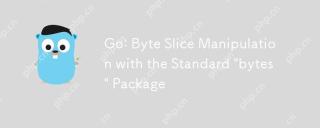
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
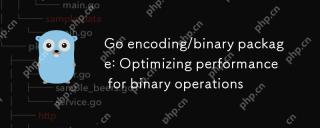
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
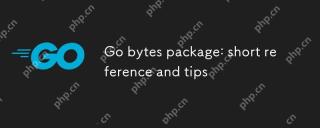
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
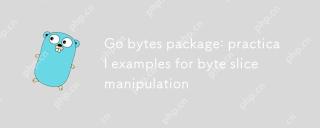
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
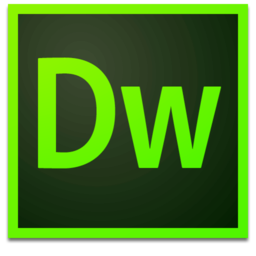
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
