Although design patterns in PHP have advantages, there are also misunderstandings and pitfalls when using them, such as blind use, violation of the single responsibility principle, confusion between inheritance and delegation, abuse of the factory method pattern and wrong implementation of the SOLID principle. Proper application of design patterns, such as separating the responsibility for calculating the total amount through the chain of responsibility pattern, can improve the modularity and maintainability of the code.
PHP Design Patterns: Common Misunderstandings and Traps
Design patterns are used to reuse code, reduce duplicate code and improve development efficiency Valuable tool. However, there are also some common misunderstandings and pitfalls when using design patterns in PHP:
Myth 1: Blind use of design patterns
is not suitable for use in all circumstances Design Patterns. Premature or overuse of design patterns can lead to unnecessary complexity and overhead. When choosing a design pattern, you should carefully consider its suitability and impact on your code.
Myth 2: Misunderstanding the Single Responsibility Principle (SRP)
SRP means that a class should only have one reason for change. Violating SRP results in loosely coupled, difficult-to-maintain code. Using design patterns such as compositional reuse, aggregation, and dependency injection can help enforce SRP.
Myth 3: Confusing inheritance and delegation
Inheritance is a way to create a new class and inherit its characteristics from an existing class. Delegation allows one class to delegate to another class to perform a specific task. Confusing inheritance and delegation can lead to scalability and maintainability problems in your code.
Myth 4: Abusing the Factory Method Pattern
The factory method pattern can help create and manage objects, but excessive use of it will create sacred objects (Singletons) and dependency injection containers (DI) container. Use the factory method pattern sparingly and only when you need to create objects of a specific type.
Myth 5: Wrong SOLID Implementation
The SOLID (Single Responsibility, Open-Closed, Liskov Substitution, Interface Isolation, and Dependency Inversion) principles provide good design, Guidance for maintainable code. However, if SOLID principles are applied incorrectly, it can lead to scalability issues and difficult-to-understand structures in your code.
Practical case:
Consider a shopping cart system, in which the Cart
class is responsible for managing the items in the user's shopping cart. We want to calculate the total amount based on the items in the shopping cart.
Bad implementation:
class Cart { private $items; public function __construct() { $this->items = []; } public function addItem(Item $item) { $this->items[] = $item; } public function calculateTotalAmount() { $total = 0; foreach ($this->items as $item) { $total += $item->getPrice(); } return $total; } }
This implementation violates the SRP because the Cart
class is responsible for both storing the item and calculating the total amount.
Improved implementation:
We can use the chain of responsibility pattern to separate the responsibility for calculating the total amount:
interface TotalCalculator { public function calculateTotal(array $items); } class ItemTotalCalculator implements TotalCalculator { public function calculateTotal(array $items) { $total = 0; foreach ($items as $item) { $total += $item->getPrice(); } return $total; } } class Cart { private $items; private $totalCalculator; public function __construct(TotalCalculator $totalCalculator) { $this->items = []; $this->totalCalculator = $totalCalculator; } public function addItem(Item $item) { $this->items[] = $item; } public function calculateTotalAmount() { return $this->totalCalculator->calculateTotal($this->items); } }
With the chain of responsibility pattern, we separate The responsibility of calculating the total amount is removed, making the Cart
code more modular and maintainable.
The above is the detailed content of PHP Design Patterns: Common Misunderstandings and Traps. For more information, please follow other related articles on the PHP Chinese website!
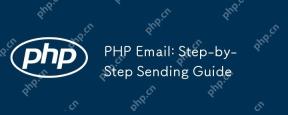
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
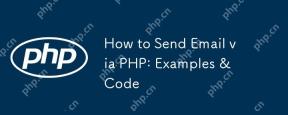
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
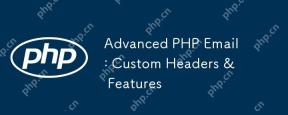
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
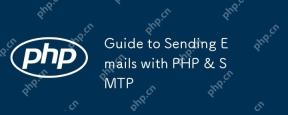
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
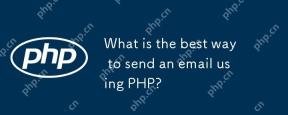
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
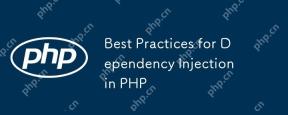
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
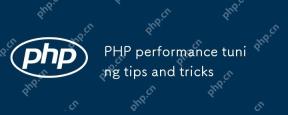
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
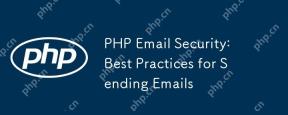
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version
