


What are the best practices for implementing fault-tolerant code in C++ using exception handling?
Best practices for implementing fault-tolerant code using exception handling in C++ include using custom exception types for specific error handling. An exception is thrown only if the error cannot be recovered. Use a constant variable to save the error message. Follow exception safety principles to ensure resource cleanup. Handle unknown exceptions, but use caution to avoid masking serious problems.
Best practices for implementing fault-tolerant code in C++ using exception handling
Exception handling is a method that separates error handling tasks from regular A powerful mechanism for separation in code flow. In C++, exceptions can be handled using the try-catch
statement.
Best Practices:
-
Use appropriate exception types: Create custom exception classes for specific error types instead of dependencies For the general
std::exception
. - Throw exceptions only when necessary: Throw exceptions only when the code cannot recover from the error. For errors that can be easily handled, error codes or return codes are more appropriate.
-
Use a
const
variable to save the error message: The error message should be static so that it does not change accidentally during exception propagation. - Follow the exception safety principle: Ensure that functions that throw exceptions can safely clean up resources at any time.
-
Handling unknown exceptions: Use the
catch(...)
statement to handle any exception type that is not specifically handled. However, it should be used with caution as it can mask potentially serious problems.
Practical case:
Suppose we have a function processFile()
, which is used to read files and perform some processing. We can use exception handling to handle potential errors such as the file does not exist or cannot be read:
#include <iostream> #include <fstream> #include <stdexcept> using namespace std; struct FileReadError : runtime_error { FileReadError(const string& msg) : runtime_error(msg) {} }; void processFile(const string& filename) { ifstream file(filename); if (!file.is_open()) { throw FileReadError("File not found or cannot be opened."); } // 在此处处理文件内容 file.close(); } int main() { try { processFile("input.txt"); } catch (const FileReadError& e) { cout << "File read error: " << e.what() << endl; } catch (const exception& e) { cout << "Unknown exception occurred: " << e.what() << endl; } return 0; }
In this example:
-
FileReadError
is a custom Exception type for errors specific to reading files. -
processFile()
The function throws aFileReadError
exception when the file cannot be opened. The -
main()
function uses thetry-catch
statement to handleFileReadError
and other exceptions that may occur.
The above is the detailed content of What are the best practices for implementing fault-tolerant code in C++ using exception handling?. For more information, please follow other related articles on the PHP Chinese website!
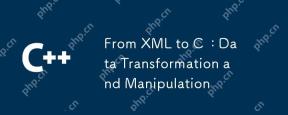
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
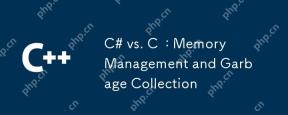
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
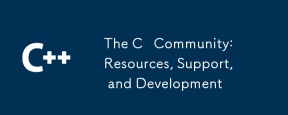
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
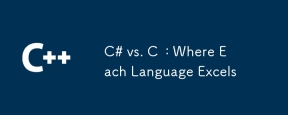
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
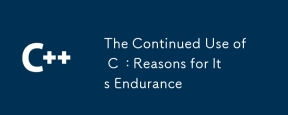
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
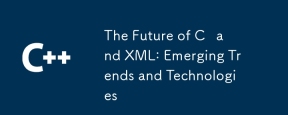
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
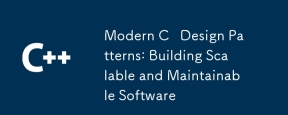
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),