How to use bufio to read JSON data from io.Reader in Go? Use bufio.NewReader to create a buffered io.Reader. Use bufio.ReadBytes to read JSON until a delimiter (usually a newline character) is encountered. Use the encoding/json package to parse JSON data.
How to use bufio in Golang to read JSON data from io.Reader
Reading JSON data is in Golang Common tasks. To read JSON from io.Reader
you can use the bufio
package. This is a simple tutorial demonstrating how to read JSON data from io.Reader
using bufio
.
Install bufio package
import "bufio"
Create io.Reader
To read JSON from io.Reader
, you need to create a io.Reader
first. You can use os.Stdin
to use standard input or create a file to read from.
Use bufio.NewReader to create a buffered io.Readerbufio
The package provides the NewReader
function, which can create a buffered io.Reader. Buffered io.Reader
. This can improve read performance on small files or network connections.
reader := bufio.NewReader(in)
Read JSON using bufio.ReadBytesbufio
package provides a function named ReadBytes
, which can be read from io.Reader
reads until a delimiter is encountered. For JSON data, the delimiter is usually the newline character ('\n').
line, err := reader.ReadBytes('\n') if err != nil { // 处理错误 }
Parsing JSON
After reading the JSON lines, you can use the encoding/json
package to parse the JSON data.
var data map[string]interface{} err = json.Unmarshal(line, &data) if err != nil { // 处理错误 }
Practical case
The following is a complete example of how to use bufio
to read JSON data from io.Reader
.
import ( "bufio" "encoding/json" "fmt" "os" ) func main() { // 使用标准输入创建 io.Reader reader := bufio.NewReader(os.Stdin) // 读取 JSON 数据直到遇到换行符 line, err := reader.ReadBytes('\n') if err != nil { fmt.Println("Error reading JSON:", err) os.Exit(1) } // 解析 JSON 数据 var data map[string]interface{} err = json.Unmarshal(line, &data) if err != nil { fmt.Println("Error parsing JSON:", err) os.Exit(1) } // 打印数据 fmt.Println(data) }
The above is the detailed content of How to read JSON data from io.Reader using bufio in Golang?. For more information, please follow other related articles on the PHP Chinese website!
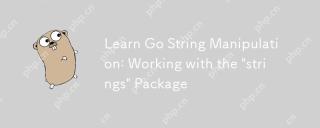
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
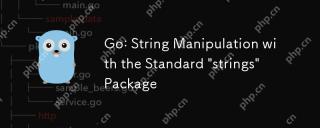
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
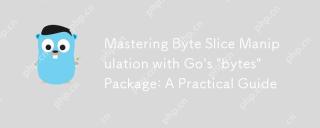
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
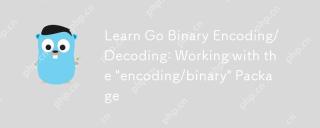
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
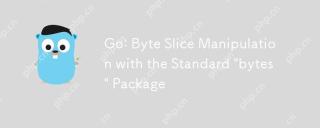
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
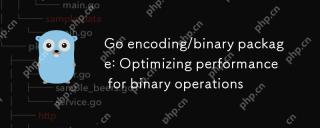
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
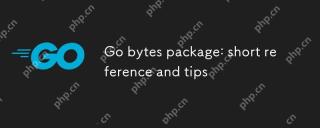
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
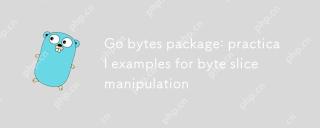
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version
