Best practice: Choose the right container: Choose the appropriate container according to the element access mode, such as vector, list, map. Capacity management: Pre-allocate container space (such as using reserve()) to improve insertion/deletion efficiency. Range accessors: Use begin()/end() to return an iterator for concise access to elements (such as a for loop). Practical case: For example, use a vector to store a large number of grades, pre-allocate space and use range accessor traversal to calculate the average grade.
C++ Container Library Best Practices Guide
Preface
C++ Container Library It is a powerful collection of data structures that can be used to efficiently manage and process data. However, improper use can lead to performance issues and code errors. This article provides guidance on C++ container library best practices to help you optimize your code and avoid common pitfalls.
Choose the right container
The container library provides various containers such as vectors, lists, and maps. It is important to choose the appropriate container based on data type and access pattern. The following are recommended containers for common situations:
- Frequently add or remove elements: Vector (vector)
- Need to find elements quickly: Map ( map) or unordered_map
- Need to preserve the insertion order of elements: List(list)
- Need to access the starting and ending points of elements: Double-ended queue (deque)
Capacity management
Allocating too little capacity will trigger frequent reallocation, resulting in poor performance . Pre-allocating enough capacity improves the efficiency of insert and delete operations. Use the reserve()
method to reserve space for a vector, for example:
std::vector<int> vec; vec.reserve(1000);
Range accessor
The range accessor provides a concise method to access the container element. Use the begin()
and end()
functions to return iterators, for example:
std::vector<int> vec = {1, 2, 3, 4, 5}; for (auto it = vec.begin(); it != vec.end(); ++it) { std::cout << *it << " "; }
Practical case
Consider using The vector stores the scores of a large number of students. You can use reserve()
to preallocate space and use range accessors to iterate over grades:
#include <vector> int main() { std::vector<int> grades; grades.reserve(1000); // 添加成绩 for (int i = 0; i < 1000; i++) { grades.push_back(rand() % 100); } // 计算平均成绩 int sum = 0; for (auto grade : grades) { sum += grade; } double average = static_cast<double>(sum) / grades.size(); std::cout << "平均成绩为:" << average << std::endl; return 0; }
Conclusion
By following these best practices, you C++ container libraries can be used more efficiently. Choosing your containers wisely, managing capacity, and using range accessors can significantly improve the performance of your code, reduce errors, and enhance maintainability.
The above is the detailed content of C++ Container Library Best Practices Guide. For more information, please follow other related articles on the PHP Chinese website!
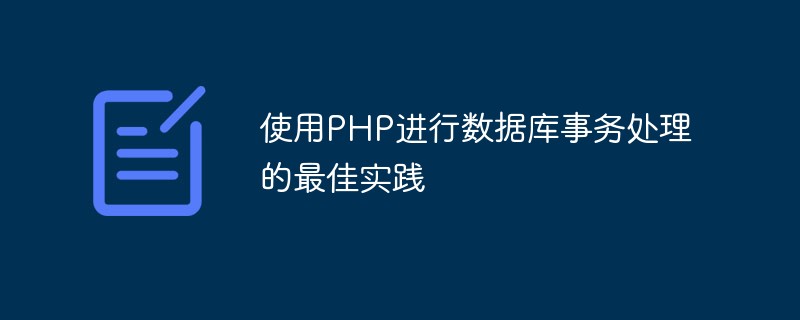
在Web开发中,数据库事务处理是一个重要的问题。当程序需要操作多个数据库表格时,保证数据一致性和完整性变得尤为重要。事务处理提供了一种方法来保证这些操作要么全部成功,要么全部失败。PHP作为一门流行的Web开发语言,也提供了事务处理的功能。本文将介绍使用PHP进行数据库事务处理的最佳实践。什么是数据库事务?在数据库中,事务是指一系列操作作为一个整体来执行的过
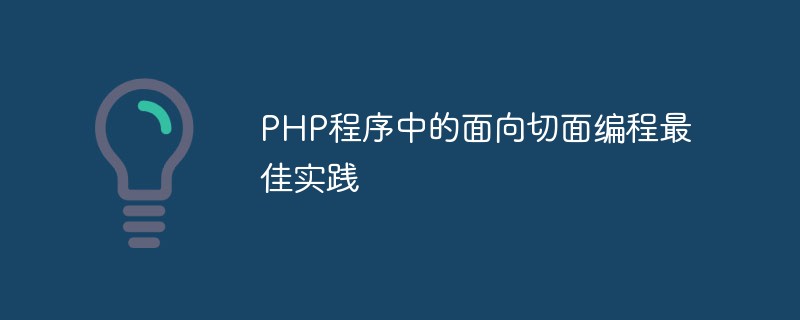
随着互联网技术的不断发展,PHP语言作为一种开源的脚本编程语言在Web应用程序开发中广受欢迎,而面向切面编程(AOP)则是PHP程序员日常工作中的重要组成部分之一。AOP是一种程序设计方法,它在主业务逻辑代码执行过程中插入针对横切关注点的代码,这些代码可能涉及到日志记录、异常处理、缓存控制等方面。在本文中,我们将介绍PHP程序中的AOP最佳实践。一、AOP的
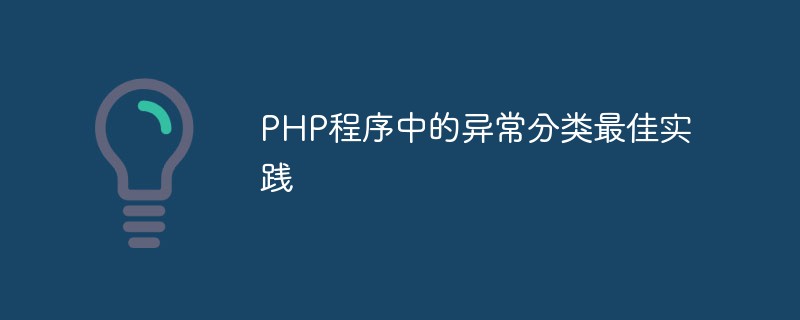
在编写PHP代码时,异常处理是不可或缺的一部分,它可以使代码更加健壮和可维护。但是,异常处理也需要谨慎使用,否则就可能带来更多的问题。在这篇文章中,我将分享一些PHP程序中异常分类的最佳实践,以帮助你更好地利用异常处理来提高代码质量。异常的概念在PHP中,异常是指在程序运行时发生的错误或意外情况。通常情况下,异常会导致程序停止运行并输出异常信息。
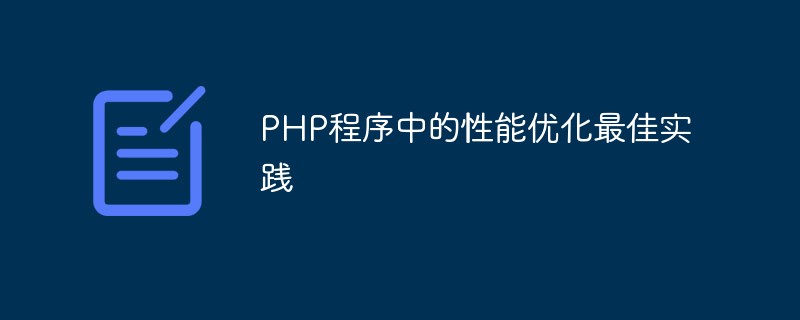
PHP是一种流行的编程语言,被广泛用于网站和Web应用程序的开发。然而,当PHP应用程序变得越来越复杂时,性能问题也会显现出来。因此,性能优化成为了PHP开发中的一个重要方面。在本文中,我们将介绍PHP程序中的优化最佳实践,以帮助你提高应用程序的性能。1.选择正确的PHP版本和扩展首先,确保你是使用最新的PHP版本。新版本通常会改进性能并修复bug,同时也会
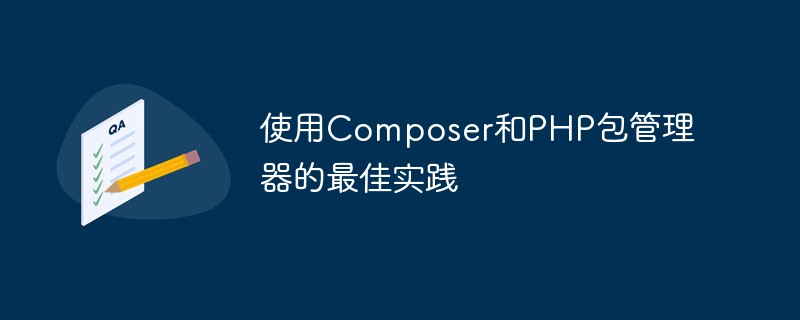
随着PHP的日益流行,PHP开发人员面临着许多挑战,其中包括代码管理、可重用性和依赖性管理。这些问题可以使用包管理器来解决,而Composer是PHP最受欢迎的包管理器之一。在本文中,我们将探讨使用Composer和PHP包管理器的最佳实践,从而提高您的PHP开发效率和代码质量。何为Composer?Composer是一款PHP包管理器,它可以轻松管理PHP
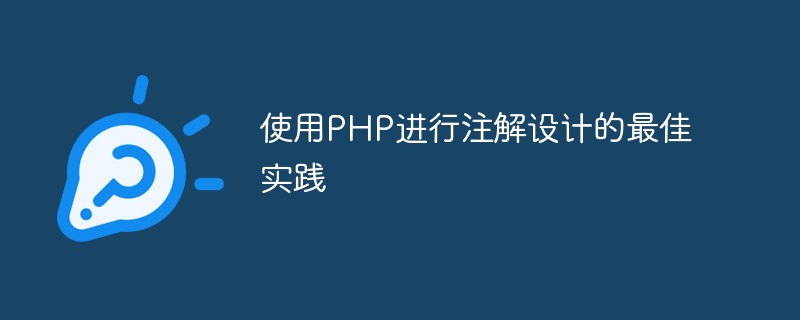
随着Web应用程序的不断发展,代码越来越复杂,开发人员需要能够更好地组织和管理代码。注解设计是一种使代码更加可读、可维护和可扩展的有效方法。PHP是一种强大的编程语言,而且支持注解。在这篇文章中,我们将介绍使用PHP进行注解设计的最佳实践。什么是注解?注解是将元数据添加到源代码中的一种方法。它们提供了对类、方法、属性等的额外信息,这些信息可以被其他程序或框架
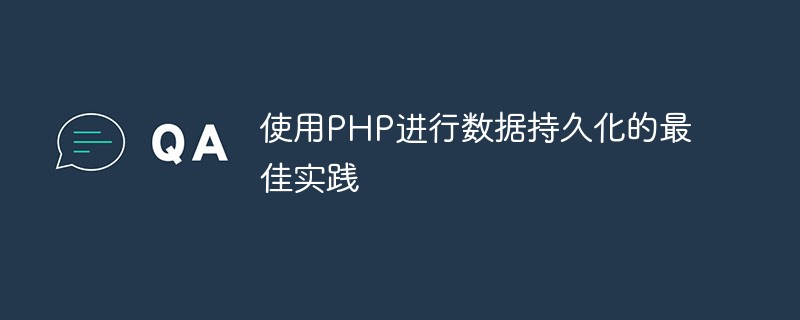
PHP是一种广泛应用于Web开发的编程语言,其强大的数据持久化功能使得PHP成为了许多项目的首选语言之一。在PHP中,数据持久化是一个重要的话题,因为它涉及到存储和检索数据的方法。在本文中,我们将介绍一些使用PHP进行数据持久化的最佳实践。使用数据库管理系统使用数据库管理系统(DBMS)是进行数据持久化的最常见方法之一。PHP中有一些成熟的数据库管理系统可以
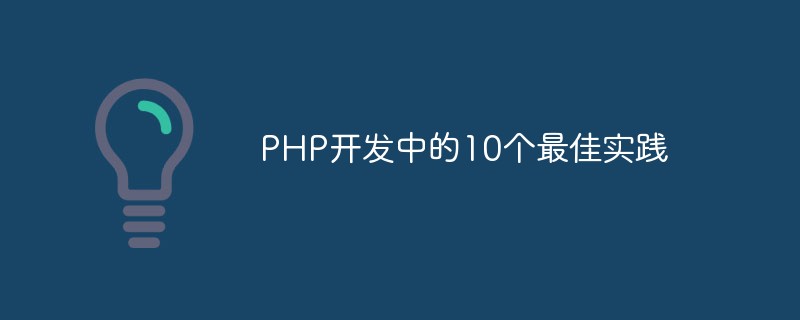
PHP是一种广泛使用的开源脚本语言,特别适用于Web开发领域。与许多其他编程语言相比,PHP的学习曲线较为平滑,但是为了生产高质量、可维护的代码,遵守最佳实践是非常重要的。下面是PHP开发中的10个最佳实践。使用命名空间在开发PHP应用程序时,避免全局名称冲突是非常重要的。使用命名空间是一个非常好的办法,可以将代码包装在一个逻辑上的包中,从而使之与其他代码分


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
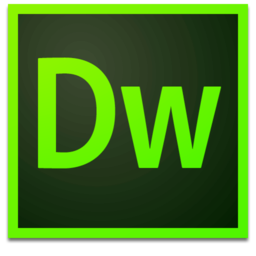
Dreamweaver Mac version
Visual web development tools
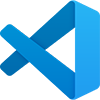
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
