Methods to implement multi-threading in the C++ standard library: include header files: #include <thread> create thread objects: std::thread t(function_or_lambda) start threads: t.start() wait for threads to complete: t.join ()
Use the C++ standard library to implement multi-threading
Multi-threading refers to executing multiple different tasks at the same time in a program. This is for improving Program concurrency and responsiveness are very important concepts. The C++ standard library provides several classes and functions that make multithreading easy.
Here's how to implement multithreading using the C++ standard library:
-
Include header files:
#include <thread>
-
Create a thread object:
Use thestd::thread
class to create a thread object and specify the function or callable object to be executed.std::thread t(function_or_lambda);
-
Start the thread:
Use thestd::thread::start()
method to start the thread. This method will start a new thread and execute the specified function or callable object.t.start();
-
Wait for a thread to complete:
Use thestd::thread::join()
method to wait for a thread to complete its task.t.join();
Practical case:
Calculate the sum of multiple numbers:
Use multi-threading to calculate the sum of a set of numbers sum. Split the array into multiple subarrays and create multiple threads to calculate the sum of each subarray simultaneously. Finally, the sum of the subarrays calculated by each thread is added to obtain the total.
#include <thread> #includeusing namespace std; vector numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; int num_threads = 4; int sum(const vector & arr, int start, int end) { int sum = 0; for (int i = start; i < end; i++) { sum += arr[i]; } return sum; } int main() { // 创建线程数组 vector threads(num_threads); // 计算每个线程负责的范围 int chunk_size = numbers.size() / num_threads; vector partial_sums(num_threads); for (int i = 0; i < num_threads; i++) { int start = i * chunk_size; int end = min((i + 1) * chunk_size, numbers.size()); // 创建线程并计算部分和 threads[i] = thread(sum, ref(numbers), start, end); } // 等待所有线程完成 for (int i = 0; i < num_threads; i++) { threads[i].join(); } // 计算总体和 int total_sum = 0; for (int partial_sum : partial_sums) { total_sum += partial_sum; } cout << "Total sum: " << total_sum << endl; return 0; }
The above is the detailed content of How to implement multithreading using the C++ standard library?. For more information, please follow other related articles on the PHP Chinese website!
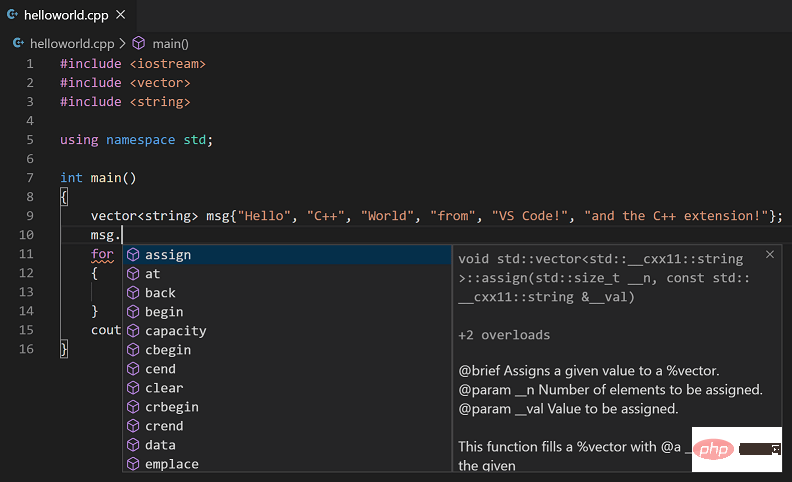
C++是一种广泛使用的面向对象的计算机编程语言,它支持您与之交互的大多数应用程序和网站。你需要编译器和集成开发环境来开发C++应用程序,既然你在这里,我猜你正在寻找一个。我们将在本文中介绍一些适用于Windows11的C++编译器的主要推荐。许多审查的编译器将主要用于C++,但也有许多通用编译器您可能想尝试。MinGW可以在Windows11上运行吗?在本文中,我们没有将MinGW作为独立编译器进行讨论,但如果讨论了某些IDE中的功能,并且是DevC++编译器的首选
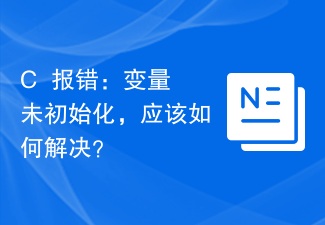
在C++程序开发中,当我们声明了一个变量但是没有对其进行初始化,就会出现“变量未初始化”的报错。这种报错经常会让人感到很困惑和无从下手,因为这种错误并不像其他常见的语法错误那样具体,也不会给出特定的代码行数或者错误类型。因此,下面我们将详细介绍变量未初始化的问题,以及如何解决这个报错。一、什么是变量未初始化错误?变量未初始化是指在程序中声明了一个变量但是没有
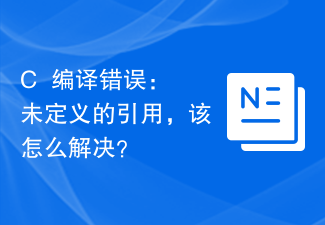
C++是一门广受欢迎的编程语言,但是在使用过程中,经常会出现“未定义的引用”这个编译错误,给程序的开发带来了诸多麻烦。本篇文章将从出错原因和解决方法两个方面,探讨“未定义的引用”错误的解决方法。一、出错原因C++编译器在编译一个源文件时,会将它分为两个阶段:编译阶段和链接阶段。编译阶段将源文件中的源码转换为汇编代码,而链接阶段将不同的源文件合并为一个可执行文
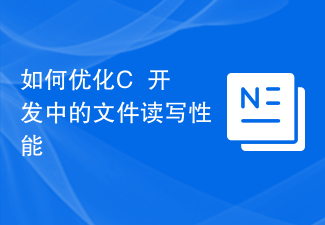
如何优化C++开发中的文件读写性能在C++开发过程中,文件的读写操作是常见的任务之一。然而,由于文件读写是磁盘IO操作,相对于内存IO操作来说会更为耗时。为了提高程序的性能,我们需要优化文件读写操作。本文将介绍一些常见的优化技巧和建议,帮助开发者在C++文件读写过程中提高性能。使用合适的文件读写方式在C++中,文件读写可以通过多种方式实现,如C风格的文件IO
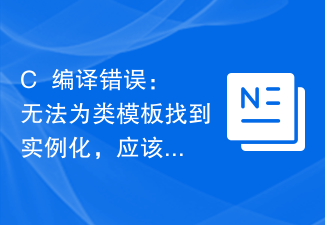
C++是一门强大的编程语言,它支持使用类模板来实现代码的复用,提高开发效率。但是在使用类模板时,可能会遭遇编译错误,其中一个比较常见的错误是“无法为类模板找到实例化”(error:cannotfindinstantiationofclasstemplate)。本文将介绍这个问题的原因以及如何解决。问题描述在使用类模板时,有时会遇到以下错误信息:e
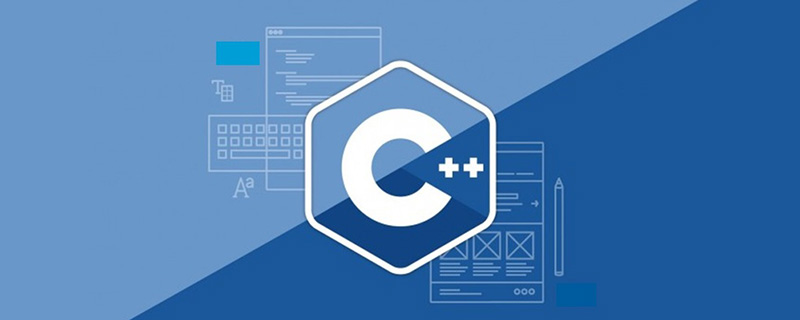
iostream头文件包含了操作输入输出流的方法,比如读取一个文件,以流的方式读取;其作用是:让初学者有一个方便的命令行输入输出试验环境。iostream的设计初衷是提供一个可扩展的类型安全的IO机制。
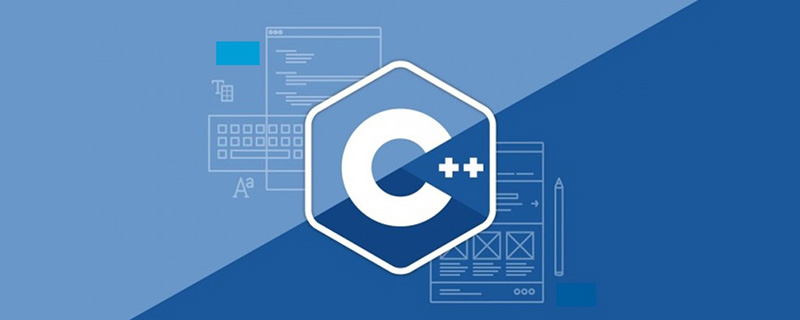
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。
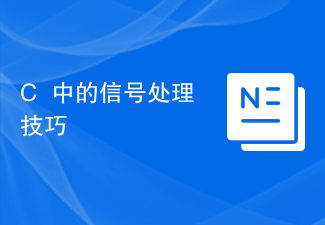
C++是一种流行的编程语言,它强大而灵活,适用于各种应用程序开发。在使用C++开发应用程序时,经常需要处理各种信号。本文将介绍C++中的信号处理技巧,以帮助开发人员更好地掌握这一方面。一、信号处理的基本概念信号是一种软件中断,用于通知应用程序内部或外部事件。当特定事件发生时,操作系统会向应用程序发送信号,应用程序可以选择忽略或响应此信号。在C++中,信号可以


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
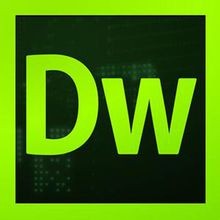
Dreamweaver CS6
Visual web development tools
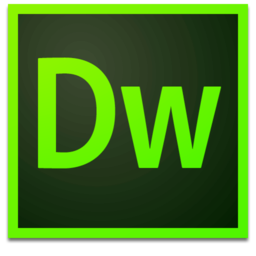
Dreamweaver Mac version
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment
