C++ Generic Programming: The Path to Code Readability
Generic programming refers to the use of type parameters in code, allowing writing to handle a variety of data Type functions and classes. It improves code readability and maintainability by abstracting common logic.
Template function
The parameters of the template function can be of any type and are instantiated at compile time. For example, we can use the swap
function to swap two values:
template<typename T> void swap(T& a, T& b) { T tmp = a; a = b; b = tmp; }
This function can be used for any type of data as follows:
int a = 1; int b = 2; swap(a, b); // a 现在为 2,b 现在为 1
Template class
Template classes can create objects that can store different types of data. For example, we can use the Vector
class to represent a variable array:
template<typename T> class Vector { private: T* data; int size; public: Vector() : size(0), data(nullptr) {} ~Vector() { delete[] data; } void push_back(const T& value) { ... } void pop_back() { ... } T& operator[](int index) { ... } };
This class can be used to store any type of data, as shown below:
Vector<int> numbers; numbers.push_back(1); numbers.push_back(2); Vector<std::string> names; names.push_back("Alice"); names.push_back("Bob");
Practical case
In actual development, generic programming is widely used in:
-
Data structure: Generic data structure (such as
std::vector
andstd::map
) allow different types of data to be processed in a unified manner. -
Algorithms: Generic algorithms (such as
std::sort
andstd::find
) can be applied to any type of data collection. -
Containers: Generic containers (such as
std::function
andstd::shared_ptr
) allow flexible storage and management of different types of data.
Advantages
Using generic programming can bring the following advantages:
- Readability: By abstracting common logic , generic code is easier to understand and maintain.
- Reusability: Generic components can be used to solve a wide range of problems and improve code reusability.
- Type safety: The compiler enforces type safety to ensure that no type errors occur in generic code.
The above is the detailed content of How does C++ generic programming help improve code readability?. For more information, please follow other related articles on the PHP Chinese website!

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
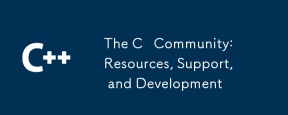
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
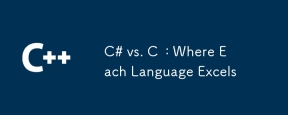
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
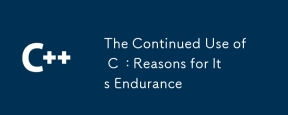
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
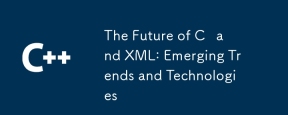
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
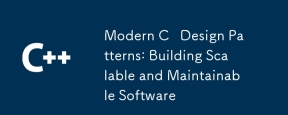
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
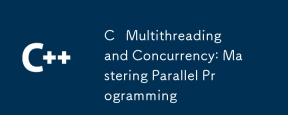
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
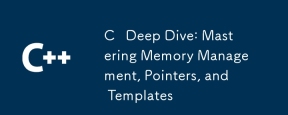
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
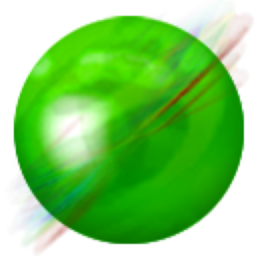
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
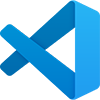
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
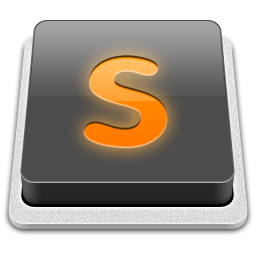
SublimeText3 Mac version
God-level code editing software (SublimeText3)
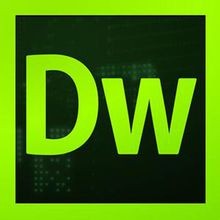
Dreamweaver CS6
Visual web development tools