Debugging tips for C++ multi-threaded programming include using a data race analyzer to detect read and write conflicts and using synchronization mechanisms (such as mutex locks) to resolve them. Use thread debugging tools to detect deadlocks and resolve them by avoiding nested locks and using deadlock detection mechanisms. Use the Data Race Analyzer to detect data races and resolve them by moving write operations into critical sections or using atomic operations. Use performance analysis tools to measure context switch frequency and resolve excessive overhead by reducing the number of threads, using thread pools, and offloading tasks.
Debugging and Troubleshooting Tips in C++ Multithreaded Programming
Multi-threaded programming can play an important role in improving application performance and responsiveness, but It also introduces new debugging and troubleshooting challenges. This article introduces common multi-threading problems in C++ and their resolution techniques, and provides real cases to illustrate.
Read-write conflicts
Read-write conflicts occur when multiple threads access shared memory at the same time, and one thread tries to write while the other threads try to read. This can lead to data corruption and undefined behavior.
Detection:
Use a data race analyzer (such as Valgrind's tsan tool) or define a global variable to track the number of read and write operations.
Solution:
Use synchronization mechanisms, such as mutex locks or read-write locks, to control access to shared resources.
Deadlock
Deadlock occurs when two or more threads are waiting for each other's lock. This causes the application to freeze without any progress.
Detection:
Use graphical thread debugging tools (such as Visual Studio's Parallel Task Window) to visualize the status of threads.
Solution:
Avoid nested locks and use deadlock detection and recovery mechanisms.
Data race
Data race is similar to read and write conflicts, but it occurs when multiple threads write to shared memory at the same time. This can lead to unpredictable data corruption.
Detection:
Use a data race analyzer or write a custom check to ensure that shared variables are only written to in one thread.
Solution:
Move the write operation to the critical section or use atomic operations.
Context switch overhead
Context switching is the overhead that occurs when a thread switches from one processor core to another. Excessive context switching can cause application performance degradation.
Detection:
Measure the frequency of context switches using a performance profiling tool such as perf or gprof.
Solution:
Reduce the number of threads, use thread pools, and offload computationally intensive tasks to other processor cores whenever possible.
Practical case:
Suppose there is a multi-threaded application in which multiple threads update a linked list in parallel. Without proper synchronization, read and write conflicts and data corruption can result. You can use a mutex lock to protect modifications to a linked list, as follows:
std::mutex list_mutex; void update_list(int value) { std::lock_guard<std::mutex> lock(list_mutex); // 对链表进行修改... }
Developing and maintaining C++ multithreaded applications can be greatly simplified by following these debugging and troubleshooting tips.
The above is the detailed content of Debugging and Troubleshooting Techniques in C++ Multithreaded Programming. For more information, please follow other related articles on the PHP Chinese website!
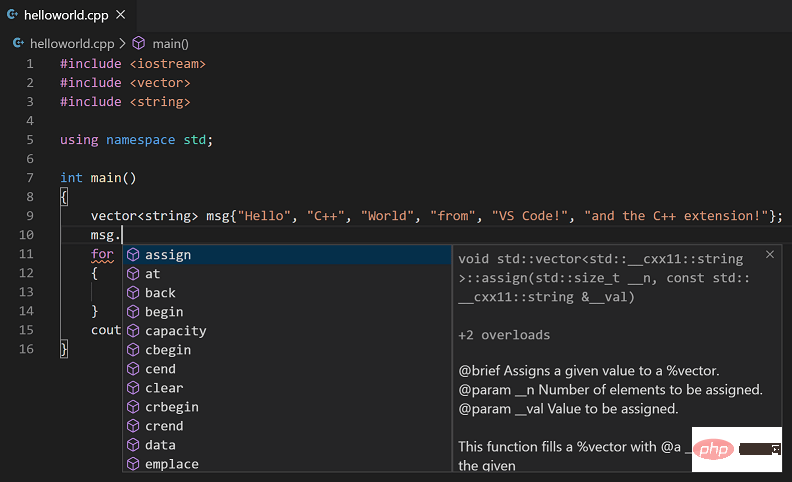
C++是一种广泛使用的面向对象的计算机编程语言,它支持您与之交互的大多数应用程序和网站。你需要编译器和集成开发环境来开发C++应用程序,既然你在这里,我猜你正在寻找一个。我们将在本文中介绍一些适用于Windows11的C++编译器的主要推荐。许多审查的编译器将主要用于C++,但也有许多通用编译器您可能想尝试。MinGW可以在Windows11上运行吗?在本文中,我们没有将MinGW作为独立编译器进行讨论,但如果讨论了某些IDE中的功能,并且是DevC++编译器的首选
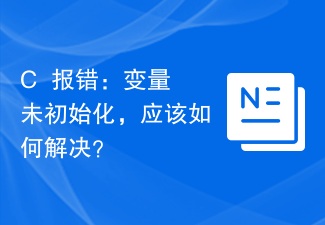
在C++程序开发中,当我们声明了一个变量但是没有对其进行初始化,就会出现“变量未初始化”的报错。这种报错经常会让人感到很困惑和无从下手,因为这种错误并不像其他常见的语法错误那样具体,也不会给出特定的代码行数或者错误类型。因此,下面我们将详细介绍变量未初始化的问题,以及如何解决这个报错。一、什么是变量未初始化错误?变量未初始化是指在程序中声明了一个变量但是没有
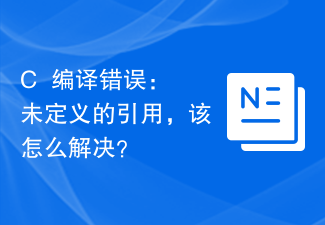
C++是一门广受欢迎的编程语言,但是在使用过程中,经常会出现“未定义的引用”这个编译错误,给程序的开发带来了诸多麻烦。本篇文章将从出错原因和解决方法两个方面,探讨“未定义的引用”错误的解决方法。一、出错原因C++编译器在编译一个源文件时,会将它分为两个阶段:编译阶段和链接阶段。编译阶段将源文件中的源码转换为汇编代码,而链接阶段将不同的源文件合并为一个可执行文
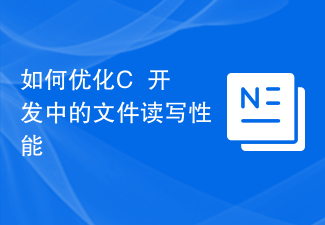
如何优化C++开发中的文件读写性能在C++开发过程中,文件的读写操作是常见的任务之一。然而,由于文件读写是磁盘IO操作,相对于内存IO操作来说会更为耗时。为了提高程序的性能,我们需要优化文件读写操作。本文将介绍一些常见的优化技巧和建议,帮助开发者在C++文件读写过程中提高性能。使用合适的文件读写方式在C++中,文件读写可以通过多种方式实现,如C风格的文件IO
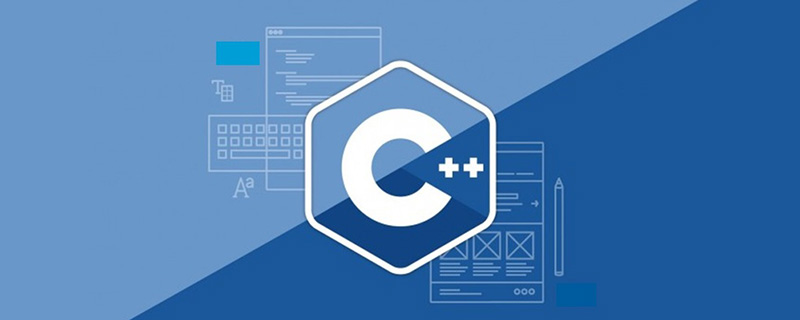
iostream头文件包含了操作输入输出流的方法,比如读取一个文件,以流的方式读取;其作用是:让初学者有一个方便的命令行输入输出试验环境。iostream的设计初衷是提供一个可扩展的类型安全的IO机制。
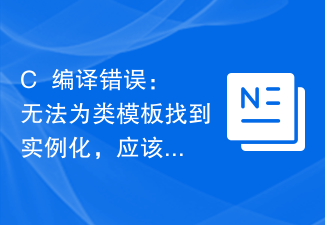
C++是一门强大的编程语言,它支持使用类模板来实现代码的复用,提高开发效率。但是在使用类模板时,可能会遭遇编译错误,其中一个比较常见的错误是“无法为类模板找到实例化”(error:cannotfindinstantiationofclasstemplate)。本文将介绍这个问题的原因以及如何解决。问题描述在使用类模板时,有时会遇到以下错误信息:e
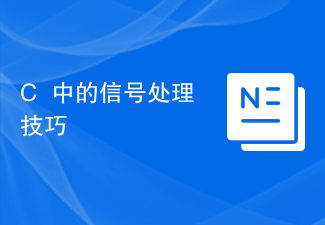
C++是一种流行的编程语言,它强大而灵活,适用于各种应用程序开发。在使用C++开发应用程序时,经常需要处理各种信号。本文将介绍C++中的信号处理技巧,以帮助开发人员更好地掌握这一方面。一、信号处理的基本概念信号是一种软件中断,用于通知应用程序内部或外部事件。当特定事件发生时,操作系统会向应用程序发送信号,应用程序可以选择忽略或响应此信号。在C++中,信号可以
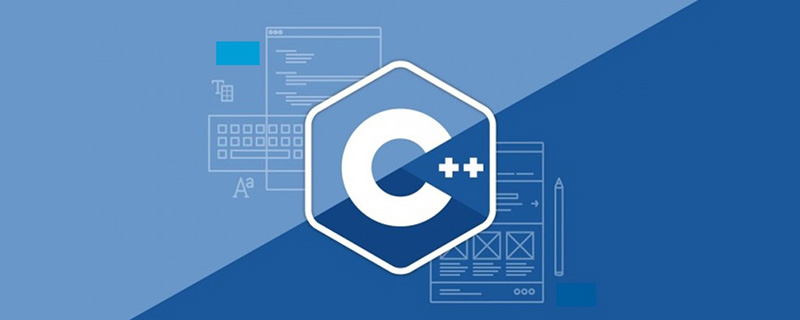
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
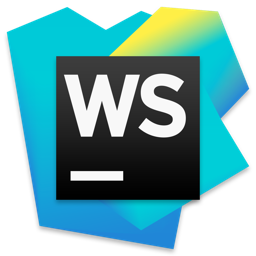
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
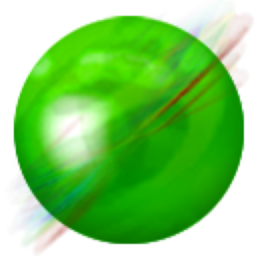
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
