In Golang, query results can be sorted by using the ORDER BY clause in the database/sql package. Syntax: func (db *DB) Query(query string, args ...interface{}) (*Rows, error) Sorting example: SELECT * FROM users ORDER BY name ASC Other sorting options: DESC (descending), multiple columns (Comma separated), NULL value sort order (NULLS FIRST or NULLS LAST) Practical case: Sort orders in descending order by "order_date": SELECT * FROM orders ORDER BY order_date DESC.
How to sort database records in Golang?
When using the database/sql package to operate the database in Golang, you can use the ORDER BY
clause to sort the query results.
Syntax:
func (db *DB) Query(query string, args ...interface{}) (*Rows, error)
Sort example:
The following example shows how to sort the Records sorted by "name" column in ascending order:
package main import ( "database/sql" "fmt" _ "github.com/go-sql-driver/mysql" ) func main() { // 连接到数据库 db, err := sql.Open("mysql", "user:password@tcp(127.0.0.1:3306)/database") if err != nil { panic(err) } defer db.Close() // 构建查询 query := `SELECT * FROM users ORDER BY name ASC` // 执行查询 rows, err := db.Query(query) if err != nil { panic(err) } defer rows.Close() // 遍历结果 for rows.Next() { var id int var name, email string if err := rows.Scan(&id, &name, &email); err != nil { panic(err) } fmt.Printf("%d %s %s\n", id, name, email) } }
Other sorting options:
- DESC: Sort in descending order
-
Multiple columns: Use commas to separate multiple columns, for example:
ORDER BY name DESC, age ASC
-
NULL value: Use
NULLS FIRST
orNULLS LAST
Specifies whether to place NULL values at the beginning or end of the result set
Practical case:
Suppose we have an "orders" table with columns "order_id", "customer_id" and "order_date". We can write a Golang program to sort orders by "order_date" in descending order:
package main import ( "database/sql" "fmt" _ "github.com/go-sql-driver/mysql" ) func main() { // 连接到数据库 db, err := sql.Open("mysql", "user:password@tcp(127.0.0.1:3306)/database") if err != nil { panic(err) } defer db.Close() // 构建查询 query := `SELECT * FROM orders ORDER BY order_date DESC` // 执行查询 rows, err := db.Query(query) if err != nil { panic(err) } defer rows.Close() // 遍历结果 for rows.Next() { var orderID, customerID int var orderDate string if err := rows.Scan(&orderID, &customerID, &orderDate); err != nil { panic(err) } fmt.Printf("%d %d %s\n", orderID, customerID, orderDate) } }
The above is the detailed content of How to sort database records in Golang?. For more information, please follow other related articles on the PHP Chinese website!
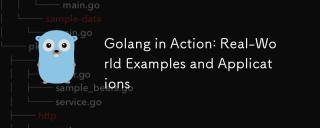
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
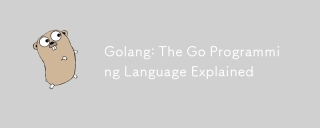
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
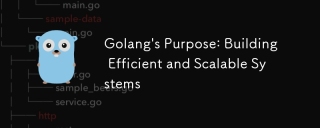
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
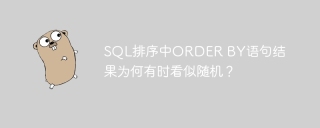
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
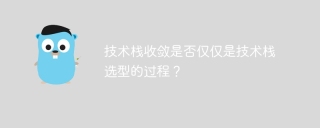
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
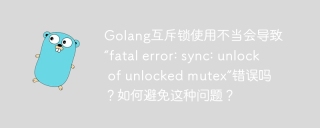
Golang ...
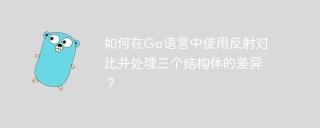
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...
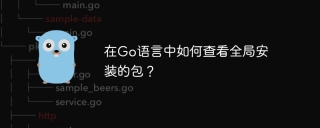
How to view globally installed packages in Go? In the process of developing with Go language, go often uses...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),