Key strategies for optimizing Java framework performance include: using caching to retrieve data quickly. Use a thread pool to manage the creation and reuse of threads. Optimize database schemas, use indexes and query caching to improve query speed. Optimize HTTP requests and responses to reduce page load times. Use code analysis tools to identify inefficient code and fix it.
Practical Guide to Java Framework Performance Optimization
In modern web development, choosing the right framework is crucial to the performance of the application important. However, even if you choose an efficient framework, it is crucial to optimize performance to provide the best user experience.
This article will explore key strategies for Java framework performance optimization and provide practical code examples to help you turn theory into practice.
1. Caching
Cache is a mechanism for storing data for quick retrieval. By storing frequently accessed data in cache, you avoid expensive database queries or file system calls.
Practical case:
// 使用 Caffeine 缓存器创建缓存 Cache<String, Object> cache = Caffeine.newBuilder() .expireAfterWrite(10, TimeUnit.MINUTES) .maximumSize(100) .build(); // 将对象放入缓存 cache.put("my-key", myObject); // 从缓存获取对象 Object cachedObject = cache.getIfPresent("my-key");
2. Thread pool
The thread pool manages the creation and reuse of threads. This helps avoid the overhead of thread creation and prevents the creation of too many threads from straining system resources.
Practical case:
// 使用 ThreadPoolExecutor 创建线程池 ExecutorService executorService = new ThreadPoolExecutor( 5, // 核心线程数 10, // 最大线程数 100, // 线程空闲时间 TimeUnit.MILLISECONDS, new LinkedBlockingQueue<>() // 任务队列 ); // 提交任务到线程池 executorService.submit(new MyTask());
3. Database optimization
Database performance plays a role in the overall performance of Java applications Crucial role. By optimizing your database schema, using indexes, and query caching, you can significantly increase the speed of database queries.
Practical case:
// 创建一个带索引的表 Connection connection = ...; Statement statement = connection.createStatement(); statement.executeUpdate( "CREATE TABLE users (id INT NOT NULL, name VARCHAR(255) NOT NULL, PRIMARY KEY (id), INDEX (name))" );
4. HTTP optimization
Optimizing your application’s HTTP requests and responses can improve Page load time and user experience. This can be achieved using compression, enabling HTTPS, and minimizing redirects.
Practical case:
// 启用 HTTP 压缩 response.addHeader("Content-Encoding", "gzip"); response.getOutputStream().write(GZIPOutputStream.wrap(response.getOutputStream()).getBytes()); // 启用 HTTPS ServerConnector serverConnector = new ServerConnector(...); serverConnector.addSslContext(getSslContext());
5. Code analysis
Code analysis tools can help you identify performance in your application Bottlenecks and inefficient code. By using these tools, you can identify and fix problems in your code, thereby improving the overall performance of your application.
Practical Examples:
// 使用 OpenJDK Flight Recorder 分析代码 Recording recording = FlightRecorder.getFlightRecorder() .newRecording(); recording.start(); // 运行一段代码 ... recording.stop(); // 分析记录器数据 ObjectAnalyzer objectAnalyzer = new ObjectAnalyzer(); objectAnalyzer.analyze(recording);
By following these strategies and implementing the provided practical examples, you can significantly optimize the performance of your Java framework and provide a smooth and efficient user experience.
The above is the detailed content of Practical Guide to Java Framework Performance Optimization. For more information, please follow other related articles on the PHP Chinese website!
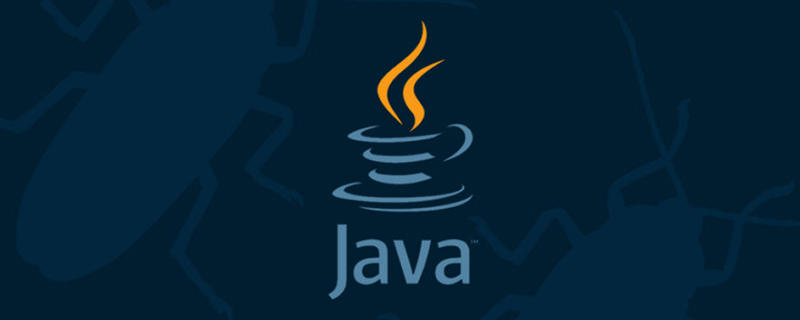
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
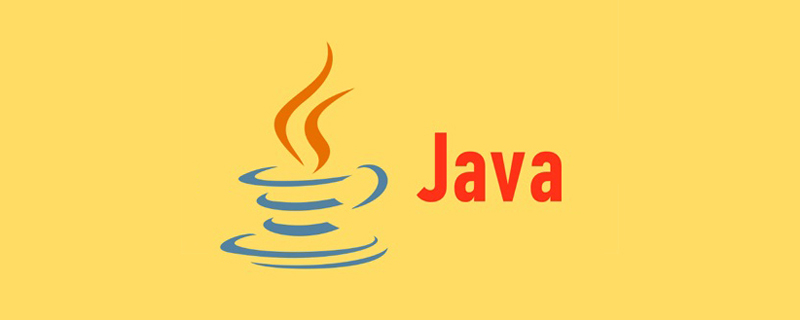
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
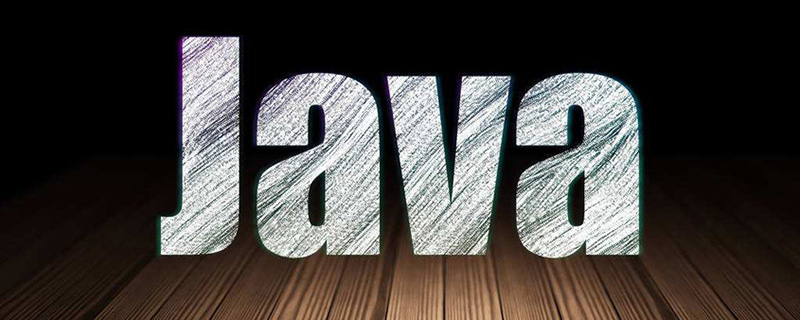
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
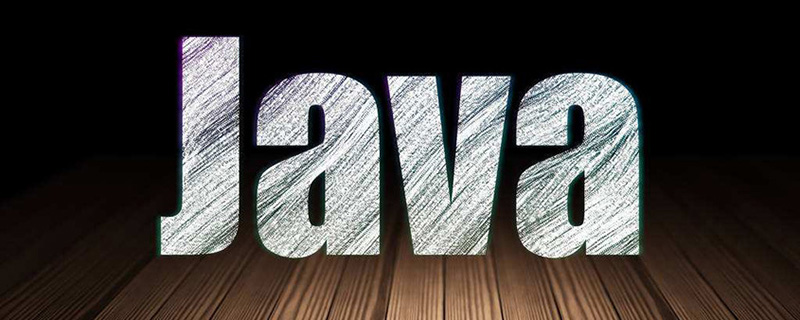
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
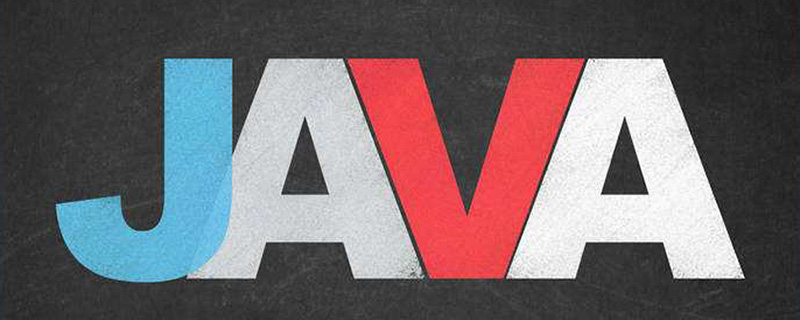
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
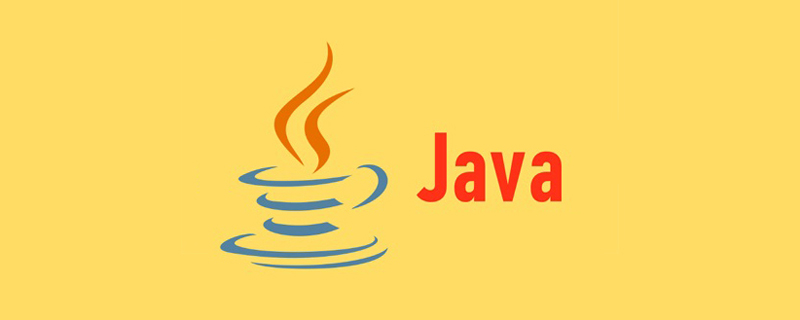
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
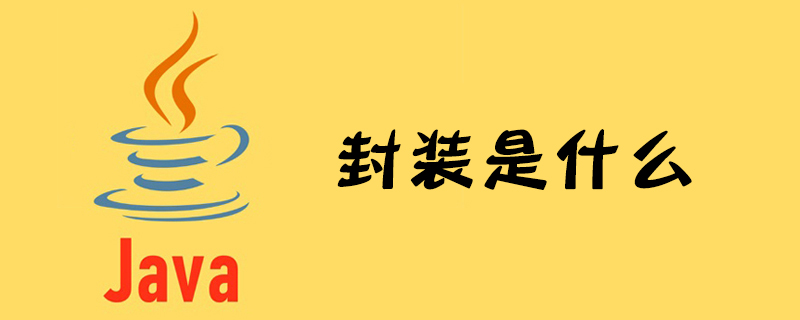
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
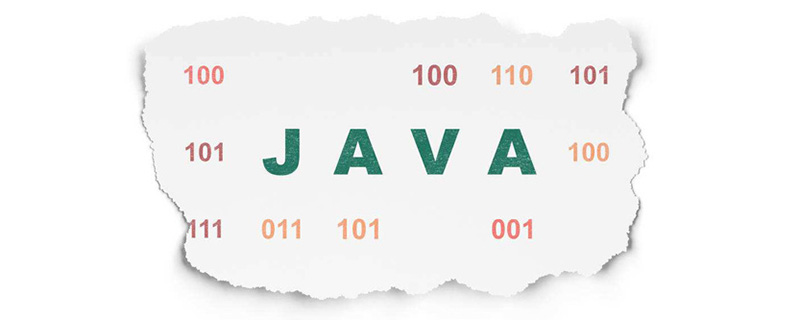
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于设计模式的相关问题,主要将装饰器模式的相关内容,指在不改变现有对象结构的情况下,动态地给该对象增加一些职责的模式,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
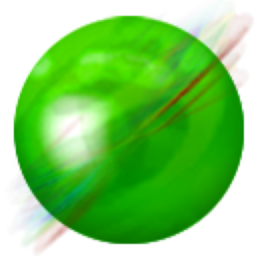
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment
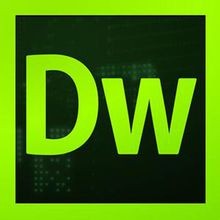
Dreamweaver CS6
Visual web development tools
