C Performance considerations for generic programming: Avoid over-segmentation: Generic algorithms may be instantiated multiple times, resulting in code segmentation and performance degradation. Avoid virtual calls: Generic classes or methods may generate virtual calls, thereby reducing performance. Consider specialization: For common types, creating type-specific implementations can avoid over-segmentation and virtual calls and improve performance.
Performance Considerations in Generic Programming in C
Generic programming is a powerful tool in C that allows us Write code that works with a variety of data types. However, when programming with generics, it's critical to understand their potential performance impact.
1. Avoid over-segmentation:
Generic algorithms may be instantiated multiple times depending on the type, resulting in code segmentation and performance degradation. Consider the following example:
template <typename T> void swap(T& a, T& b) { T temp = a; a = b; b = temp; }
To exchange a value of type int
, this function would be instantiated as swap<int></int>
. Likewise, for a value of type double
, it will be instantiated as swap<double></double>
. This over-partitioning increases binary file size and execution time.
2. Avoid virtual calls:
Generic classes or methods may cause virtual calls, further reducing performance. For example:
class Base { public: virtual void doSomething(); }; template <typename T> class Derived : public Base { public: void doSomething() override { // 重写 doSomething() 方法 } };
Since doSomething()
is a virtual method, each Derived<t></t>
object will resolve to the correct implementation at runtime. This introduces an extra layer of indirection, hurting performance.
3. Consider specializations:
For some common types, such as int
, double
and bool
, we can avoid over-segmentation and virtual calls by creating type-specific implementations. This is called specialization:
template <> void swap<int>(int& a, int& b) { // 对 int 类型进行特殊处理 }
Specialization can significantly improve performance because it eliminates multiple instantiations and virtual calls.
Practical case:
Suppose we have a Vector
class that uses generic programming to store different types of data:
template <typename T> class Vector { public: Vector(size_t size); ~Vector(); // ... };
If we frequently swap the positions of elements in a Vector
, it is recommended to create a type-specific swap()
specialization for the T
type:
template <> void Vector<int>::swap(size_t index1, size_t index2) { // 对 int 类型元素进行特殊处理 }
This avoids instantiating the genericswap()
method multiple times, thus improving performance.
The above is the detailed content of What are the performance considerations in C++ generic programming?. For more information, please follow other related articles on the PHP Chinese website!
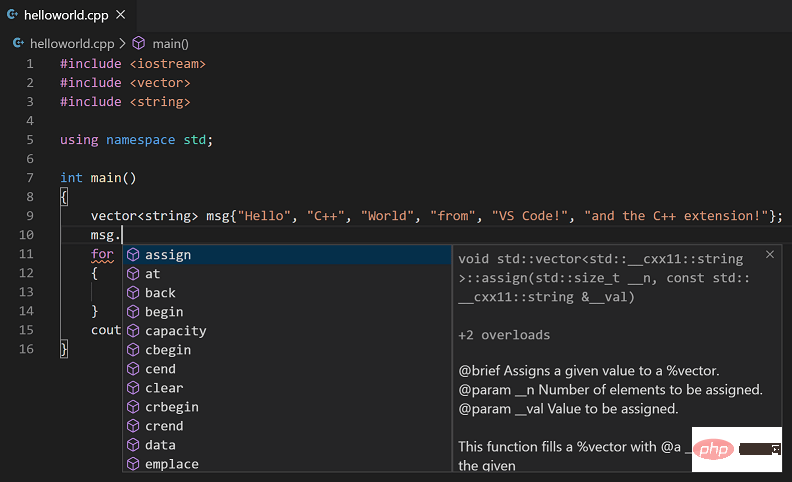
C++是一种广泛使用的面向对象的计算机编程语言,它支持您与之交互的大多数应用程序和网站。你需要编译器和集成开发环境来开发C++应用程序,既然你在这里,我猜你正在寻找一个。我们将在本文中介绍一些适用于Windows11的C++编译器的主要推荐。许多审查的编译器将主要用于C++,但也有许多通用编译器您可能想尝试。MinGW可以在Windows11上运行吗?在本文中,我们没有将MinGW作为独立编译器进行讨论,但如果讨论了某些IDE中的功能,并且是DevC++编译器的首选
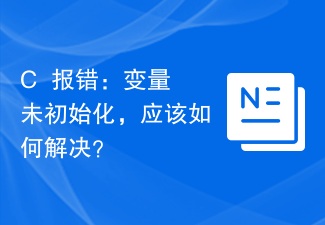
在C++程序开发中,当我们声明了一个变量但是没有对其进行初始化,就会出现“变量未初始化”的报错。这种报错经常会让人感到很困惑和无从下手,因为这种错误并不像其他常见的语法错误那样具体,也不会给出特定的代码行数或者错误类型。因此,下面我们将详细介绍变量未初始化的问题,以及如何解决这个报错。一、什么是变量未初始化错误?变量未初始化是指在程序中声明了一个变量但是没有
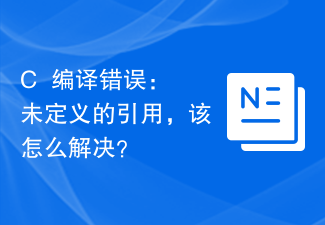
C++是一门广受欢迎的编程语言,但是在使用过程中,经常会出现“未定义的引用”这个编译错误,给程序的开发带来了诸多麻烦。本篇文章将从出错原因和解决方法两个方面,探讨“未定义的引用”错误的解决方法。一、出错原因C++编译器在编译一个源文件时,会将它分为两个阶段:编译阶段和链接阶段。编译阶段将源文件中的源码转换为汇编代码,而链接阶段将不同的源文件合并为一个可执行文
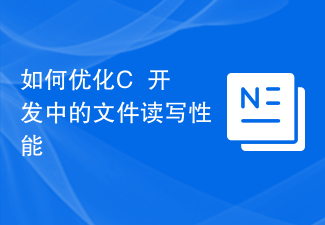
如何优化C++开发中的文件读写性能在C++开发过程中,文件的读写操作是常见的任务之一。然而,由于文件读写是磁盘IO操作,相对于内存IO操作来说会更为耗时。为了提高程序的性能,我们需要优化文件读写操作。本文将介绍一些常见的优化技巧和建议,帮助开发者在C++文件读写过程中提高性能。使用合适的文件读写方式在C++中,文件读写可以通过多种方式实现,如C风格的文件IO
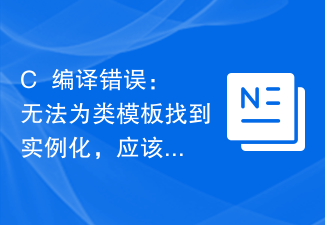
C++是一门强大的编程语言,它支持使用类模板来实现代码的复用,提高开发效率。但是在使用类模板时,可能会遭遇编译错误,其中一个比较常见的错误是“无法为类模板找到实例化”(error:cannotfindinstantiationofclasstemplate)。本文将介绍这个问题的原因以及如何解决。问题描述在使用类模板时,有时会遇到以下错误信息:e
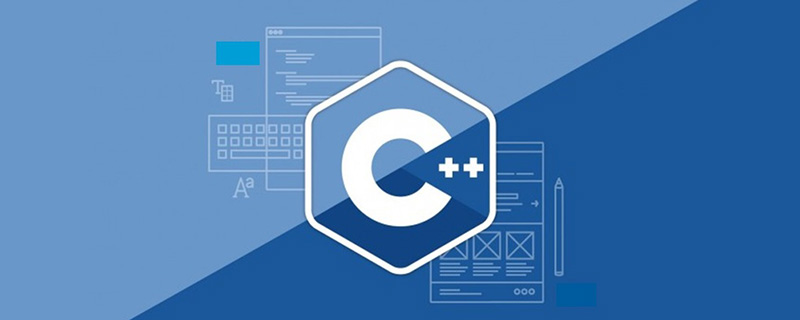
iostream头文件包含了操作输入输出流的方法,比如读取一个文件,以流的方式读取;其作用是:让初学者有一个方便的命令行输入输出试验环境。iostream的设计初衷是提供一个可扩展的类型安全的IO机制。
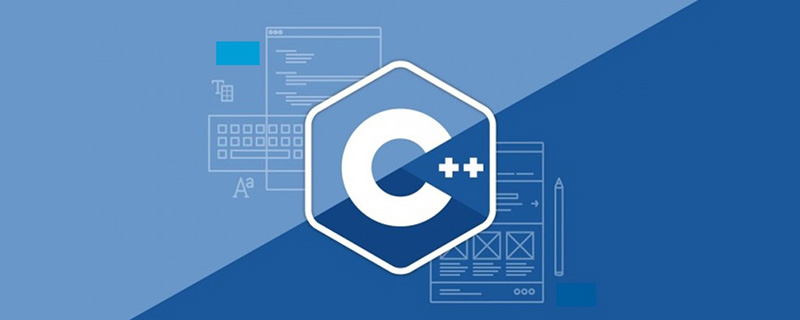
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。
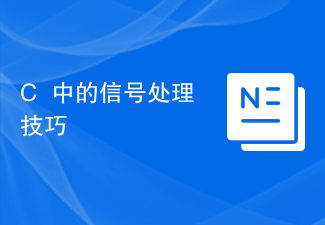
C++是一种流行的编程语言,它强大而灵活,适用于各种应用程序开发。在使用C++开发应用程序时,经常需要处理各种信号。本文将介绍C++中的信号处理技巧,以帮助开发人员更好地掌握这一方面。一、信号处理的基本概念信号是一种软件中断,用于通知应用程序内部或外部事件。当特定事件发生时,操作系统会向应用程序发送信号,应用程序可以选择忽略或响应此信号。在C++中,信号可以


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
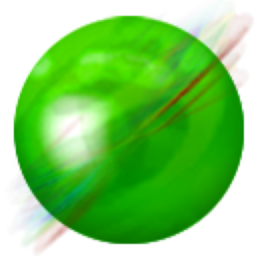
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
