


How does C++ memory management prevent memory leaks and wild pointer problems?
For memory management in C, there are two common errors: memory leaks and wild pointers. Methods to solve these problems include: using smart pointers (such as std::unique_ptr and std::shared_ptr) to automatically release memory that is no longer used; following the RAII principle to ensure that resources are released when the object goes out of scope; initializing the pointer and accessing only Valid memory, with array bounds checking; always use the delete keyword to free dynamically allocated memory that is no longer needed.
C Memory Management: Preventing Memory Leakage and Wild Pointer Problems
Preface
Memory management is a crucial aspect of C. Improper handling can lead to serious errors such as memory leaks and wild pointers. This article will explore how to manage memory effectively to prevent these problems.
What is a memory leak?
Memory leaks occur when dynamically allocated memory is no longer used by the program, but the memory is still occupied. This can cause serious performance issues and memory exhaustion over time.
What is a wild pointer?
A wild pointer is a pointer to a deleted or unknown memory location. When a wild pointer is dereferenced, undefined behavior can result, such as a segfault or incorrect result.
How to prevent memory leaks
-
Use smart pointers: Smart pointers (such as
std::unique_ptr
andstd::shared_ptr
) Automatically manages memory and automatically releases memory when the object goes out of scope. - Follow the RAII principle: The RAII (resource acquisition is initialization) principle requires that resources be obtained and cleaned up during the life cycle of the object. This ensures that all resources are released when the object is destroyed.
-
Use the
delete
keyword: When dynamically allocated memory is no longer needed, use thedelete
keyword to explicitly free it.
How to prevent wild pointers
-
Always initialize pointers: Always initialize a pointer before using it Is
nullptr
or a valid value. - Access only valid memory: Make sure the pointer points to a valid memory location. Avoid dereferencing dangling pointers or out-of-bounds accesses.
- Use array bounds checking: When accessing an array, perform bounds checking to avoid accessing unsafe memory.
Practical case
The following code snippet shows how to use smart pointers to prevent memory leaks and wild pointers:
#include <memory> class MyClass { public: MyClass() { std::cout << "MyClass constructed" << std::endl; } ~MyClass() { std::cout << "MyClass destructed" << std::endl; } }; int main() { // 使用智能指针防止内存泄漏 { std::unique_ptr<MyClass> ptr = std::make_unique<MyClass>(); // ... 使用 MyClass ... } // ptr 析构,自动释放 MyClass 对象 // 防止野指针 MyClass* rawPtr = new MyClass(); { // 在作用域内确保 rawPtr 指向有效内存 delete rawPtr; // 手动释放 rawPtr 指向的 MyClass 对象 } rawPtr = nullptr; // 重置 rawPtr 以使其指向 nullptr,防止野指针 return 0; }
By using smart pointers Pointers and by following best practices, you can manage memory efficiently and prevent memory leaks and wild pointer problems.
The above is the detailed content of How does C++ memory management prevent memory leaks and wild pointer problems?. For more information, please follow other related articles on the PHP Chinese website!
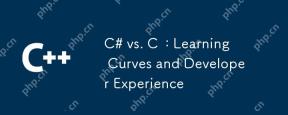
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
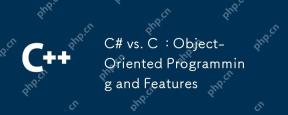
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
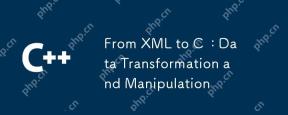
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
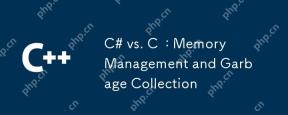
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
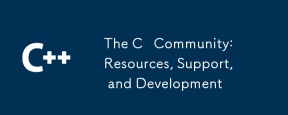
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
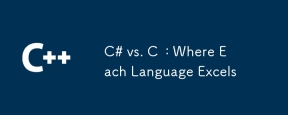
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
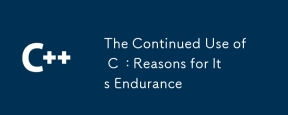
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
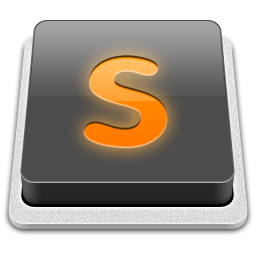
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Notepad++7.3.1
Easy-to-use and free code editor