


Best practices for coding style in PHP framework: clear, maintainable, and efficient
Coding style best practices in the PHP framework: clear, maintainable, efficient
Following a consistent and clear coding style in the PHP framework is essential for maintaining the integrity of the code base. Maintainability, collaboration, and readability are critical. This article will share the best practices of coding style in the PHP framework and provide practical cases for reference.
Indentation and line breaks
- Use 4 spaces for indentation and avoid tabs.
- Continuously indented blocks of code should be left-aligned and contain only one statement per line of code.
- Use newlines to separate adjacent statements and blocks of code to improve readability.
Naming convention
- Use camel notation for naming variables, functions, and methods.
- Use underline nomenclature to name constants.
- Class names should start with a capital letter.
Example:
// 变量 $myVariable; // 函数 function myAwesomeFunction() {} // 方法 public function myWonderfulMethod() {} // 常量 const API_KEY; // 类 class MyAwesomeClass {}
Comments
- Use documentation blocks to comment functions, methods, and classes.
- Follow PHPDoc format to write comments, including type declarations and descriptions.
- Comments should be concise and to the point, providing enough information to explain the function of the code.
Example:
/** * 获取用户的详细信息 * * @param int $userId 用户 ID * @return array 用户详细信息 */ public function getUserDetails(int $userId): array { // 获取用户详细信息 }
Grammar and language features
- Avoid using complex syntax constructs such as switch-case.
- Use expression syntax in preference to statement syntax.
- Use type declarations whenever possible to improve code readability and safety.
Example:
// 表达式语法 $output = 1 < 2 ? 'True' : 'False'; // 避免使用 switch-case $result = match ($action) { 'create' => createSomething(), 'update' => updateSomething(), 'delete' => deleteSomething(), default => null, };
Code Organization
- Group related code into modular methods or classes.
- Use namespaces to organize code and improve maintainability.
- Follow the DRY principle (don’t repeat yourself) to avoid duplicating code.
Example:
// 模块化方法 private function createSomething(array $data): void { // ... } // 命名空间 namespace App\Models; class User { // ... }
Actual case
Clear:
- ##Name Conventions are clear and consistent, making it easy for team members to understand the code.
- Comments are detailed and concise, providing a clear explanation of what the code does.
Maintainable:
- The code is well organized, modular and avoids duplication.
- Using type declarations can reduce errors and improve code safety.
Efficient:
- Expression syntax improves code simplicity and readability.
- Optimizing algorithms and data structures can improve the efficiency of the code.
The above is the detailed content of Best practices for coding style in PHP framework: clear, maintainable, and efficient. For more information, please follow other related articles on the PHP Chinese website!
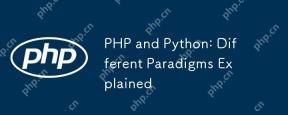
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
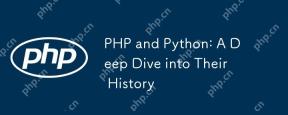
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
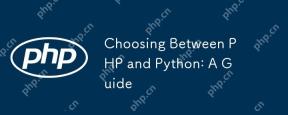
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
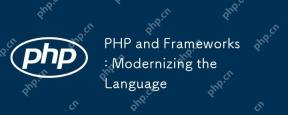
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
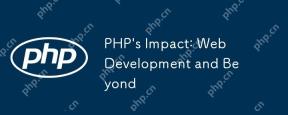
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
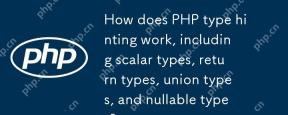
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
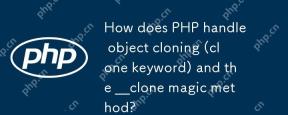
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
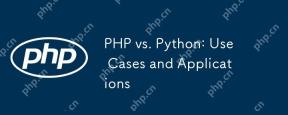
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
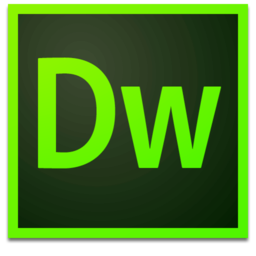
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
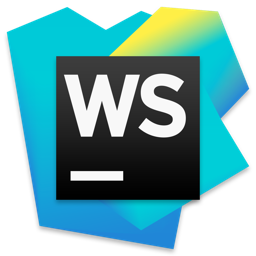
WebStorm Mac version
Useful JavaScript development tools