Array sorting algorithm is used to arrange elements in a specific order. Common types of algorithms include: Bubble sort: swap positions by comparing adjacent elements. Selection sort: Find the smallest element and swap it to the current position. Insertion sort: Insert elements one by one to the correct position. Quick sort: divide and conquer method, select the pivot element to divide the array. Merge Sort: Divide and Conquer, Recursive Sorting and Merging Subarrays.
Introduction and practice of array sorting algorithm
In computer science, the array sorting algorithm is used to sort a set of elements according to a specific An algorithm for sorting in order. Sorting algorithms are divided into many different types based on their principles and efficiency. The following will introduce some common array sorting algorithms and demonstrate their use through practical cases.
Bubble sort
Bubble sort is a simple and easy-to-understand sorting algorithm. Its basic principle is to compare the sizes of adjacent elements in sequence. If the previous If the element is larger than the next element, their positions are swapped. This process is repeated until all elements are in order.
def bubble_sort(arr): for i in range(len(arr) - 1): for j in range(len(arr) - i - 1): if arr[j] > arr[j + 1]: arr[j], arr[j + 1] = arr[j + 1], arr[j]
Selection sort
Selection sort is also a simple sorting algorithm. Its principle is to find the smallest element of the unsorted part and exchange it with the current position element. . Then repeat the process until all elements are in order.
def selection_sort(arr): for i in range(len(arr)): min_idx = i for j in range(i + 1, len(arr)): if arr[j] < arr[min_idx]: min_idx = j arr[i], arr[min_idx] = arr[min_idx], arr[i]
Insertion sort
Insertion sort is a sorting algorithm based on insertion operations. Its basic principle is to insert elements one by one into the correct position of the sorted part until All elements are arranged in order.
def insertion_sort(arr): for i in range(1, len(arr)): key = arr[i] j = i - 1 while j >= 0 and key < arr[j]: arr[j + 1] = arr[j] j -= 1 arr[j + 1] = key
Quick sort
Quick sort is a divide-and-conquer sorting algorithm. Its principle is to divide the array into two sub-arrays by selecting a pivot element, and then recursively Sort both subarrays until all elements are in order.
def quick_sort(arr): if len(arr) <= 1: return arr pivot = arr[len(arr) // 2] left = [x for x in arr if x < pivot] middle = [x for x in arr if x == pivot] right = [x for x in arr if x > pivot] return quick_sort(left) + middle + quick_sort(right)
Merge sort
Merge sort is a stable and efficient divide-and-conquer sorting algorithm. Its principle is to recursively divide the array into smaller sub-arrays. , then sort and merge the subarrays until all elements are in order.
def merge_sort(arr): if len(arr) <= 1: return arr mid = len(arr) // 2 left_half = merge_sort(arr[:mid]) right_half = merge_sort(arr[mid:]) return merge(left_half, right_half) def merge(left, right): merged = [] left_idx, right_idx = 0, 0 while left_idx < len(left) and right_idx < len(right): if left[left_idx] <= right[right_idx]: merged.append(left[left_idx]) left_idx += 1 else: merged.append(right[right_idx]) right_idx += 1 merged.extend(left[left_idx:]) merged.extend(right[right_idx:]) return merged
Practical case
Suppose we have an unordered listarr = [5, 2, 8, 3, 1, 9]
, we can use the quick sort algorithm to sort it, the code is as follows:
arr = [5, 2, 8, 3, 1, 9] sorted_arr = quick_sort(arr) print(sorted_arr) # 输出:[1, 2, 3, 5, 8, 9]
The above is the detailed content of What are the sorting algorithms for arrays?. For more information, please follow other related articles on the PHP Chinese website!
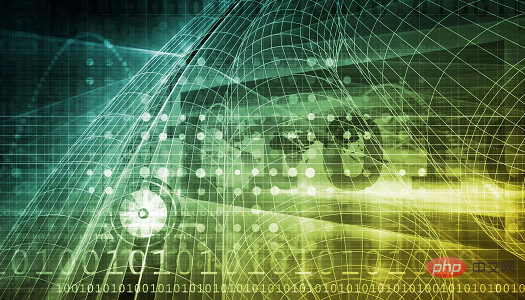
一、问题背景1、双边市场实验介绍双边市场,即平台,包含生产者与消费者两方参与者,双方相互促进。比如快手有视频的生产者,视频的消费者,两种身份可能存在一定程度重合。双边实验是在生产者和消费者端组合分组的实验方式。双边实验具有以下优点:(1)可以同时检测新策略对两方面的影响,例如产品DAU和上传作品人数变化。双边平台往往有跨边网络效应,读者越多,作者越活跃,作者越活跃,读者也会跟着增加。(2)可以检测效果溢出和转移。(3)帮助我们更好得理解作用的机制,AB实验本身不能告诉我们原因和结果之间的关系,只
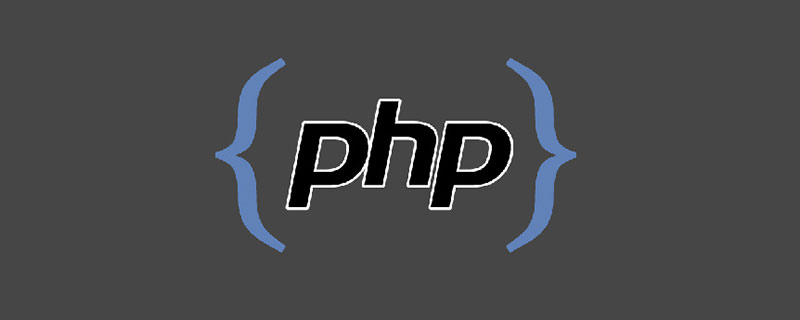
php求2个数组相同元素的方法:1、创建一个php示例文件;2、定义两个有相同元素的数组;3、使用“array_intersect($array1,$array2)”或“array_intersect_assoc()”方法获取两个数组相同元素即可。
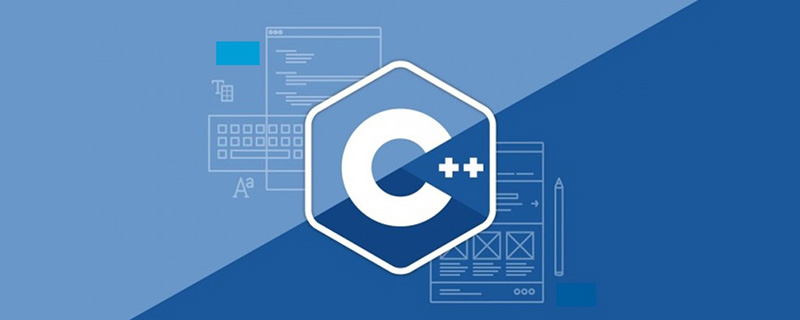
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。
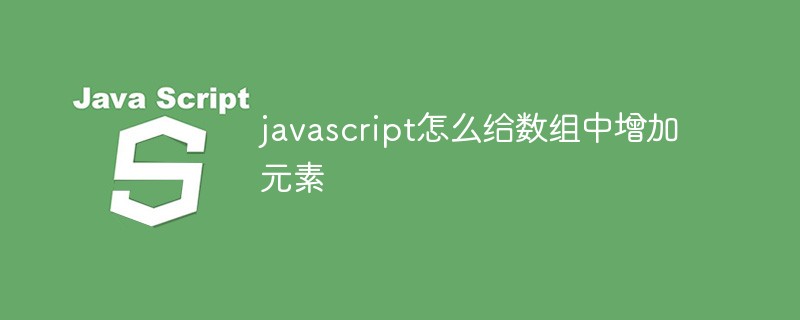
增加元素的方法:1、使用unshift()函数在数组开头插入元素;2、使用push()函数在数组末尾插入元素;3、使用concat()函数在数组末尾插入元素;4、使用splice()函数根据数组下标,在任意位置添加元素。
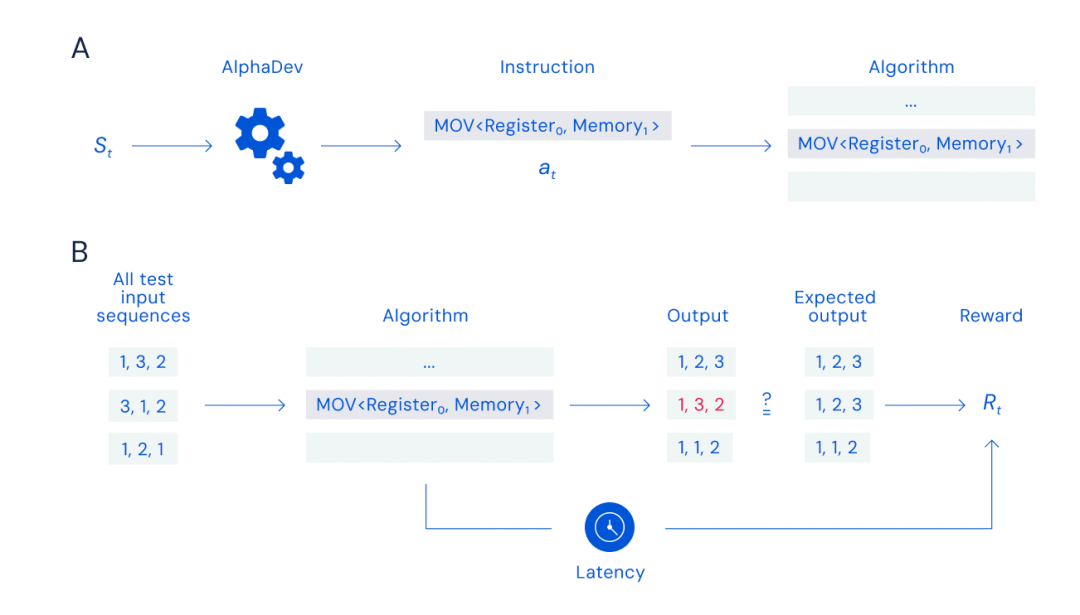
整理|核子可乐,褚杏娟接触过基础计算机科学课程的朋友们,肯定都曾亲自动手设计排序算法——也就是借助代码将无序列表中的各个条目按升序或降序方式重新排列。这是个有趣的挑战,可行的操作方法也多种多样。人们曾投入大量时间探索如何更高效地完成排序任务。作为一项基础操作,大多数编程语言的标准库中都内置有排序算法。世界各地的代码库中使用了许多不同的排序技术和算法来在线组织大量数据,但至少就与LLVM编译器配套使用的C++库而言,排序代码已经有十多年没有任何变化了。近日,谷歌DeepMindAI小组如今开发出一
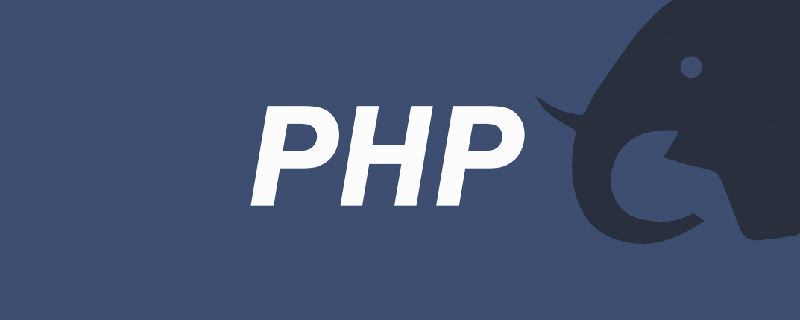
php判断数组里面是否存在某元素的方法:1、通过“in_array”函数在数组中搜索给定的值;2、使用“array_key_exists()”函数判断某个数组中是否存在指定的key;3、使用“array_search()”在数组中查找一个键值。
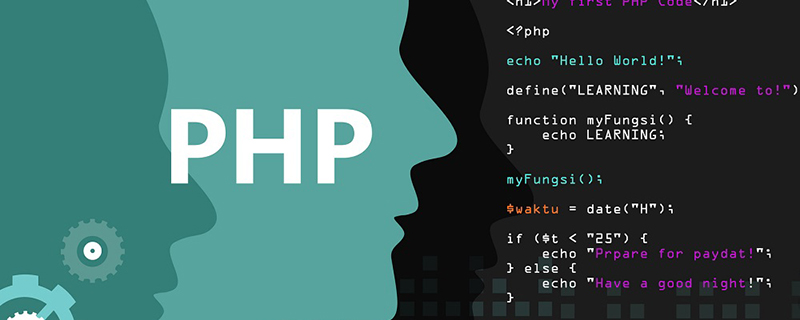
php去除第一个数组元素的方法:1、新建一个php文件,并创建一个数组;2、使用“array_shift”方法删除数组首个元素;3、通过“print_”r输出数组即可。
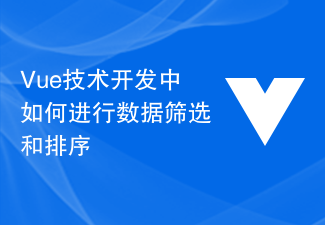
Vue技术开发中如何进行数据筛选和排序在Vue技术开发中,数据筛选和排序是非常常见和重要的功能。通过数据筛选和排序,我们可以快速查询和展示我们需要的信息,提高用户体验。本文将介绍在Vue中如何进行数据筛选和排序,并提供具体的代码示例,帮助读者更好地理解和运用这些功能。一、数据筛选数据筛选是指根据特定的条件筛选出符合要求的数据。在Vue中,我们可以通过comp


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
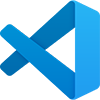
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
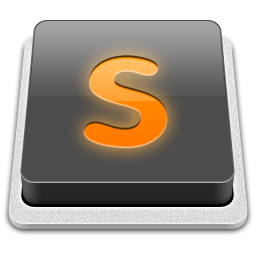
SublimeText3 Mac version
God-level code editing software (SublimeText3)
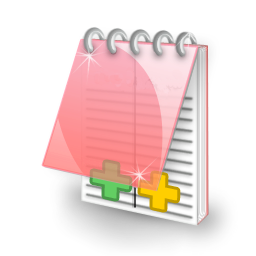
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
