Golang Concurrent Programming Framework Guide: Goroutines: lightweight coroutines to achieve parallel operation; Channels: pipelines, used for communication between goroutines; WaitGroups: allows the main coroutine to wait for multiple goroutines to complete; Context: provides goroutine context information, Such as cancellations and deadlines.
Golang Concurrent Programming Framework Guide
Introduction
Concurrent programming in building high-performance and scalable applications crucial in the program. Golang provides a wealth of concurrency primitives, but choosing the right framework can further simplify and improve the efficiency of concurrent programming. This article will explore various popular Golang concurrent programming frameworks and show their practical cases.
1. Goroutines
Goroutines are lightweight coroutines in Golang that can run in parallel in different threads. They are very efficient and easy to use, especially suitable for CPU-intensive tasks.
package main import ( "fmt" "time" ) func main() { // 创建一个 goroutine 来打印消息 go func() { for i := 0; i < 10; i++ { fmt.Println("Hello, world!") time.Sleep(100 * time.Millisecond) } }() // 主协程等待 goroutine 完成 time.Sleep(10 * time.Second) }
2. Channels
Channels are pipes used for communication between goroutines. They provide a safe and efficient way to pass values and synchronize operations.
package main import ( "fmt" "time" ) func main() { // 创建一个 channel 来传递值 ch := make(chan string) // 创建一个 goroutine 来发送数据到 channel go func() { ch <- "Hello, world!" }() // 接收 goroutine 发送的值 msg := <-ch fmt.Println(msg) time.Sleep(10 * time.Second) }
3. WaitGroups
WaitGroups allows the main coroutine to wait for multiple goroutines to complete. This helps ensure that execution of the main logic does not continue until all goroutines have finished running.
package main import ( "fmt" "sync" ) func main() { // 创建一个 WaitGroup wg := &sync.WaitGroup{} // 添加需要等待的 goroutine 数量 wg.Add(2) // 创建并运行 goroutines go func() { fmt.Println("Goroutine 1") wg.Done() }() go func() { fmt.Println("Goroutine 2") wg.Done() }() // 主协程等待 goroutines 完成 wg.Wait() fmt.Println("All goroutines completed") }
4. Context
Context provides contextual information in the goroutine, such as cancellation and deadline times. This helps manage concurrent requests and operations.
package main import ( "context" "fmt" "time" ) func main() { // 创建一个 context ctx, _ := context.WithTimeout(context.Background(), 5*time.Second) // 创建一个 goroutine 并传递 context go func(ctx context.Context) { fmt.Println("Goroutine started") // 监听 context 是否已取消 select { case <-ctx.Done(): fmt.Println("Goroutine canceled") return } // ... 执行其他操作 }(ctx) // 等待 3 秒后取消 context time.Sleep(3 * time.Second) ctx.Done() }
Conclusion
Golang provides powerful concurrent programming primitives, and these frameworks further simplify and improve the efficiency of concurrent programming. Choosing the right framework depends on the specific needs of your application. By understanding these frameworks, developers can build high-performance and scalable parallel programs.
The above is the detailed content of Which golang framework is most suitable for concurrent programming?. For more information, please follow other related articles on the PHP Chinese website!
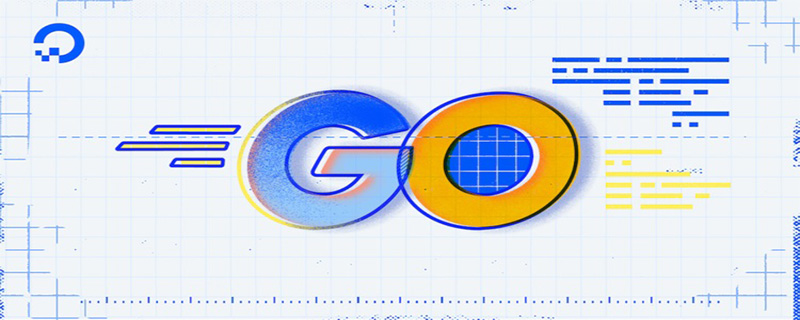
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
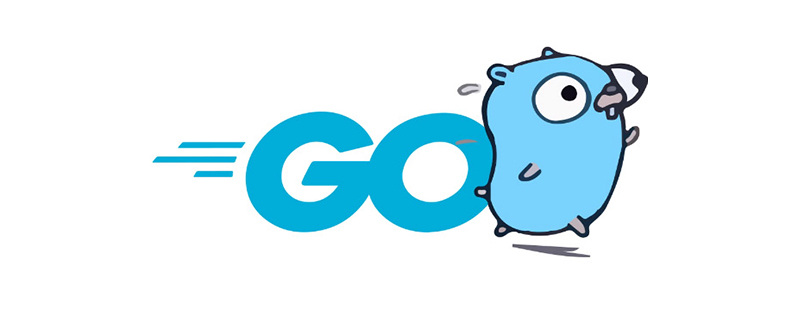
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
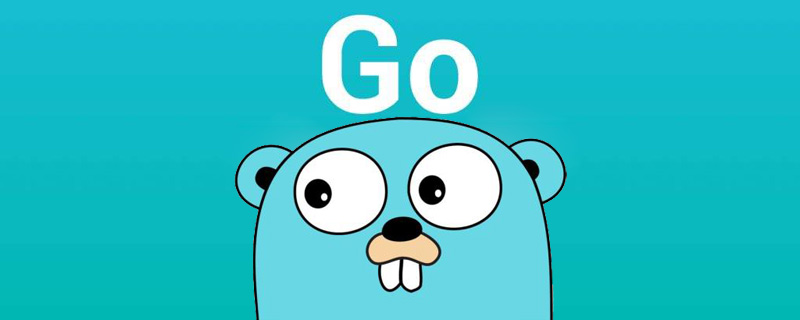
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
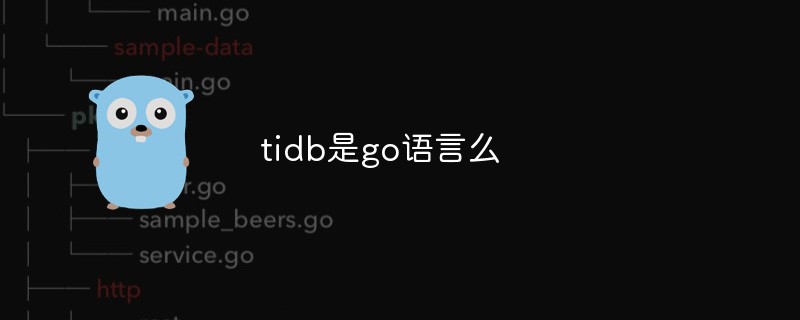
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
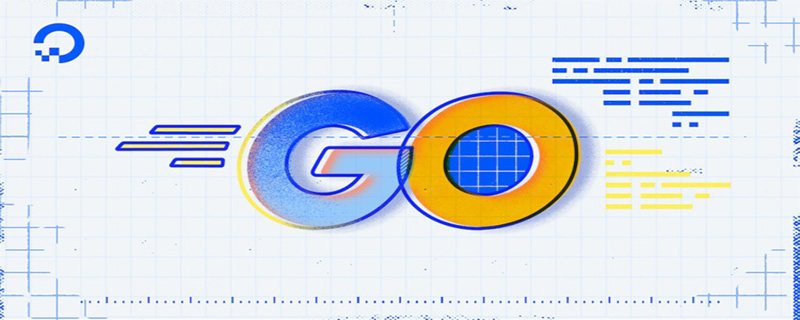
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
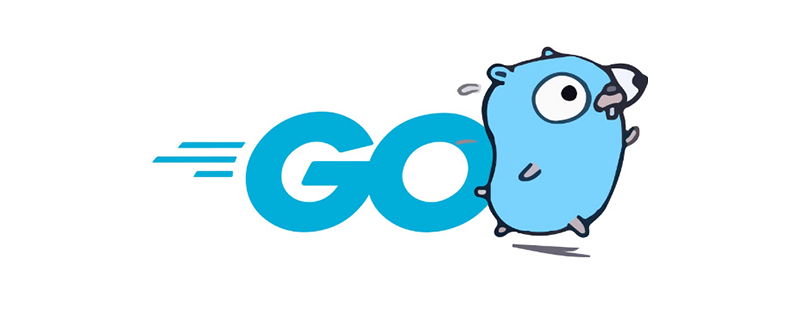
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
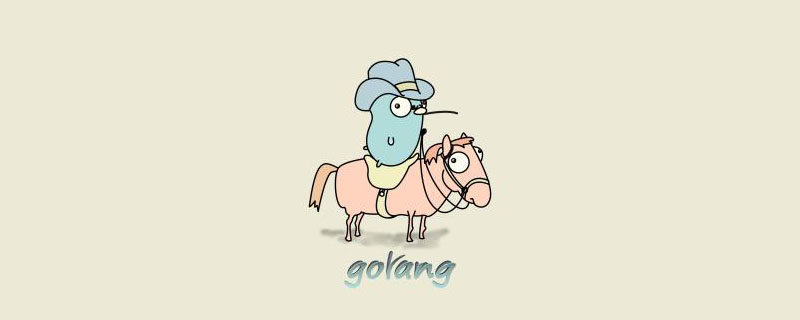
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
