The Go framework custom debugger provides powerful features for debugging large Go applications: Monitor and debug concurrent Goroutines Check memory status and resource leaks Explore the internal structure of the Go framework
Go Framework Custom Debugger
When debugging large Go applications, the standard debugger may not be enough. Custom debuggers can provide more powerful features, such as:
- Monitor and debug concurrent Goroutines
- Check memory status and resource leaks
- Explore the internals of the Go framework Structure
Practical example: Debugging the Gin framework
As an example, let's create a custom debugger to debug the Gin framework.
import ( "fmt" "github.com/gin-gonic/gin" ) // LoggerMiddleware 是一个 Gin 中间件,用于记录请求信息。 func LoggerMiddleware(c *gin.Context) { fmt.Println("Received request:", c.Request.Method, c.Request.URL.Path) // 继续处理请求 c.Next() }
Creating a custom debugger
We create a custom debugger that integrates with net/http/pprof
.
import ( "net/http/pprof" ) func CreateDebugger(router *gin.Engine) { // 添加 pprof 路由 router.GET("/debug/pprof/", pprof.Index) router.GET("/debug/pprof/cmdline", pprof.Cmdline) router.GET("/debug/pprof/profile", pprof.Profile) // 应用 LoggerMiddleware,以便在每条请求上记录信息 router.Use(LoggerMiddleware) }
Run the application
func main() { router := gin.New() CreateDebugger(router) router.Use(gin.Recovery()) router.Run(":8080") }
Use the debugger
Open your browser and navigate to http:// /localhost:8080/debug/pprof/
. This will display a page containing various debugging functions.
- CPU Profiling: Analyze the CPU usage of your application.
- Memory Profiling: Analyze the memory usage of the application.
- Goroutine Anatomy: Monitor Goroutines in an application.
Through these features, you can gain insight into the behavior of your application, discover performance bottlenecks and debug issues.
The above is the detailed content of golang framework custom debugger. For more information, please follow other related articles on the PHP Chinese website!
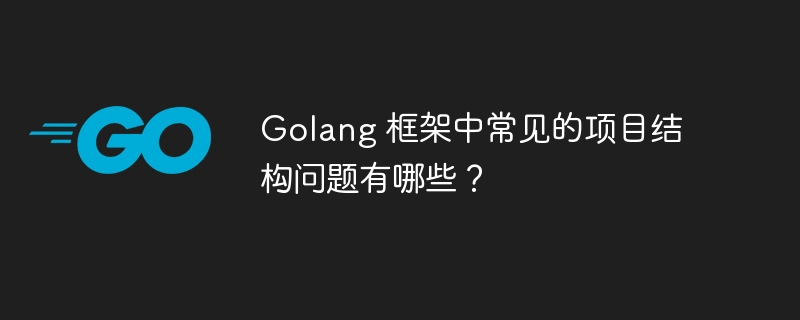
Go项目的常见结构问题包括:缺乏分层:解决方法:采用垂直分层结构,使用接口实现松散耦合。过度嵌套:解决方法:减少嵌套深度,使用函数或结构体封装复杂逻辑。缺少模块化:解决方法:将代码分解成可管理的模块,使用包和依赖管理工具。路由多级目录:解决方法:使用明确的目录结构,避免依赖关系过多的目录。缺乏自动化测试:解决方法:模块化测试逻辑,使用自动化测试框架。
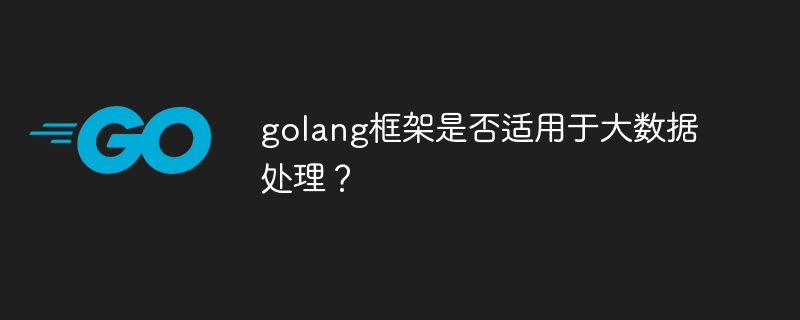
Go框架在巨量数据处理中表现出色,其优势包括并发性、高性能和类型安全。适用于大数据处理的Go框架包括ApacheBeam、Flink和Spark。在实战案例中,利用Beam管道可以高效地处理和转换大批量数据,例如将字符串列表转换为大写。

目前最流行的Go框架有:Gin:轻量级、高性能的Web框架,简洁易用。Echo:快速、高度可定制的Web框架,提供高性能路由和中间件。GorillaMux:快速、灵活的多路复用器,提供高级路由配置选项。Fiber:性能优化的高性能Web框架,处理高并发请求。Martini:面向对象设计的模块化Web框架,提供丰富的功能集。
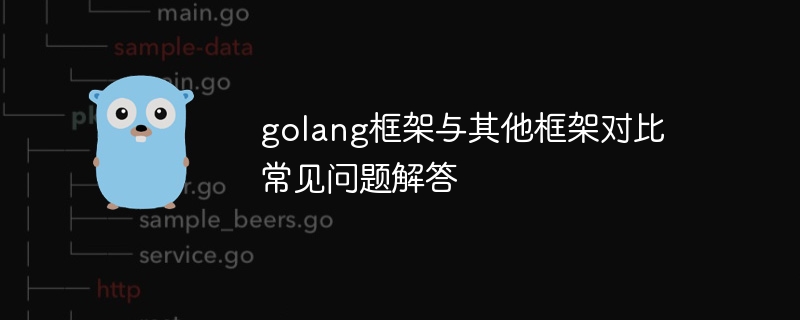
GoLang框架与其他框架的对比:与Django相比:注重类型安全和并发性。与Node.js相比:以高性能和内存效率著称。与SpringBoot相比:更注重性能,适合大型应用。
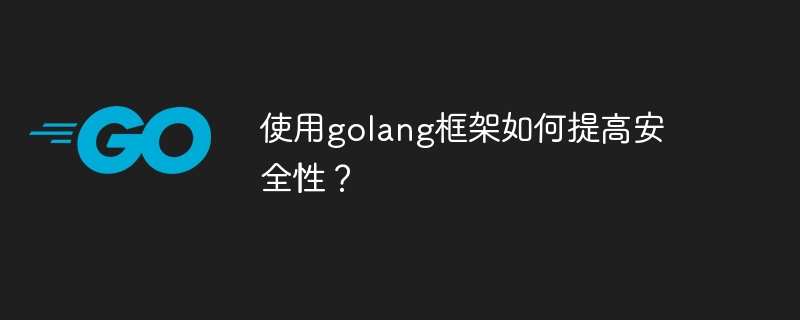
通过以下步骤可提升Golang应用安全性:加密和认证:利用crypto/tls和crypto/bcrypt加密数据,并使用oauth2进行身份验证。输入验证:使用validator和regexp验证用户输入,防止恶意攻击。SQL注入保护:使用sqlx查询构建器和database/sqlScan方法抵御SQL注入。
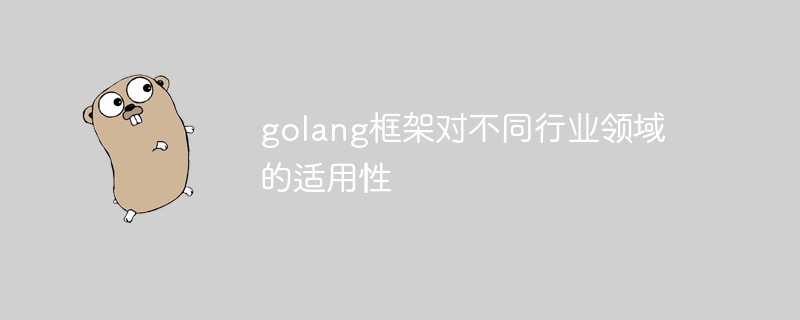
Golang框架适用于:1.Web开发(Gin、Echo);2.微服务(gRPC、Kafka);3.数据处理(ApacheBeam、ApacheFlink);4.区块链(HyperledgerFabric、Ethereum);5.DevOps(GoCD、Jenkins)。选择框架时应考虑应用程序类型、性能要求、可扩展性需求和安全性顾虑。
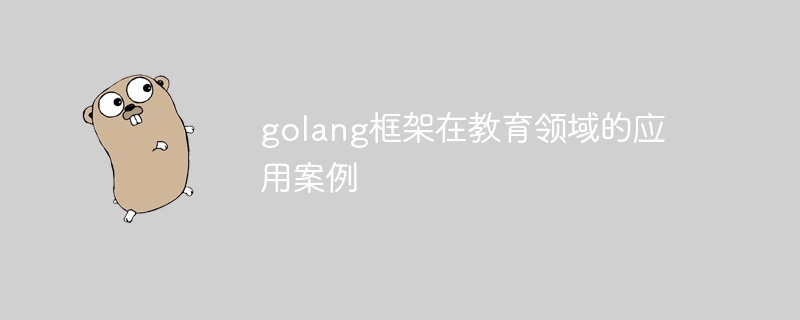
Go框架在教育领域得到广泛应用。案例一展示了基于Go开发的高性能在线学习平台,具有互动课程和作业评估功能。案例二则是一套功能强大的学校管理系统,集中管理学生信息、教师详情和财务记录。这表明Go框架在教育领域的优势在于:高性能、可扩展性和易于维护。
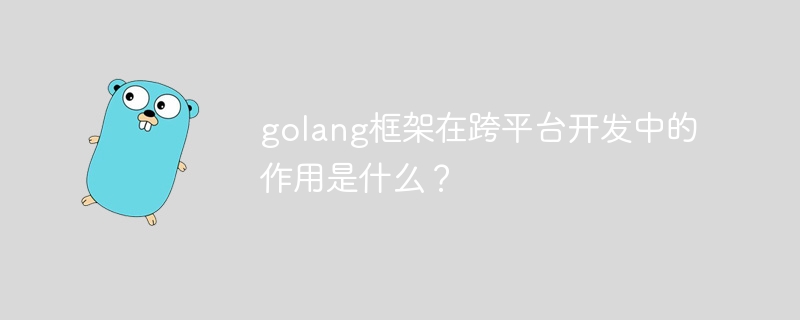
Go框架在跨平台开发中的作用:促进代码复用,提高开发效率和代码维护性。提供平台抽象层,隐藏底层平台差异。提供工具和实用程序,简化跨平台开发,例如gotool和goget。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
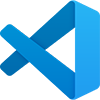
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
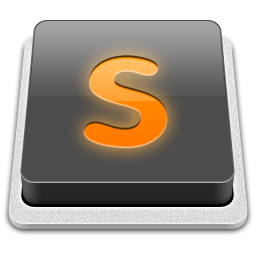
SublimeText3 Mac version
God-level code editing software (SublimeText3)
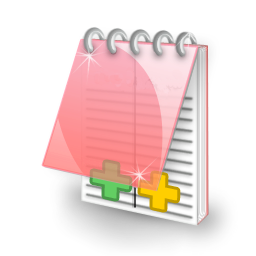
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
