Answer: C Cloud Computing Seamless integration with AWS, Azure and GCP. Expand description: The AWS SDK for C simplifies interacting with AWS services like Amazon EC2 and S3. Azure SDK for C enables the use of Azure compute, storage, and database services. The GCP Cloud SDK and gRPC client libraries support integration with Google Compute Engine and Google BigQuery.
C Cloud Computing: Integration with Mainstream Cloud Platforms
Cloud computing has become an indispensable part of modern software development. It provides on-demand access to scalable computing resources, allowing developers to focus on building applications rather than managing the underlying infrastructure. For developers using C, integration with major cloud platforms is critical.
Amazon Web Services (AWS)
AWS is the world’s largest cloud platform, providing a range of services to C developers. The AWS SDK for C simplifies interaction with AWS services such as Amazon Elastic Compute Cloud (EC2), Amazon Simple Storage Service (S3), and Amazon DynamoDB.
#include <aws/core/Aws.h> #include <aws/core/client/AsyncCallerContext.h> #include <aws/ec2/Ec2Client.h> #include <aws/ec2/model/RunInstancesRequest.h> int main() { Aws::InitAPI(Aws::SDKOptions{}); { Aws::Client::AsyncCallerContext context; Aws::EC2::Ec2Client ec2_client; Aws::EC2::Model::RunInstancesRequest run_instances_request; run_instances_request.SetImageId("ami-id"); run_instances_request.SetInstanceType("t2.micro"); run_instances_request.SetMinCount(1); run_instances_request.SetMaxCount(1); auto outcome = ec2_client.RunInstancesAsync(run_instances_request, context); if (outcome.IsSuccess()) { std::cout << "Instance launched" << std::endl; } else { std::cout << "Error launching instance: " << outcome.GetError().GetMessage() << std::endl; } } Aws::ShutdownAPI(Aws::SDKOptions{}); return 0; }
Microsoft Azure
Azure also offers a wide range of services for C developers. Azure SDK for C allows developers to use Azure compute, storage, and database services.
#include <azure/core.hpp> #include <azure/identity.hpp> #include <azure/storage.hpp> int main() { auto tenant_id = std::getenv("AZURE_TENANT_ID"); auto client_id = std::getenv("AZURE_CLIENT_ID"); auto client_secret = std::getenv("AZURE_CLIENT_SECRET"); auto storage_account_name = std::getenv("AZURE_STORAGE_ACCOUNT_NAME"); Azure::Core::Credentials::ManagedIdentityCredential creds(tenant_id, client_id, client_secret); Azure::Storage::StorageClient storage_client(storage_account_name, creds); auto blob_client = storage_client.GetBlobContainerClient("container-name"); std::string blob_name = "blob-name"; auto blob = blob_client.DownloadBlob(blob_name); std::cout << "Downloaded blob: " << blob.ContentAs<std::string>() << std::endl; return 0; }
Google Cloud Platform (GCP)
GCP also provides a comprehensive set of C services. The GCP Cloud SDK and gRPC client libraries enable developers to easily integrate Google Cloud services such as Google Compute Engine (GCE), Google Cloud Storage (GCS), and Google BigQuery.
#include <gmock/gmock.h> #include <google/cloud/bigquery/bigquery_read_client.h> #include <google/cloud/bigquery/metadata.h> #include <google/cloud/bigquery/storage/bigquery_storage_client.h> TEST(BigqueryStorageReadWriteIntegrationTest, Read) { google::cloud::bigquery::storage::BigQueryReadClient client; auto channel = client.CreateBigQueryReadChannel( google::cloud::bigquery::storage::v1beta1::ReadSession{}); auto session = google::cloud::bigquery::storage::v1beta1::ReadSession{}; auto writer = client.WriteReadSessionAsync(channel.get(), google::cloud::CompletionQueue{}, std::move(session)); google::cloud::StatusOr<google::cloud::bigquery::storage::v1beta1:: ReadRowsResponse> rows_received; auto read_rows = client.ReadRowsAsync(channel.get(), google::cloud::CompletionQueue{}, google::bigquery::ReadRowsRequest{}); google::cloud::future<void> session_write = writer.get(); do { rows_received = read_rows.get(); } while (true); EXPECT_STATUS_OK(rows_received); }
Conclusion
By integrating with mainstream cloud platforms, C developers can take advantage of elastic, scalable cloud resources. AWS SDK for C, Azure SDK for C, and GCP Cloud SDK provide easy-to-use APIs, giving developers the ability to interact seamlessly with these platforms. These practical examples demonstrate how to use these SDKs to interact with cloud services, thereby expanding the possibilities of C applications.
The above is the detailed content of C++ cloud computing: integration with mainstream cloud platforms. For more information, please follow other related articles on the PHP Chinese website!
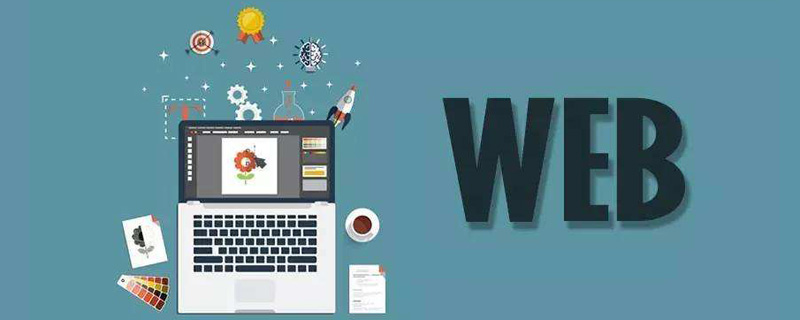
云计算与web前端有挂钩。云计算在web前端的体现就是可以到云里拿一些资源来支撑业务;这些资源可以是计算能力、存储空间等硬件资源,也可以是各种应用、服务甚至桌面等软件资源。再次细分之后可以看到,当云计算体现到前端时,终端用户获得的要么是应用,要么是桌面;那桌面云的概念就应运而生了。桌面云的重点也在于应用,为用户搭建了种种桌面云应用环境,解决用户所遇到的各种业务问题。
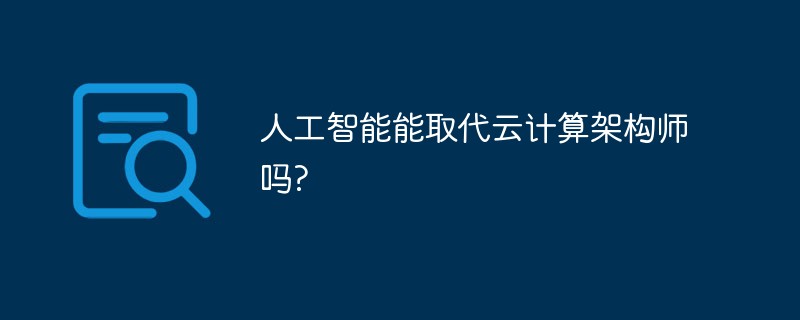
人工智能系统每天都令人印象深刻。如今的人工智能可以自动化许多信息工作者的任务,因此那些从事云计算工作的人担心自己会成为下一个。人们对人工智能及其应用的兴趣大约在五年前发生了变化。后来发生了大流行,一些预算转向了快速云迁移。现在一切都恢复正常了,人工智能又回来了。大多数企业都掌握了人工智能的基本可能性,并希望将这项技术武器化,用于自己的业务。在这个过程中,技术变得更加令人印象深刻。例如,随着ChatGPT等生成式AI服务的出现,生成式人工智能从博士论文变成了可访问的免费现实。生成式人工智能是一种基
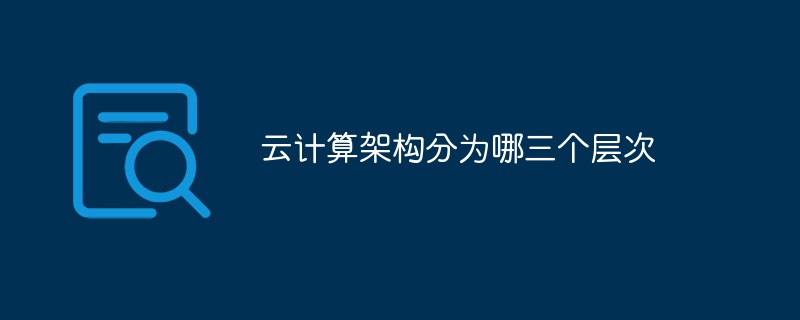
云计算架构分为基础设施层、平台层和软件服务层三个层次,云计算的目的是通过基于网络的计算方式,将共享的软件或硬件资源和信息进行组织整合,按需提供给计算机或其他系统使用。
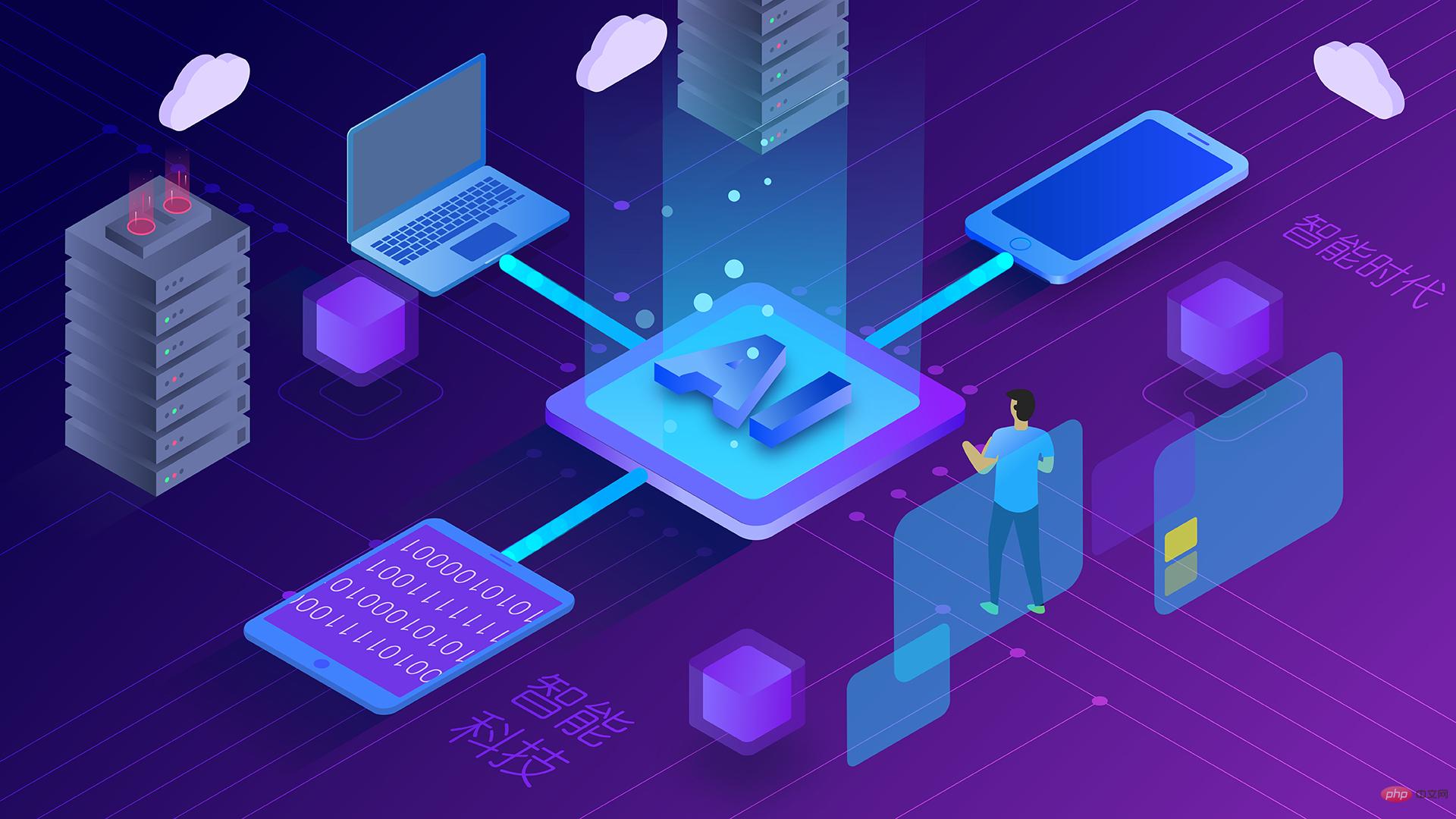
如今,边缘计算一直是热门话题。被誉为近年来最令人兴奋的技术转变,关于其变革力量的讨论很多!随着越来越强大的AI/ML算法重新定义“智能”以及更便宜、更强大的“边缘”设备的可用性,这种炒作在很大程度上是真实的。但是,如果要考虑边缘计算的历史,它会比最近的兴趣让我们相信的更早。事实上,计算和智能最初始于边缘,当时大多数应用程序几乎不存在高带宽网络连接。即使在1990年代后期,远程部署在工厂或现场的关键测量设备通常也具有处理传入传感器数据的专用计算能力。然而,这些设备中的算法在“智能”方面只是初级的
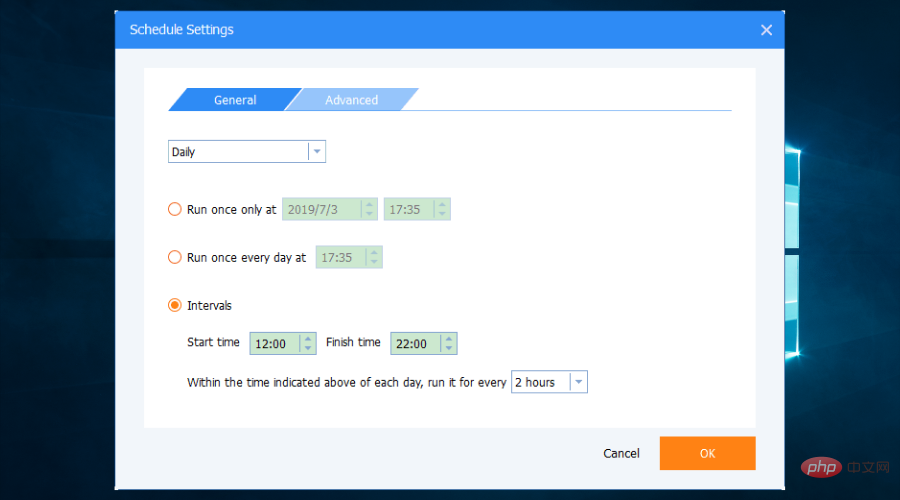
无论它多么先进,技术都可能会发生令人讨厌的转变,您可能离丢失文件只有一步之遥。例如,硬盘驱动器因崩溃而臭名昭著,而如今的勒索软件可以使计算机的内容无法访问。随着数字内容对企业以及包括视频、照片和音乐在内的个人资产变得至关重要,很明显,使用备份软件保护一切变得比以往任何时候都更加重要。为什么要使用备份软件?您需要使用备份软件的原因有很多。您的设备可能被盗,您的硬盘驱动器可能会意外崩溃,或者您可能成为恶意软件的受害者。如果您不想冒丢失所有数据的风险,请考虑使用备份软件,最好是基于云的软件,因为它们比
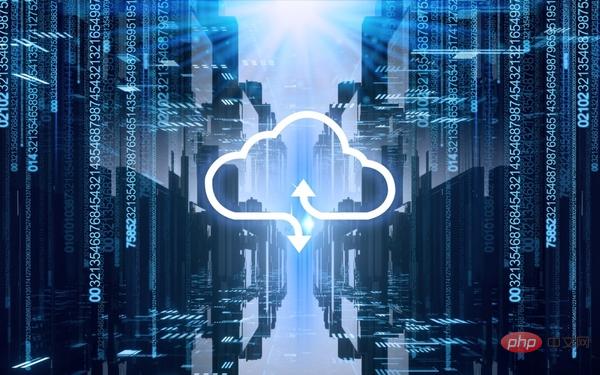
在当今的数字世界中,人工智能和云计算每天影响着许多人的工作和生活。云计算帮助企业变得更加敏捷和灵活,并提供成本效益。借助人工智能技术,有助于从数据中产生洞察力,提供卓越的客户体验。因此,协同人工智能和云计算解决方案将使企业更接近其最终客户并提高其运营效率。云计算及人工智能是什么?云计算是分布式计算的一种,指的是通过网络“云”将巨大的数据计算处理程序分解成无数个小程序,然后,通过多部服务器组成的系统进行处理和分析这些小程序得到结果并返回给用户。云计算基于按需付费的定价原则。简单来说,云计算可以定义
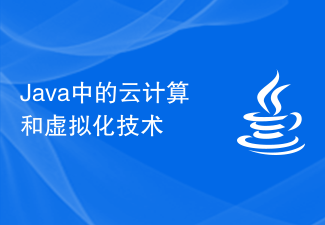
随着互联网的普及,云计算和虚拟化技术也逐渐成为了计算机领域的热门话题。其中,Java作为一种广泛应用于互联网应用开发的编程语言,也在云计算和虚拟化技术的应用过程中发挥了重要作用。本文将详细介绍Java中的云计算和虚拟化技术。一、云计算技术云计算是一种基于网络的计算方式,通过分布在不同地方的计算机和服务,为用户提供各种互联网服务。而Java由于其跨平
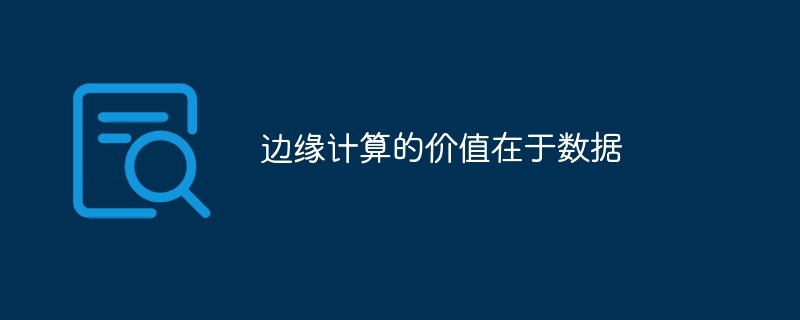
随着企业寻求可以根据不断变化的业务需求进行扩展和缩减的计算和存储资源,云的采用率急剧上升。 但即使考虑到云计算的成本和敏捷性优势,人们对另一种部署模型的兴趣也越来越高——边缘计算,即在数据源处或附近进行的计算。 它可以支持新的用例,尤其是对现代商业成功至关重要的创新人工智能和机器学习应用。在《麻省理工科技评论》(MIT Technology Review)主办的“未来计算”(Future Compute)大会上,三位工业技术专家表示,这一优势的前景在于数据。具体来说,无论是工厂、自动驾驶汽车还是


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
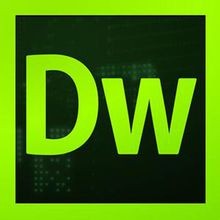
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
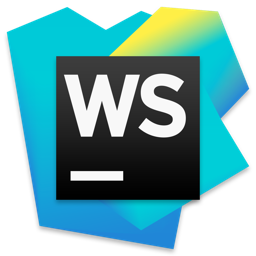
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
