Golang's practice and thinking in distributed systems
The practical application of Go language in distributed systems mainly focuses on concurrency, communication and fault tolerance. In the example of a distributed work queue, the Go language implements task communication through pipes, uses coroutines to build worker pools, and adds tasks through cron timers. Best practices include choosing the right communication mechanisms, designing resilient systems, monitoring and measuring, considering distributed transactions, and learning relevant ecosystem tools and frameworks.
Practical combat and thinking of Go language in distributed systems
Introduction
With the With the rise of distributed systems, Go language has quickly become a popular choice for building distributed systems due to its concurrency and robustness. This article will focus on the practical application of Go language in distributed systems and share some thoughts and best practices.
Core concepts of building distributed systems
- Concurrency: The Go language implements lightweight concurrency through the Goroutine mechanism, enabling We are able to easily create and manage large numbers of concurrent tasks.
- Communication: Components in a distributed system need to communicate, and the Go language provides a variety of communication mechanisms, such as pipes, RPC, and message queues.
- Fault tolerance: Distributed systems are prone to failures. The Go language provides a built-in error handling mechanism and Recover function to help us handle errors and improve the fault tolerance of the system.
Practical Case: Distributed Work Queue
To show the practical application of Go language in distributed systems, let us create a distributed work queue example.
package main import ( "context" "fmt" "log" "time" "github.com/robfig/cron" ) func main() { // 创建一个管道用于任务通信 tasks := make(chan string) // 启动工作者池(协程)处理任务 for i := 0; i < 10; i++ { go func() { for task := range tasks { // 模拟任务处理 time.Sleep(1 * time.Second) fmt.Printf("处理任务:%s\n", task) } }() } // 每分钟添加一个任务到队列 c := cron.New() c.AddFunc("@every 1m", func() { tasks <- "新任务" }) c.Start() // 等待 1 小时,然后关闭队列和工作者池 time.Sleep(1 * time.Hour) close(tasks) c.Stop() log.Println("工作队列已停止") }
Thoughts and Best Practices
- Choose the right communication mechanism: Selection is based on application requirements (performance, reliability) appropriate communication mechanism.
- Design a resilient system: Improve the fault tolerance of the system through error handling, timeout and retry mechanisms.
- Monitoring and Metrics: Use monitoring tools to track system health and identify performance bottlenecks.
- Consider distributed transactions: Use a transaction manager or distributed consensus algorithm to ensure the atomicity and consistency of distributed operations.
- Learn the ecosystem: Learn about tools and frameworks in the distributed systems space, such as Etcd, Kafka, and Kubernetes.
The above is the detailed content of Golang's practice and thinking in distributed systems. For more information, please follow other related articles on the PHP Chinese website!
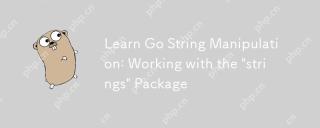
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
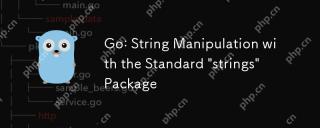
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
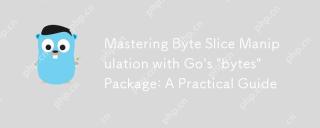
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
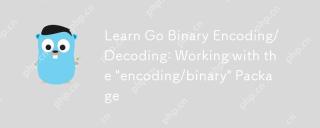
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
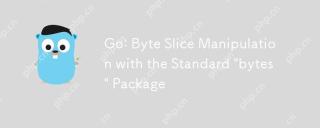
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
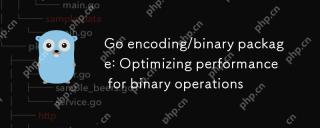
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
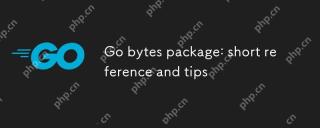
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
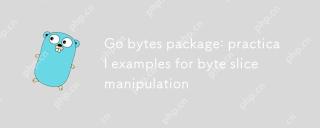
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
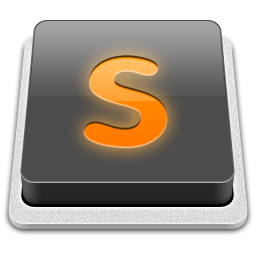
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version
