How to achieve cross-platform compatibility when using STL in C++?
To achieve cross-platform compatibility using STL in C++, follow these guidelines: Use the correct compiler options to disable or enable POSIX features depending on the target platform. Avoid relying on platform-specific features, such as file I/O or thread management. Use portability macros such as #ifdef _WIN32 to define conditional compilation. Port custom types and implementations, using platform-independent interfaces.
A practical guide to using STL in C++ for cross-platform compatibility
Introduction
The Standard Template Library (STL) is a set of C++ libraries that provides a wide range of containers, algorithms and tools. In cross-platform application development, it is crucial to ensure that STL runs consistently across different platforms. This article will guide you on how to use technology and best practices to achieve cross-platform compatibility.
1. Use the correct compiler options
Depending on the target platform, compiler options can affect the behavior of STL. For example, on Windows, you can use the /D_WIN32
option to disable POSIX functionality. On Linux and macOS, the following options are available:
/D__linux__
/D__unix__
/D__APPLE__
2. Avoid relying on platform-specific functions
STL provides many platform-independent functions and types. Avoid relying on platform-specific implementations, such as file I/O or thread management. If you need platform-specific functionality, you can use non-standard libraries or third-party libraries.
3. Use portability macros
STL provides a set of portability macros to help define conditional compilation on different platforms. For example, #ifdef _WIN32
can be used to check whether the current platform is Windows.
4. Porting custom types and implementations
If you must use custom types or implementations, use platform-independent interfaces. For example, you can use abstract base classes or interfaces to define common behavior.
Practical Case: Cross-Platform Logging
Consider a cross-platform logging application that needs to log to different targets (such as files, consoles). We can achieve cross-platform compatibility using:
Log Abstract Base Class
class ILogger { public: virtual void log(const std::string& message) = 0; virtual ~ILogger() {} };
Platform Specific Implementation
#ifdef _WIN32 class FileLogger : public ILogger { public: void log(const std::string& message) override { // Windows 文件日志记录实现 } }; #else class FileLogger : public ILogger { public: void log(const std::string& message) override { // POSIX 文件日志记录实现 } }; #endif
Application Code
auto logger = std::make_shared<FileLogger>(); logger->log("Hello, world!");
With application code, it only relies on the ILogger interface, and it can run cross-platform regardless of the underlying implementation.
The above is the detailed content of How to achieve cross-platform compatibility when using STL in C++?. For more information, please follow other related articles on the PHP Chinese website!
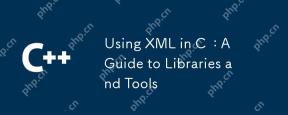
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
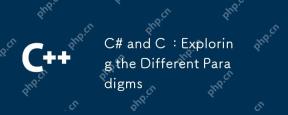
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
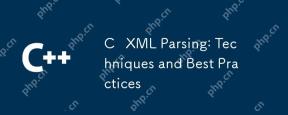
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
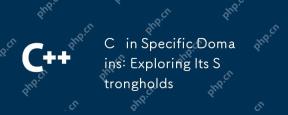
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
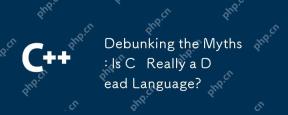
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
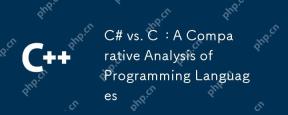
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
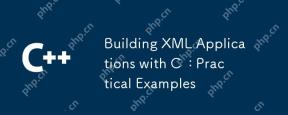
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
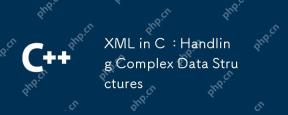
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
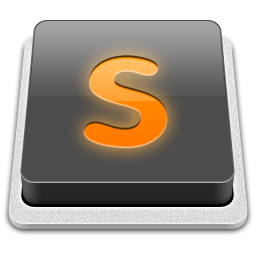
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use
